C# Ler e analisar um arquivo XML
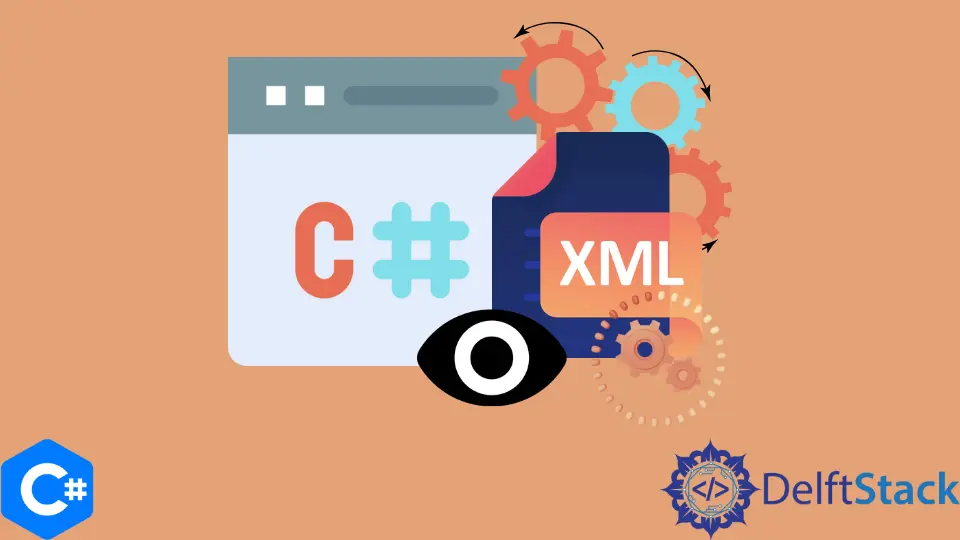
Em C#, o namespace System.Xml
é utilizado para lidar com os arquivos XML
. Tem diferentes classes e métodos para processar os arquivos XML
. Podemos ler, analisar e escrever um arquivo XML
utilizando este namespace.
Neste artigo, vamos discutir diferentes métodos que são utilizados para ler e analisar um arquivo XML
.
Programa C# para ler e analisar um arquivo XML
utilizando a classe XmlReader
A classe XmlReader
em C# fornece uma maneira eficiente de acessar os dados em XML. O método XmlReader.Read()
lê o primeiro nó do arquivo XML
e depois lê o arquivo inteiro utilizando um loop while
.
A sintaxe correta para utilizar este método é a seguinte:
XmlReader VariableName = XmlReader.Create(@"filepath");
while (reader.Read()) {
// Action to perform repeatidly
}
O arquivo XML
que lemos e analisamos em nosso programa é o seguinte. Copie e cole este código em um novo arquivo de texto e salve-o como .xml
para utilizar este arquivo para executar o programa fornecido abaixo.
<?xml version="1.0" encoding="utf-8"?>
<Students>
<Student>
</Student>
<Student>
<Name>Olivia</Name>
<Grade>A</Grade>
</Student>
<Student>
<Name>Laura</Name>
<Grade>A+</Grade>
</Student>
<Student>
<Name>Ben</Name>
<Grade>A-</Grade>
</Student>
<Student>
<Name>Henry</Name>
<Grade>B</Grade>
</Student>
<Student>
<Name>Monica</Name>
<Grade>B</Grade>
</Student>
</Students>
Exemplo de código:
using System;
using System.Xml;
namespace XMLReadAndParse {
class XMLReadandParse {
static void Main(string[] args) {
// Start with XmlReader object
// here, we try to setup Stream between the XML file nad xmlReader
using (XmlReader reader = XmlReader.Create(@"d:\Example.xml")) {
while (reader.Read()) {
if (reader.IsStartElement()) {
// return only when you have START tag
switch (reader.Name.ToString()) {
case "Name":
Console.WriteLine("The Name of the Student is " + reader.ReadString());
break;
case "Grade":
Console.WriteLine("The Grade of the Student is " + reader.ReadString());
break;
}
}
Console.WriteLine("");
}
}
Console.ReadKey();
}
}
}
Aqui criamos um objeto de XmlReader
e depois criamos um fluxo de leitura do arquivo XML
dado utilizando o método Create()
.
Então, utilizamos o método XmlReader.Read()
para ler o arquivo XML
. Este método retorna um valor bool
que indica se nosso fluxo criado contém ou não as instruções XML
.
Depois disso, verificamos se há algum elemento inicial utilizando o método XmlReader.IsStartElement()
. Como temos dois campos de elementos em nossos dados XML
, utilizamos a instrução switch
para ler os dados de ambos os campos utilizando o método ReadString()
.
Resultado:
The Name of the Student is Olivia
The Grade of the Student is A
The Name of the Student is Laura
The Grade of the Student is A +
The Name of the Student is Ben
The Grade of the Student is A -
The Name of the Student is Henry
The Grade of the Student is B
The Name of the Student is Monica
The Grade of the Student is B