How to Find Files With a Certain Extension Only in Python
-
glob.glob
Method to Find Files With Certain Extension -
os.listdir()
Method to Find Files With Certain Extension -
pathlib.glob
Method to Find Files With Certain Extension - Find Files With a Certain Extension in the Directory and Its Subdirectories in Python
-
pathlib
Module Search Files Recursively
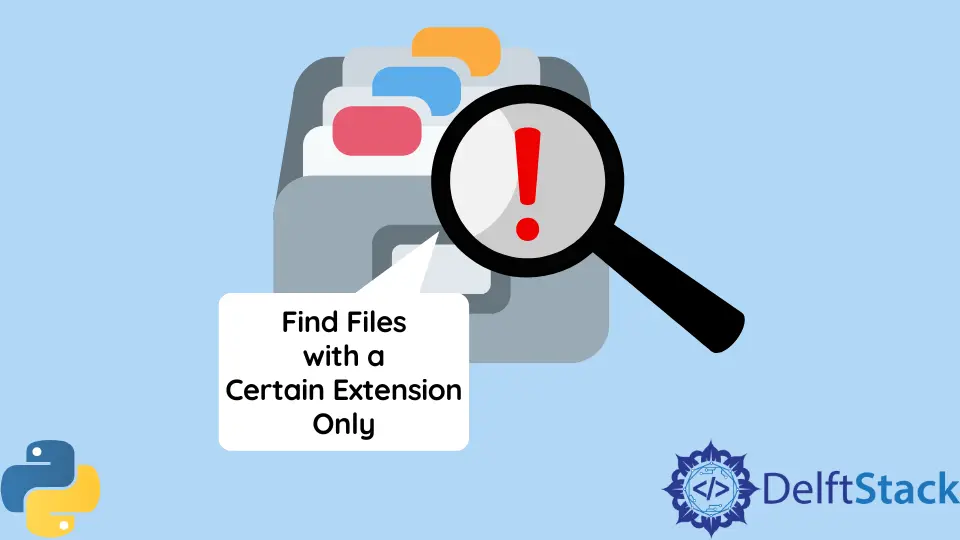
This article introduces different methods to find files with a certain extension only in Python.
glob.glob
Method to Find Files With Certain Extension
We could use glob.glob
module to find the files with a certain extension only in Python.
import glob
targetPattern = r"C:\Test\*.txt"
glob.glob(targetPattern)
The above codes demonstrate how to find the files with extension txt
in the directory C:\Test
.
os.listdir()
Method to Find Files With Certain Extension
os.listdir()
function lists all the files in the given directory, without the file path information. You could extract the files with the specific extension by using str.endswith()
function.
>>> import os
>>> fileDir = r"C:\Test"
>>> fileExt = r".txt"
>>> [_ for _ in os.listdir(fileDir) if _.endswith(fileExt)]
['test.txt', 'test1.txt']
You need to construct the full path with os.path.join()
function.
>>> import os
>>> fileDir = r"C:\Test"
>>> fileExt = r".txt"
>>> [os.path.join(fileDir, _) for _ in os.listdir(fileDir) if _.endswith(fileExt)]
['C:\\Test\\test.txt', 'C:\\Test\\test1.txt']
pathlib.glob
Method to Find Files With Certain Extension
pathlib
module is introduced in Python 3.4 which offers object-oriented filesystem paths. It provides two styles: Windows paths in Windows OS and POSIX paths in Unix-alike systems.
>>> import pathlib
>>> fileDir = r"C:\Test"
>>> fileExt = r"*.txt"
>>> list(pathlib.Path(fileDir).glob(fileExt))
[WindowsPath('C:/Test/test.txt'), WindowsPath('C:/Test/test1.txt')]
The result is represented with WindowsPath
, and you could convert the result to the string representation by adding str()
, like
>>> [str(_) for _ in pathlib.Path(fileDir).glob(fileExt)]
['C:\\Test\\test.txt', 'C:\\Test\\test.txt']
Find Files With a Certain Extension in the Directory and Its Subdirectories in Python
The pattern C:\Test\*.txt
only searches the txt
files in the directory C:\Test
, but not in its subdirectories. If you want to also get txt
files in the subdirectories, you could modify the pattern a bit.
import glob
targetPattern = r"C:\Test\**\*.txt"
glob.glob(targetPattern)
The wild cards **
between Test
and \*.txt
means it should find the txt
files both in the directory and its subdirectories.
pathlib
Module Search Files Recursively
Similar to adding **
in glob.glob
to search files recursively, you can also add **
in pathlib.Path.glob
method to find the files with a certain extension recursively.
>>> import pathlib
>>> fileDir = r"C:\Test"
>>> fileExt = r"**\*.txt"
>>> list(pathlib.Path(fileDir).glob(fileExt))
[WindowsPath('C:/Test/test.txt'), WindowsPath('C:/Test/test1.txt'), WindowsPath('C:/Test/sub/test1.txt')]
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook