Use KeyListener em Java
-
Classe
KeyEvent
-
Implementando a interface
KeyListener
- Uma aplicação simples de KeyListener
- Um aplicativo de jogo simples usando KeyListener
- Resumo
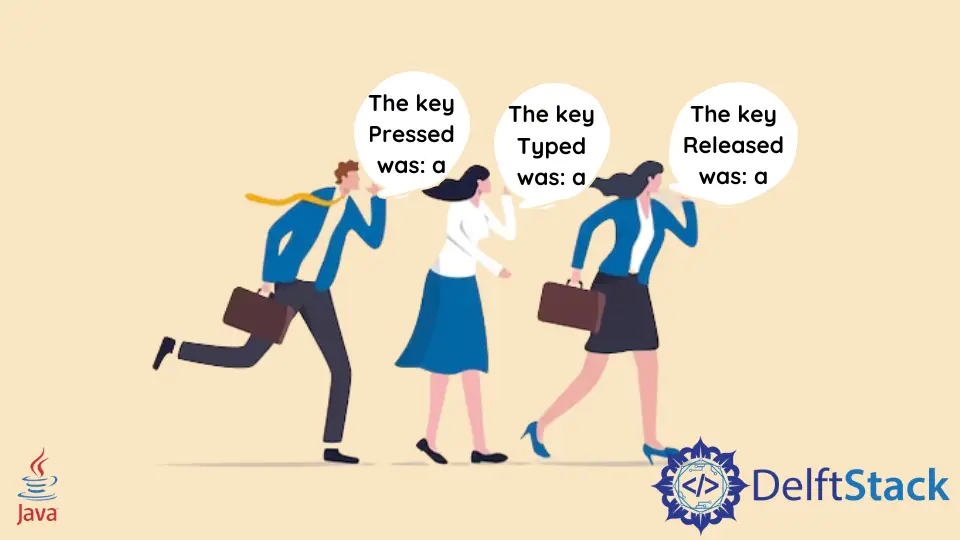
Este tutorial apresenta como usar o KeyListener em Java e lista alguns códigos de exemplo para entender o tópico.
KeyListener
é uma interface que lida com as mudanças no estado das teclas do nosso teclado. Como o nome da interface sugere, ela escuta as teclas e age de acordo.
Neste tutorial, aprenderemos como implementar essa interface e trabalhar com eventos-chave.
Classe KeyEvent
Sempre que uma tecla do teclado é pressionada, um objeto da classe KeyEvent
notifica o KeyListener
. A classe KeyEvent
possui alguns métodos usados para obter mais informações sobre o evento chave. Três dos métodos mais importantes desta classe são resumidos abaixo.
- O método
getKeyChar()
busca o caractere chave associado ao evento. Este método é muito benéfico se quisermos adicionar alguma funcionalidade a uma chave específica. Por exemplo, see
for pressionado, o aplicativo deve sair. - O método
getKeyCode()
é muito semelhante ao métodogetKeyChar()
. Ele retorna um código-chave inteiro associado à tecla pressionada. - O método
isActionKey()
pode dizer se uma tecla de ação (como Caps Lock) foi pressionada. Ele retorna um booleano verdadeiro ou falso.
Implementando a interface KeyListener
A interface KeyListener escuta os eventos principais e executa alguma ação. A declaração desta interface é mostrada abaixo.
public interface KeyListener extends EventListener
Precisamos substituir os três métodos a seguir dessa interface em nossa classe.
- O método
keyPressed(KeyEvent e)
será invocado quando uma tecla for pressionada. - O método
keyReleased(KeyEvent e)
será invocado quando a tecla for liberada. - O método
keyTyped(KeyEvent e)
será invocado quando uma chave digitar um caractere.
Também usaremos o método addKeyListener()
. Precisamos passar um objeto da classe que implementa a interface KeyListener
para este método. É uma forma de registrar o objeto para ouvir e reagir a eventos importantes.
Uma aplicação simples de KeyListener
Vamos criar um aplicativo simples que ouve eventos importantes e imprime algo no console. Vamos criar um quadro e adicionar um rótulo a ele. Nosso programa deve imprimir o caractere-chave e a ação-chave no console. Se a tecla pressionada for uma tecla de ação, o programa deve ser encerrado.
import java.awt.FlowLayout;
import java.awt.Frame;
import java.awt.Label;
import java.awt.event.KeyEvent;
import java.awt.event.KeyListener;
public class KeyListenerExample implements KeyListener {
@Override
public void keyTyped(KeyEvent e) {
System.out.println("The key Typed was: " + e.getKeyChar());
}
@Override
public void keyPressed(KeyEvent e) {
if (e.isActionKey())
System.exit(0);
System.out.println("The key Pressed was: " + e.getKeyChar());
}
@Override
public void keyReleased(KeyEvent e) {
System.out.println("The key Released was: " + e.getKeyChar());
}
public static void main(String[] args) {
// Setting the Frame and Labels
Frame f = new Frame("Demo");
f.setLayout(new FlowLayout());
f.setSize(500, 500);
Label l = new Label();
l.setText("This is a demonstration");
f.add(l);
f.setVisible(true);
// Creating and adding the key listener
KeyListenerExample k = new KeyListenerExample();
f.addKeyListener(k);
}
}
Produção:
The key Pressed was: a
The key Typed was: a
The key Released was: a
The key Pressed was: b
The key Typed was: b
The key Released was: b
Um aplicativo de jogo simples usando KeyListener
Vamos criar outro programa que terá as teclas de seta como entrada. Este programa moverá o número 0 para locais diferentes na matriz de acordo com a tecla pressionada. A saída é impressa no console.
import java.awt.FlowLayout;
import java.awt.Frame;
import java.awt.Label;
import java.awt.event.KeyEvent;
import java.awt.event.KeyListener;
import java.util.Arrays;
public class KeyListenerExample implements KeyListener {
// Matrix and x, y coordinates of 0
int[][] matrix;
int x;
int y;
KeyListenerExample() {
// Initializing the Matrix
matrix = new int[3][3];
matrix[0] = new int[] {1, 1, 1};
matrix[1] = new int[] {1, 0, 1};
matrix[2] = new int[] {1, 1, 1};
x = 1;
y = 1;
// Printing the Matrix
for (int i = 0; i < 3; i++) System.out.println(Arrays.toString(matrix[i]));
System.out.println();
}
// keyPressed() method takes care of moving the zero according to the key pressed
@Override
public void keyPressed(KeyEvent e) {
if (e.getKeyCode() == KeyEvent.VK_RIGHT) {
if (x != 2) {
x += 1;
System.out.println("Moving Right");
} else
System.out.println("Cannot Move Right");
}
if (e.getKeyCode() == KeyEvent.VK_LEFT) {
if (x != 0) {
x -= 1;
System.out.println("Moving Left");
} else
System.out.println("Cannot Move Left");
}
if (e.getKeyCode() == KeyEvent.VK_DOWN) {
if (y != 2) {
y += 1;
System.out.println("Moving Down");
} else
System.out.println("Cannot Move Down");
}
if (e.getKeyCode() == KeyEvent.VK_UP) {
if (y != 0) {
y -= 1;
System.out.println("Moving Up");
} else
System.out.println("Cannot Move Up");
}
matrix[0] = new int[] {1, 1, 1};
matrix[1] = new int[] {1, 1, 1};
matrix[2] = new int[] {1, 1, 1};
matrix[y][x] = 0;
for (int i = 0; i < 3; i++) System.out.println(Arrays.toString(matrix[i]));
System.out.println();
}
// We don't need the other two methods
@Override
public void keyReleased(KeyEvent e) {}
@Override
public void keyTyped(KeyEvent e) {}
public static void main(String[] args) {
// Setting the frame and labels
Frame f = new Frame("Demo");
f.setLayout(new FlowLayout());
f.setSize(200, 200);
Label l = new Label();
l.setText("This is a Game");
f.add(l);
f.setVisible(true);
// Creating and adding the key listener
KeyListenerExample k = new KeyListenerExample();
f.addKeyListener(k);
}
}
Produção:
[1, 1, 1]
[1, 0, 1]
[1, 1, 1]
Moving Right
[1, 1, 1]
[1, 1, 0]
[1, 1, 1]
Cannot Move Right
[1, 1, 1]
[1, 1, 0]
[1, 1, 1]
Moving Left
[1, 1, 1]
[1, 0, 1]
[1, 1, 1]
Moving Left
[1, 1, 1]
[0, 1, 1]
[1, 1, 1]
Cannot Move Left
[1, 1, 1]
[0, 1, 1]
[1, 1, 1]
Moving Up
[0, 1, 1]
[1, 1, 1]
[1, 1, 1]
Cannot Move Up
[0, 1, 1]
[1, 1, 1]
[1, 1, 1]
Moving Down
[1, 1, 1]
[0, 1, 1]
[1, 1, 1]
Moving Down
[1, 1, 1]
[1, 1, 1]
[0, 1, 1]
Cannot Move Down
[1, 1, 1]
[1, 1, 1]
[0, 1, 1]
Resumo
As classes implementam a interface KeyListener
para ouvir e reagir aos principais eventos. Neste tutorial, aprendemos alguns métodos importantes da classe KeyEvent
. Também aprendemos como implementar a interface KeyListener e como substituir os métodos keyPressed()
, keyReleased()
e keyTyped()
. Também vimos alguns exemplos que demonstram o uso dessa interface.