TypeScript에서 객체 배열 반복
- TypeScript의 배열
-
TypeScript에서
forEach
메서드를 사용하여 객체 배열을 반복합니다. -
TypeScript에서
for...of
문을 사용하여 객체 배열을 반복합니다. -
TypeScript에서
for...in
문을 사용하여 객체 배열을 반복합니다. -
TypeScript에서
for
루프를 사용하여 객체 배열을 반복합니다.
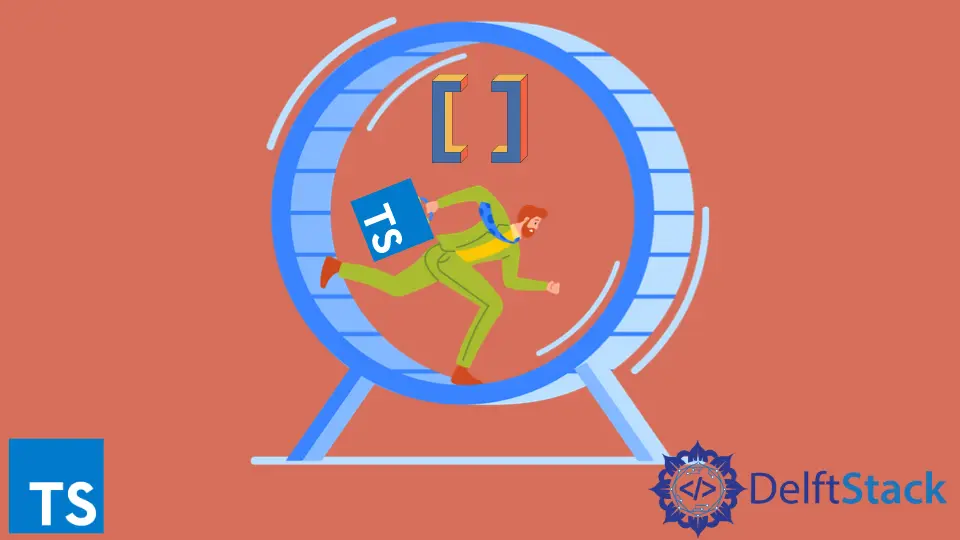
이 기사에서는 TypeScript에서 객체 배열을 반복하는 방법을 설명합니다.
TypeScript의 배열
TypeScript 배열은 사용자 정의입니다. 문자열, 숫자 등과 같은 다양한 데이터 유형의 여러 값을 저장할 수 있는 특수 데이터 유형입니다.
배열은 고정 크기 데이터 구조입니다. 요소 배열은 인덱스 기반 저장소에 저장됩니다.
첫 번째 요소는 인덱스 0에 저장되고 추가된 각 요소에 대해 1씩 증가합니다.
색인 | 값/요소 |
---|---|
0 | 12 |
1 | 25 |
2 | 100 |
삼 | 45 |
TypeScript는 다음 구문을 사용하여 배열을 선언하고 초기화합니다.
let carBrands: string[] = ["Audi", "BMW", "Toyota", "Mercedes"];
배열 요소에 액세스
요소의 배열은 요소의 인덱스를 사용하여 아래와 같이 액세스할 수 있습니다.
예제 코드:
console.log(carBrands[0]);
console.log(carBrands[1]);
console.log(carBrands[2]);
console.log(carBrands[3]);
출력:
Audi
BMW
Toyota
Mercedes
TypeScript에서 요소 배열을 반복하는 방법에는 여러 가지가 있습니다.
forEach
방법for
루프for...of
문for...in
문
TypeScript에서 forEach
메서드를 사용하여 객체 배열을 반복합니다.
이 메소드는 TypeScript 배열의 각 요소 또는 객체에 대해 함수를 실행합니다. forEach
메서드는 다음과 같이 선언할 수 있습니다.
통사론:
your_array.forEach(callback function);
문자열 배열을 선언해 봅시다.
let carBrands: string[] = ["Audi", "BMW", "Toyota", "Mercedes"];
forEach
메소드를 사용하여 carBrands
의 모든 배열 요소를 다른 배열로 복사합니다. copyOfCarBrands
라는 빈 배열을 선언해 보겠습니다.
let copyOfCarBrands: string[] = [];
forEach
메서드를 사용하여 carBrands
배열의 각 요소를 반복하고 새 copyOfCarBrands
배열로 푸시합니다.
carBrands.forEach(function(brand){
copyOfCarBrands.push(brand);
});
마지막으로 copyOfCarBrands
배열을 인쇄해 보겠습니다.
console.log(copyOfCarBrands);
출력:
[ 'Audi', 'BMW', 'Toyota', 'Mercedes' ]
다중 유형 배열에도 forEach
메서드를 사용할 수 있습니다. multiValues
라는 다중 유형 배열을 정의하고 각 항목을 콘솔에 기록해 보겠습니다.
let multiValues: (string | number)[] = ['Asia', 1000, 'Europe', 340, 20];
multiValues.forEach(function(value){
console.log(value);
});
출력:
Asia
1000
Europe
340
20
forEach
방법에는 몇 가지 단점이 있습니다.
- 이 방법은 배열에만 사용할 수 있습니다.
- 루프를 끊을 방법이 없습니다.
TypeScript에서 for...of
문을 사용하여 객체 배열을 반복합니다.
for...of
루프 문은 배열의 요소에 액세스하여 반환할 수 있습니다. 아래와 같이 사용할 수 있습니다.
통사론:
for (let variable of givenArray ) {
statement;
}
각 반복에서 variable
은 givenArray
의 배열 요소에 할당됩니다.
배열을 선언해 봅시다.
let fruits: string[] = ["Apple", "Grapes", "Mangoe", "Banana"];
아래에서 for...of
루프를 사용하여 fruits
배열의 각 요소에 액세스할 수 있습니다.
for (let fruitElement of fruits) {
console.log(fruitElement);
}
출력:
Apple
Grapes
Mangoe
Banana
TypeScript에서 for...in
문을 사용하여 객체 배열을 반복합니다.
for...in
루프 구문은 for...of
와 거의 유사합니다. 그러나 각 반복에서 배열 요소의 인덱스를 반환합니다.
이 루프는 다음과 같이 사용할 수 있습니다.
통사론:
for (let variable in givenArray ) {
statement;
}
변수
는 각 반복에서 각 요소의 인덱스에 할당됩니다. givenArray
는 반복 가능한 배열입니다.
숫자 배열을 정의해 봅시다.
let numberArr: number[] = [100,560,300,20];
반환된 인덱스 값을 사용하여 numberArr
의 각 요소에 액세스할 수 있습니다.
for (let variable in numberArr ) {
console.log(numberArr[variable]);
}
각 반복에서 변수
는 numberArr
의 각 요소 인덱스에 할당됩니다. 따라서 numberArr[index]
로 요소 값에 액세스할 수 있습니다.
이 경우 인덱스
값은 각 반복에서 변수
가 됩니다.
출력:
100
560
300
20
TypeScript에서 for
루프를 사용하여 객체 배열을 반복합니다.
for
루프는 배열 요소를 반복하는 가장 일반적인 방법입니다. 아래와 같이 사용할 수 있습니다.
통사론:
for (let index; index<arraySize; index++) {
statement;
}
index
값은 반복할 때마다 증가하고 index < arraySize
조건이 충족될 때까지 루프가 계속됩니다.
문자열 배열을 만들어 봅시다.
let oceanArr: string[] = ['Atlantic', 'Indian', 'Pacific'];
oceanArr
의 각 요소를 반복하고 아래와 같이 인쇄할 수 있습니다.
for (let index=0; index<oceanArr.length; index++) {
console.log(oceanArr[index]);
}
출력:
Atlantic
Indian
Pacific
Nimesha is a Full-stack Software Engineer for more than five years, he loves technology, as technology has the power to solve our many problems within just a minute. He have been contributing to various projects over the last 5+ years and working with almost all the so-called 03 tiers(DB, M-Tier, and Client). Recently, he has started working with DevOps technologies such as Azure administration, Kubernetes, Terraform automation, and Bash scripting as well.
관련 문장 - TypeScript Array
- TypeScript 인터페이스에서 배열 정의
- TypeScript를 사용하여 개체를 배열로 푸시
- TypeScript에서 배열 초기화
- TypeScript에서 빈 배열 만들기
- TypeScript에서 객체 배열 정렬