Python Tutorial - Operators
- Python Arithmetic Operators
- Python Comparison Operators
- Python Logical Operators
- Python Bitwise Operators
- Python Assignment Operators
- Python Special operators
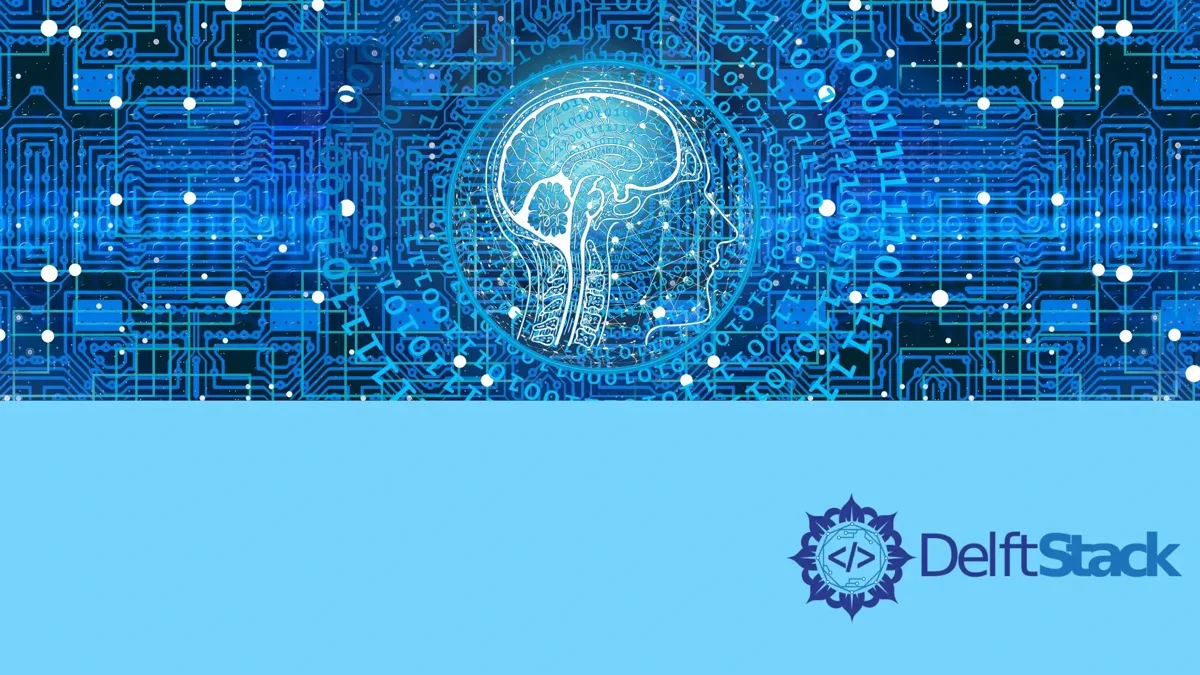
In this section, Python operators will be discussed with the help of example codes.
Operators are the special symbols to perform specific tasks on operands (values or variables) for example when you write a+b
, here +
is an operator that operates on variables a
and b
and these variables are called operands.
The following are some of the types of Python operators:
Python Arithmetic Operators
Arithmetic operators perform arithmetic (mathematical) operations on variables such as addition, subtraction, multiplication, etc.
Consider the following table in which Python arithmetic operators are described along with examples:
Operator | Description | Example |
---|---|---|
+ |
used to add to values. You can also use as unary + operator | a + b , +a |
- |
used to subtract a value from another. It can also be used as unary - operator | a - b , -a |
* |
used to multiply two numbers | a * b |
/ |
used to divide one value by another. division will always return float data type. | a / b |
% |
used to return the remainder of division on two operands. | a % b |
// |
It is called floor division which returns a whole number | a // b |
** |
It is exponent meaning left operand raise to the power of right operand | a ** b |
Python Arithmetic Operators Example
In the following code, arithmetic operators are used on two operands:
a = 24
b = 7
print("a + b =", a + b)
print("a - b =", a - b)
print("a * b =", a * b)
print("a / b =", a / b)
print("a // b =", a // b)
print("a ** b =", a ** b)
a + b = 31
a - b = 17
a * b = 168
a / b = 3.4285714285714284
a // b = 3
a ** b = 4586471424
You can see here, division returned a floating point value but floor division returned whole number.
Python Comparison Operators
Comparison operators compares two or more values. The result obtained after applying comparison operators is either True
or False
based on the condition.
The following are the comparison operators in Python programming:
Operator | Description | Example |
---|---|---|
> |
Greater than operator which returns true when value of left operand is greater than value of right operand. | a > b |
< |
Less than operator which returns true when value of left operand is less than value of right operand. | a < b |
== |
Equals to operator returns true when values of both operands are equal | a == b |
!= |
Not equal to operator returns true when values of operands are not equal | a != b |
>= |
Greater than equal to operator which returns true when value of left operand is greater than or equal to value of right operand. | a >= b |
<= |
Less than or equal to operator which returns true when value of left operand is less than or equal to value of right operand. | a <= b |
Python Comparison Operators Example
Consider the code below in which comparison operators are used:
a = 12
b = 24
print("a > b =", a > b)
print("a < b =", a < b)
print("a == b =", a == b)
print("a != b =", a != b)
print("a >= b =", a >= b)
print("a <= b =", a <= b)
a > b = False
a < b = True
a == b = False
a != b = True
a >= b = False
a <= b = True
Python Logical Operators
Logical operators in Python include and
, or
and not
.
Operator | Description | Example |
---|---|---|
and |
results in true only when both operands (or conditions) are true | a and b |
or |
results in true when any of the operands is true | a or b |
not |
takes complement of the operand (true to false, false to true) | not a |
Python Logical Operators Example
Consider the following example in which logical operators are used:
a = False
b = True
print("a and b =", a and b)
print("a or b =", a or b)
print("not b =", not b)
a and b = False
a or b = True
not b = False
Python Bitwise Operators
Bitwise operators performs bit by bit operations. The operands are considered to be binary digits on which bitwise operations are performed.
Consider an example where we have a = 4 (100 in binary) and b = 5 (101 in binary):
Operator | Description | Example |
---|---|---|
& |
Bitwise AND operator which results in 1 when bits of both operands are 1 | a &b = 4 |
| |
Bitwise OR operator which results in 1 if one of the bits of operands is 1. | a | b = 5 |
~ |
Negation, takes two’s complement. | ~a = -5 |
^ |
Bitwise XOR operator results in 1 when the bits of operands are different. | a^b= 1 |
>> |
Bitwise right shifting will shift the bits to right side by specified number of times. | a >>2= 1 |
<< |
Bitwise left shifting will shift the bits to left side by specified number of times. | a << 2 =16 |
Python Assignment Operators
Assignment operator =
assigns values to variables. Assignment operator initializes a variable for example x = 1
is an assignment statement which assigns 1 to x
.
In Python, there are compound assignment operators that are the combination of arithmetic operators and the assignment operator and sometimes a combination of bitwise and assignment operators.
For example, x += 2
is a compound assignment statement in which 2 is added to the actual value of variable x
and the result is stored in variable x
.
The following table demonstrates compound assignment statements:
Operator | Example | Equivalent to |
---|---|---|
= |
a = 8 |
a = 8 |
+= |
a += 8 |
a = a + 8 |
-= |
a -= 8 |
a = a - 8 |
*= |
a *= 8 |
a = a * 8 |
/= |
a /= 8 |
a = a / 8 |
%= |
a %= 8 |
a = a % 8 |
/= |
a /= 8 |
a = a / 8 |
*= |
a *= 8 |
a = a * 8 |
&= |
a &= 8 |
a = a & 8 |
` | =` | `a |
^= |
a ^= 8 |
a = a ^ 8 |
>>= |
a >>= 8 |
a = a >> 8 |
<<= |
a <<= 8 |
a = a << 8 |
Python Special operators
In Python programming, there are some operators that have a special meaning and are called special operators for example membership operator, identity operators, etc.
Python Identity Operators
In Python the operators is
and is not
are called identity operators. The identity operators are used to determine if two variables lie in the same part of memory. So two variables to be equal and two variables to be identical are two different statements.
Operator | Description |
---|---|
is |
returns true when variables are identical (variables referring to same objects) |
is not |
returns true when variables are not identical (variables not referring to same objects) |
Python Identity Operators Example
Consider the code below:
a = 3
b = 3
print(a is b)
print(a is not b)
True
False
In this example, variables a
and b
have same values and you can see that they are identical as well.
Python Membership Operators
In Python the operators in
and not in
are called membership operators. The membership operators are used to determine if variables lie in a sequence (list, tuple, string and dictionary) or not.
You can do dictionary membership operation for the key
in dictionary but not for the value
.
Operator | Description |
---|---|
in |
return true when value is present in the sequence |
not in |
return true when value is not present in the sequence |
Python Membership Operators Example
Consider the code below:
a = "Python Programming"
print("y" in a)
print("b" not in a)
print("b" in a)
True
True
False
In this code, y
is present in the sequence (string) so True
is returned. Similarly, b
is not present in a
so again True
is returned as not in
is used. The False
is returned as b
is not in a
and in
operator is used.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook