Obtenha valor de entrada em JavaScript
-
Utilize
document.getElementById(id_name)
para obter o valor de entrada em JavaScript -
Utilize
document.getElementsByClassName('class_name')
para obter o valor de entrada em JavaScript -
Utilize
document.querySelector('selector')
para obter o valor de entrada em JavaScript
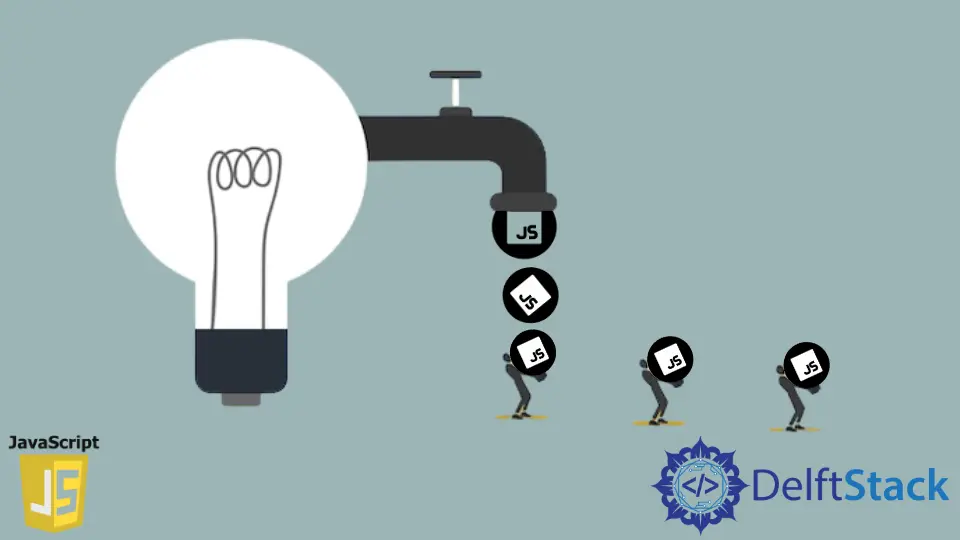
Podemos obter o valor de entrada sem o envolver dentro de um elemento do formulário em JavaScript seleccionando o elemento de entrada DOM e utilizando a propriedade value
.
O JavaScript tem diferentes métodos para seleccionar o elemento de entrada do DOM. Cada método abaixo terá um exemplo de código, que pode ser executado na sua máquina.
Utilize document.getElementById(id_name)
para obter o valor de entrada em JavaScript
Damos a propriedade id
ao nosso elemento de entrada Dom, e depois utilizamos document.getElementById(id_name)
para seleccionar o elemento de entrada DOM, pode simplesmente utilizar a propriedade value
.
Exemplo:
<!DOCTYPE html>
<html>
<body>
<h4>
Change the text of the text field
,and then click the button.
</h4>
<label for="domTextElement">Name: </label>
<input type="text" id="domTextElement" >
<button type="button" onclick="getValueInput()">
click me!!
</button>
<p id="valueInput"></p>
<script>
const getValueInput = () =>{
let inputValue = document.getElementById("domTextElement").value;
document.getElementById("valueInput").innerHTML = inputValue;
}
</script>
</body>
</html>
Utilize document.getElementsByClassName('class_name')
para obter o valor de entrada em JavaScript
Damos a propriedade de classe ao nosso elemento de entrada Dom, e depois utilizamos document.getElementsByClassName('class_name')
para seleccionar o elemento de entrada DOM, mas se tivermos diferentes elementos de entrada Dom com o mesmo nome de classe, então ele retornará um array de entradas Dom, por isso devemos especificar qual deles iremos seleccionar dando o número de índice: document.getElementsByClassName('class_name')[index_number].value
.
Exemplo:
<!DOCTYPE html>
<html>
<body>
<h4>
Change the text of the text field
,and then click the button.
</h4>
<input type="text" class="domTextElement" >
<label for="domTextElement">Name: </label>
<input type="text" class="domTextElement" >
<button type="button" onclick="getValueInput()">
click me!!
</button>
<p id="valueInput"></p>
<script>
const getValueInput = () =>{
let inputValue = document.getElementsByClassName("domTextElement")[1].value;
document.getElementById("valueInput").innerHTML = inputValue;
}
</script>
</body>
</html>
Utilize document.querySelector('selector')
para obter o valor de entrada em JavaScript
O document.querySelector('selector')
utiliza selectores CSS
o que significa, pode seleccionar elementos por id, classe, nome da etiqueta, e propriedade do nome do elemento DOM.
Exemplo:
document.querySelector('class_name')
document.querySelector('id_name')
document.querySelector('input') // tag name
document.querySelector('[name="domTextElement"]') // name property
Exemplo:
<!DOCTYPE html>
<html>
<body>
<h4>
Change the text of the text field
,and then click the button.
</h4>
<input type="text" id="domTextElement1" >
<label for="domTextElement">Name: </label>
<input type="text" class="domTextElement2" >
<button type="button" onclick="getValueInput()">
click me!!
</button>
<p id="valueInput"></p>
<script>
const getValueInput = () =>{
let inputValue1 = document.querySelector("#domTextElement1").value;
let inputValue2 = document.querySelector(".domTextElement2").value;
document.querySelector("#valueInput").innerHTML = `First input value: ${inputValue1} Second Input Value: ${inputValue2}`;
}
</script>
</body>
</html>