Como reverter uma string em C++
- Use o String Constructor para reverter uma string
-
Utilize
std::reverse()
Algoritmo para reverter uma String -
Utilize
std::copy()
Algoritmo para inverter string
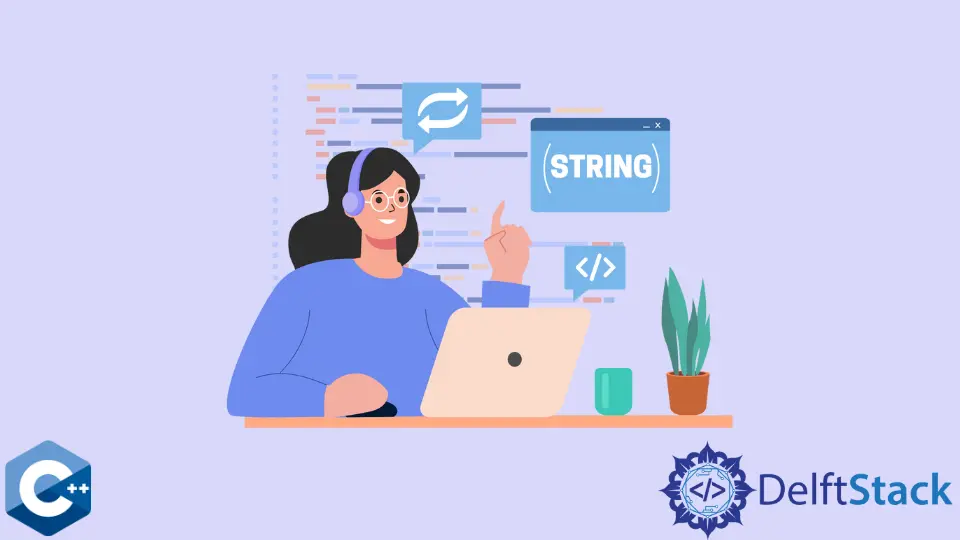
Este artigo explicará como reverter uma string em C++.
Use o String Constructor para reverter uma string
std::basic_string
tem o construtor, que pode construir um string com o conteúdo da gama. Podemos então declarar uma nova variável string e alimentar seu construtor com os iteradores invertidos da variável string original - tmp_s
. O exemplo a seguir demonstra este método e produz ambas as strings para verificação.
#include <algorithm>
#include <iostream>
#include <string>
using std::cout;
using std::endl;
using std::reverse;
using std::string;
int main() {
string tmp_s = "This string will be reversed";
cout << tmp_s << endl;
string tmp_s_reversed(tmp_s.rbegin(), tmp_s.rend());
cout << tmp_s_reversed << endl;
return EXIT_SUCCESS;
}
Resultado:
This string will be reversed
desrever eb lliw gnirts sihT
Utilize std::reverse()
Algoritmo para reverter uma String
O método std::reverse
é da <algorithm>
biblioteca STL, e inverte a ordem dos elementos da gama. O método opera sobre objetos passados como argumentos e não devolve uma nova cópia dos dados, portanto, precisamos declarar outra variável para preservar a seqüência original.
Note que a função reverse
lança a exceção std::bad_alloc
se o algoritmo não alocar memória.
#include <algorithm>
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::reverse;
using std::string;
int main() {
string tmp_s = "This string will be reversed";
cout << tmp_s << endl;
string tmp_s_reversed(tmp_s);
reverse(tmp_s_reversed.begin(), tmp_s_reversed.end());
cout << tmp_s_reversed << endl;
return EXIT_SUCCESS;
}
Utilize std::copy()
Algoritmo para inverter string
O std::copy
é outro algoritmo poderoso, que pode ser utilizado para múltiplos cenários. Esta solução inicializa uma nova variável de string e modifica seu tamanho utilizando o método redimensionar
. Em seguida, chamamos o método copy
para preencher a string declarada com os dados da string original. Observe, no entanto, que os dois primeiros parâmetros devem ser iteradores inversos da faixa de origem.
#include <algorithm>
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::reverse;
using std::string;
int main() {
string tmp_s = "This string will be reversed";
cout << tmp_s << endl;
string tmp_s_reversed;
tmp_s_reversed.resize(tmp_s.size());
copy(tmp_s.rbegin(), tmp_s.rend(), tmp_s_reversed.begin());
cout << tmp_s_reversed << endl;
return EXIT_SUCCESS;
}
Caso os dados da string invertida não precisem ser armazenados, podemos utilizar o algoritmo copy()
para emitir diretamente os dados da string em ordem inversa para o console, como mostrado na seguinte amostra de código:
#include <algorithm>
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::reverse;
using std::string;
int main() {
string tmp_s = "This string will be reversed";
cout << tmp_s << endl;
copy(tmp_s.rbegin(), tmp_s.rend(), std::ostream_iterator<char>(cout, ""));
return EXIT_SUCCESS;
}
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook