Pandas loc vs iloc
-
Selecione um valor particular em DataFrame, especificando índice e rótulo de coluna usando o método
.loc()
-
Selecione Colunas Particulares do DataFrame usando o método
.loc()
-
Filtrar linhas aplicando condição às colunas usando o método
.loc()
-
Filtrar linhas com índices usando
iloc
- Filtrar linhas e colunas específicas do DataFrame
-
Filtrar intervalo de linhas e colunas do DataFrame usando
iloc
-
Pandas
loc
vsiloc
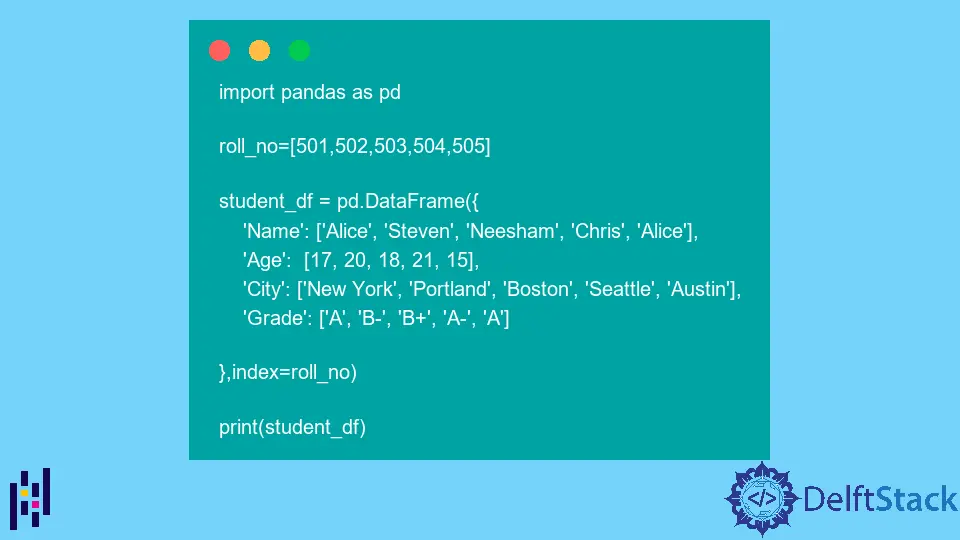
Este tutorial explica como podemos filtrar dados de um Pandas DataFrame usando loc
e iloc
em Python. Para filtrar entradas do DataFrame usando iloc
, usamos o índice inteiro para linhas e colunas, e para filtrar entradas do DataFrame usando loc
, usamos nomes de linhas e colunas.
Para demonstrar a filtragem de dados usando loc
, usaremos o DataFrame descrito no exemplo a seguir.
import pandas as pd
roll_no = [501, 502, 503, 504, 505]
student_df = pd.DataFrame(
{
"Name": ["Alice", "Steven", "Neesham", "Chris", "Alice"],
"Age": [17, 20, 18, 21, 15],
"City": ["New York", "Portland", "Boston", "Seattle", "Austin"],
"Grade": ["A", "B-", "B+", "A-", "A"],
},
index=roll_no,
)
print(student_df)
Resultado:
Name Age City Grade
501 Alice 17 New York A
502 Steven 20 Portland B-
503 Neesham 18 Boston B+
504 Chris 21 Seattle A-
505 Alice 15 Austin A
Selecione um valor particular em DataFrame, especificando índice e rótulo de coluna usando o método .loc()
Podemos passar um rótulo de índice e rótulo de coluna como um argumento para o método .loc()
para extrair o valor correspondente ao índice e rótulo de coluna fornecidos.
import pandas as pd
roll_no = [501, 502, 503, 504, 505]
student_df = pd.DataFrame(
{
"Name": ["Alice", "Steven", "Neesham", "Chris", "Alice"],
"Age": [17, 20, 18, 21, 15],
"City": ["New York", "Portland", "Boston", "Seattle", "Austin"],
"Grade": ["A", "B-", "B+", "A-", "A"],
},
index=roll_no,
)
print("The DataFrame of students with marks is:")
print(student_df)
print("")
print("The Grade of student with Roll No. 504 is:")
value = student_df.loc[504, "Grade"]
print(value)
Resultado:
The DataFrame of students with marks is:
Name Age City Grade
501 Alice 17 New York A
502 Steven 20 Portland B-
503 Neesham 18 Boston B+
504 Chris 21 Seattle A-
505 Alice 15 Austin A
The Grade of student with Roll No. 504 is:
A-
Ele seleciona o valor no DataFrame com rótulo de índice como 504
e rótulo de coluna Grade
. O primeiro argumento para o método .loc()
representa o nome do índice, enquanto o segundo argumento se refere ao nome da coluna.
Selecione Colunas Particulares do DataFrame usando o método .loc()
Também podemos filtrar as colunas necessárias do DataFrame usando o método .loc()
. Passamos a lista de nomes de coluna obrigatórios como um segundo argumento para o método .loc()
para filtrar as colunas especificadas.
import pandas as pd
roll_no = [501, 502, 503, 504, 505]
student_df = pd.DataFrame(
{
"Name": ["Alice", "Steven", "Neesham", "Chris", "Alice"],
"Age": [17, 20, 18, 21, 15],
"City": ["New York", "Portland", "Boston", "Seattle", "Austin"],
"Grade": ["A", "B-", "B+", "A-", "A"],
},
index=roll_no,
)
print("The DataFrame of students with marks is:")
print(student_df)
print("")
print("The name and age of students in the DataFrame are:")
value = student_df.loc[:, ["Name", "Age"]]
print(value)
Resultado:
The DataFrame of students with marks is:
Name Age City Grade
501 Alice 17 New York A
502 Steven 20 Portland B-
503 Neesham 18 Boston B+
504 Chris 21 Seattle A-
505 Alice 15 Austin A
The name and age of students in the DataFrame are:
Name Age
501 Alice 17
502 Steven 20
503 Neesham 18
504 Chris 21
505 Alice 15
O primeiro argumento para .loc()
é :
, que denota todas as linhas no DataFrame. Da mesma forma, passamos ["Name", "Age"]
como o segundo argumento para o método .loc()
que representa a seleção apenas das colunas Name
e Age
do DataFrame.
Filtrar linhas aplicando condição às colunas usando o método .loc()
Também podemos filtrar linhas que satisfaçam a condição especificada para valores de coluna usando o método .loc()
.
import pandas as pd
roll_no = [501, 502, 503, 504, 505]
student_df = pd.DataFrame(
{
"Name": ["Alice", "Steven", "Neesham", "Chris", "Alice"],
"Age": [17, 20, 18, 21, 15],
"City": ["New York", "Portland", "Boston", "Seattle", "Austin"],
"Grade": ["A", "B-", "B+", "A-", "A"],
},
index=roll_no,
)
print("The DataFrame of students with marks is:")
print(student_df)
print("")
print("Students with Grade A are:")
value = student_df.loc[student_df.Grade == "A"]
print(value)
Resultado:
The DataFrame of students with marks is:
Name Age City Grade
501 Alice 17 New York A
502 Steven 20 Portland B-
503 Neesham 18 Boston B+
504 Chris 21 Seattle A-
505 Alice 15 Austin A
Students with Grade A are:
Name Age City Grade
501 Alice 17 New York A
505 Alice 15 Austin A
Ele seleciona todos os alunos no DataFrame com nota A
.
Filtrar linhas com índices usando iloc
import pandas as pd
roll_no = [501, 502, 503, 504, 505]
student_df = pd.DataFrame(
{
"Name": ["Alice", "Steven", "Neesham", "Chris", "Alice"],
"Age": [17, 20, 18, 21, 15],
"City": ["New York", "Portland", "Boston", "Seattle", "Austin"],
"Grade": ["A", "B-", "B+", "A-", "A"],
},
index=roll_no,
)
print("The DataFrame of students with marks is:")
print(student_df)
print("")
print("2nd and 3rd rows in the DataFrame:")
filtered_rows = student_df.iloc[[1, 2]]
print(filtered_rows)
Resultado:
The DataFrame of students with marks is:
Name Age City Grade
501 Alice 17 New York A
502 Steven 20 Portland B-
503 Neesham 18 Boston B+
504 Chris 21 Seattle A-
505 Alice 15 Austin A
2nd and 3rd rows in the DataFrame:
Name Age City Grade
502 Steven 20 Portland B-
503 Neesham 18 Boston B+
Ele filtra a segunda e a terceira linhas do DataFrame.
Passamos o índice inteiro das linhas como um argumento para o método iloc
para filtrar as linhas do DataFrame. Aqui, o índice inteiro para a segunda e terceira linhas são 1
e 2
respectivamente, já que o índice começa em 0
.
Filtrar linhas e colunas específicas do DataFrame
import pandas as pd
roll_no = [501, 502, 503, 504, 505]
student_df = pd.DataFrame(
{
"Name": ["Alice", "Steven", "Neesham", "Chris", "Alice"],
"Age": [17, 20, 18, 21, 15],
"City": ["New York", "Portland", "Boston", "Seattle", "Austin"],
"Grade": ["A", "B-", "B+", "A-", "A"],
},
index=roll_no,
)
print("The DataFrame of students with marks is:")
print(student_df)
print("")
print("Filtered values from the DataFrame:")
filtered_values = student_df.iloc[[1, 2, 3], [0, 3]]
print(filtered_values)
Resultado:
The DataFrame of students with marks is:
Name Age City Grade
501 Alice 17 New York A
502 Steven 20 Portland B-
503 Neesham 18 Boston B+
504 Chris 21 Seattle A-
505 Alice 15 Austin A
Filtered values from the DataFrame:
Name Grade
502 Steven B-
503 Neesham B+
504 Chris A-
Ele filtra a primeira e a última coluna, ou seja, Name
e Grade
da segunda, terceira e quarta linhas do DataFrame. Passamos a lista com índices inteiros da linha como primeiro argumento e a lista com índices inteiros da coluna como segundo argumento para o método iloc
.
Filtrar intervalo de linhas e colunas do DataFrame usando iloc
Para filtrar o intervalo de linhas e colunas, podemos usar a divisão de lista e passar as fatias para cada linha e coluna como um argumento para o método iloc
.
import pandas as pd
roll_no = [501, 502, 503, 504, 505]
student_df = pd.DataFrame(
{
"Name": ["Alice", "Steven", "Neesham", "Chris", "Alice"],
"Age": [17, 20, 18, 21, 15],
"City": ["New York", "Portland", "Boston", "Seattle", "Austin"],
"Grade": ["A", "B-", "B+", "A-", "A"],
},
index=roll_no,
)
print("The DataFrame of students with marks is:")
print(student_df)
print("")
print("Filtered values from the DataFrame:")
filtered_values = student_df.iloc[1:4, 0:2]
print(filtered_values)
Resultado:
The DataFrame of students with marks is:
Name Age City Grade
501 Alice 17 New York A
502 Steven 20 Portland B-
503 Neesham 18 Boston B+
504 Chris 21 Seattle A-
505 Alice 15 Austin A
Filtered values from the DataFrame:
Name Age
502 Steven 20
503 Neesham 18
504 Chris 21
Ele seleciona a segunda, terceira e quarta linhas e a primeira e a segunda colunas do DataFrame. 1:4
representa as linhas com um índice variando de 1
a 3
e 4
é exclusivo no intervalo. Da mesma forma, 0:2
representa colunas com um índice variando de 0
a 1
.
Pandas loc
vs iloc
Para filtrar as linhas
e colunas
do DataFrame usando loc()
, precisamos passar o nome das linhas e colunas a serem filtradas. Da mesma forma, precisamos passar os índices inteiros de linhas
e colunas
a serem filtrados para filtrar os valores usando iloc()
.
import pandas as pd
roll_no = [501, 502, 503, 504, 505]
student_df = pd.DataFrame(
{
"Name": ["Alice", "Steven", "Neesham", "Chris", "Alice"],
"Age": [17, 20, 18, 21, 15],
"City": ["New York", "Portland", "Boston", "Seattle", "Austin"],
"Grade": ["A", "B-", "B+", "A-", "A"],
},
index=roll_no,
)
print("The DataFrame of students with marks is:")
print(student_df)
print("")
print("Filtered values from the DataFrame using loc:")
iloc_filtered_values = student_df.loc[[502, 503, 504], ["Name", "Age"]]
print(iloc_filtered_values)
print("")
print("Filtered values from the DataFrame using iloc:")
iloc_filtered_values = student_df.iloc[[1, 2, 3], [0, 3]]
print(iloc_filtered_values)
The DataFrame of students with marks is:
Name Age City Grade
501 Alice 17 New York A
502 Steven 20 Portland B-
503 Neesham 18 Boston B+
504 Chris 21 Seattle A-
505 Alice 15 Austin A
Filtered values from the DataFrame using loc:
Name Age
502 Steven 20
503 Neesham 18
504 Chris 21
Filtered values from the DataFrame using iloc:
Name Grade
502 Steven B-
503 Neesham B+
504 Chris A-
Ele mostra como podemos filtrar os mesmos valores do DataFrame usando loc
e iloc
.
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn