PyQt5 Tutorial - Etiqueta
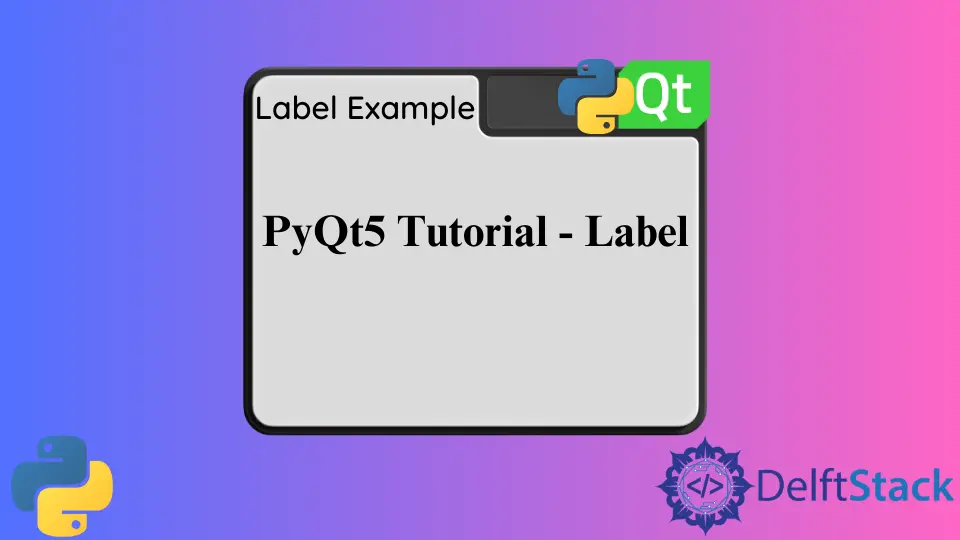
Vamos aprender a utilizar o widget de etiqueta PyQt5 QLabel
neste tutorial.
PyQt5 Label Widget
Vamos adicionar duas etiquetas à nossa janela, onde uma dessas etiquetas vai segurar algum texto e uma dessas etiquetas vai segurar uma imagem.
import sys
from PyQt5 import QtWidgets, QtGui
def basicWindow():
app = QtWidgets.QApplication(sys.argv)
windowExample = QtWidgets.QWidget()
labelA = QtWidgets.QLabel(windowExample)
labelB = QtWidgets.QLabel(windowExample)
labelA.setText("Label Example")
labelB.setPixmap(QtGui.QPixmap("python.jpg"))
windowExample.setWindowTitle("Label Example")
windowExample.setGeometry(100, 100, 300, 200)
labelA.move(100, 40)
labelB.move(120, 120)
windowExample.show()
sys.exit(app.exec_())
basicWindow()
Onde,
labelA = QtWidgets.QLabel(w)
O primeiro rótulo labelA
é um QtWidgets.QtLabel
e o QtWidgets
- w
está entre parênteses porque diz ao programa que o rótulo labelA
é adicionado à janela w
.
labelA.setText("Label Example")
O labelA.setText
define o texto no rótulo.
windowExample.setGeometry(100, 100, 300, 200)
Ele define o tamanho da janela para ser (300, 200)
e a coordenada do canto superior esquerdo para ser (100, 100)
. Você pode consultar a explicação setGeometry
em última seção.
labelA.move(100, 40)
labelB.move(120, 120)
O método move()
move a etiqueta para a direção da direita e para baixo. Como labelA.move(100, 40)
move o labelA
para a coordenada de (100, 40)
em relação ao canto superior esquerdo da janela.
labelB.setPixmap(QtGui.QPixmap("globe.png"))
Ele exibe imagens no labelB
. O QPixmap
é o módulo em QtGui
e leva uma imagem de Qt
.
PyQt5 QLabel
Set Font
No exemplo acima, usamos a fonte padrão no widget da etiqueta, e você poderia especificar a fonte preferida como tamanho, peso e família de fontes do texto da etiqueta.
import sys
from PyQt5 import QtWidgets, QtGui
def basicWindow():
app = QtWidgets.QApplication(sys.argv)
windowExample = QtWidgets.QWidget()
labelA = QtWidgets.QLabel(windowExample)
labelB = QtWidgets.QLabel(windowExample)
labelA.setText("Times Font")
labelA.setFont(QtGui.QFont("Times", 12, QtGui.QFont.Bold))
labelB.setText("Arial Font")
labelB.setFont(QtGui.QFont("Arial", 14, QtGui.QFont.Black))
windowExample.setWindowTitle("Label Example")
windowExample.setGeometry(100, 100, 300, 200)
labelA.move(100, 40)
labelB.move(100, 120)
windowExample.show()
sys.exit(app.exec_())
basicWindow()
labelA.setFont(QtGui.QFont("Times", 12, QtGui.QFont.Bold))
O método setFont()
define a fonte da etiqueta. A classe QFont
especifica uma fonte com atributos específicos.
PyQt5 Alinhamento de Etiquetas
O texto da etiqueta é por padrão alinhado à borda esquerda, e esta propriedade pode ser modificada com o método QLabel.setAlignment()
.
import sys
from PyQt5 import QtWidgets, QtGui, QtCore
def basicWindow():
app = QtWidgets.QApplication(sys.argv)
windowExample = QtWidgets.QWidget()
labelLeft = QtWidgets.QLabel(windowExample)
labelRight = QtWidgets.QLabel(windowExample)
labelCenter = QtWidgets.QLabel(windowExample)
labelLeft.setText("Left Align")
labelRight.setText("Right Align")
labelCenter.setText("Center Align")
windowExample.setWindowTitle("Label Align Example")
windowExample.setGeometry(100, 100, 300, 200)
labelLeft.setFixedWidth(160)
labelRight.setFixedWidth(160)
labelCenter.setFixedWidth(160)
labelLeft.setStyleSheet("border-radius: 25px;border: 1px solid black;")
labelRight.setStyleSheet("border-radius: 25px;border: 1px solid black;")
labelCenter.setStyleSheet("border-radius: 25px;border: 1px solid black;")
labelLeft.setAlignment(QtCore.Qt.AlignLeft)
labelRight.setAlignment(QtCore.Qt.AlignRight)
labelCenter.setAlignment(QtCore.Qt.AlignCenter)
labelLeft.move(80, 40)
labelRight.move(80, 80)
labelCenter.move(80, 120)
windowExample.show()
sys.exit(app.exec_())
basicWindow()
labelLeft.setFixedWidth(160)
labelRight.setFixedWidth(160)
labelCenter.setFixedWidth(160)
Ele define a largura fixa para as três etiquetas, caso contrário, a largura da etiqueta é automaticamente definida de acordo com o comprimento do texto da etiqueta.
labelLeft.setStyleSheet("border-radius: 25px;border: 1px solid black;")
Poderíamos utilizar a folha de estilo CSS-alike para definir os estilos dos widgets PyQt5. Aqui, a borda da etiqueta é definida para ser preta sólida com a borda de 1px, e o raio da borda é especificado como 25px.
labelLeft.setAlignment(QtCore.Qt.AlignLeft)
labelRight.setAlignment(QtCore.Qt.AlignRight)
labelCenter.setAlignment(QtCore.Qt.AlignCenter)
A propriedade de alinhamento do widget é definida pelo método setAlignment
e suas opções estão no módulo PyQt5.QtCore.Qt
, como
PyQt5.QtCore.Qt.AlignLeft
PyQt5.QtCore.Qt.AlignRight
PyQt5.QtCore.Qt.AlignCenter
Como você pode ver na imagem abaixo, as etiquetas são alinhadas de acordo com suas propriedades de alinhamento.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook