TypeScript에서 배열 초기화
Shuvayan Ghosh Dastidar
2023년6월21일
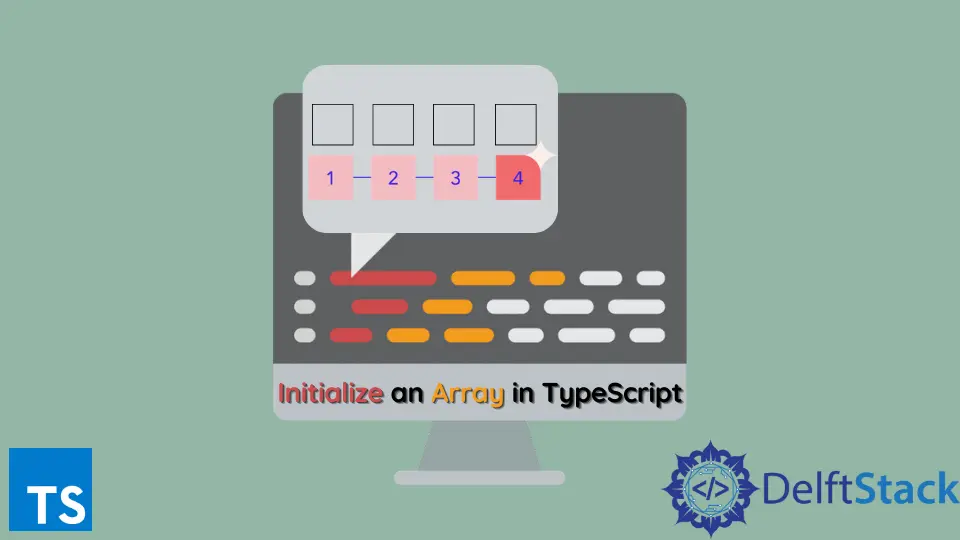
TypeScript 또는 JavaScript의 배열은 연속 메모리 세그먼트를 저장하기 위한 데이터 구조입니다. 배열은 매우 유용하며 거의 모든 응용 프로그램에서 자주 사용됩니다.
이 튜토리얼은 TypeScript에서 배열을 초기화하는 방법을 보여줍니다.
TypeScript에서 특정 유형의 배열 초기화
TypeScript의 배열은 빈 배열 값과 사용자 정의 유형에 할당된 Array
유형을 사용하여 초기화할 수 있습니다.
class Product {
name : string;
quantity : number;
constructor(opt : { name? : string, quantity? : number}){
this.name = opt.name ?? 'default product';
this.quantity = opt.quantity ?? 0;
}
}
var products : Array<Product> = [];
products.push( new Product({name : 'pencil', quantity : 10}) )
products.push( new Product({name : 'pen', quantity : 4}) )
console.log(products);
출력:
[Product: {
"name": "pencil",
"quantity": 10
}, Product: {
"name": "pen",
"quantity": 4
}]
var products : Array<Product> = [];
대신 var products : Product[] = [];
를 사용할 수도 있으며 TypeScript에서 동일한 기능과 타이핑 지원을 얻을 수 있습니다.
배열은 인터페이스
유형일 수도 있습니다.
interface Product {
name: string;
quantity : number;
}
var products : Array<Product> = [];
var prod : Product[] = []
products.push({name : 'pencil', quantity : 10});
products.push({name : 'pen', quantity : 4});
console.log(products);
출력:
[{
"name": "pencil",
"quantity": 10
}, {
"name": "pen",
"quantity": 4
}]
명명된 매개변수를 사용하여 TypeScript에서 배열 초기화
Array()
클래스를 사용하여 클래스 내에서 배열을 초기화할 수도 있습니다. 그런 다음 클래스 변수를 직접 사용하여 배열의 요소를 설정하거나 요소를 푸시할 수 있습니다.
class Car {
model : string;
buildYear : number;
constructor( opt : { model? : string, buildYear? : number }){
this.model = opt.model ?? 'default';
this.buildYear = opt.buildYear ?? 0;
}
}
class Factory {
cars : Car[] = new Array();
}
var factory = new Factory();
factory.cars = [ new Car({model : 'mercedes', buildYear : 2004}), new Car( { model : 'BMW', buildYear : 2006}) ];
console.log(factory.cars);
출력:
[Car: {
"model": "mercedes",
"buildYear": 2004
}, Car: {
"model": "BMW",
"buildYear": 2006
}]