TypeScript의 implements 키워드
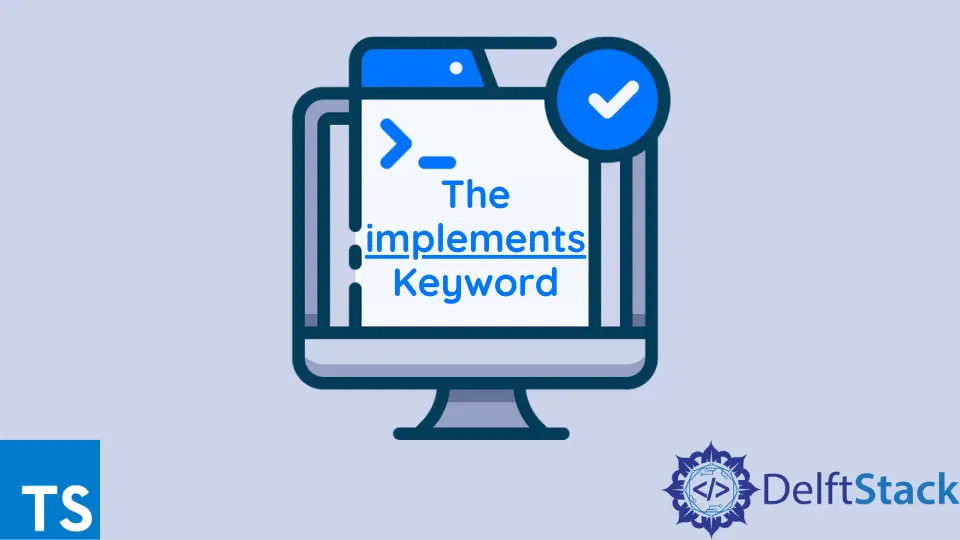
이 문서에서는 TypeScript implements
키워드에 대해 설명합니다.
TypeScript 유형 검사
TypeScript는 컴파일 시간에 유형 검사를 포함하는 JavaScript의 상위 집합입니다. 컴파일 타임 유형 검사는 주로 변수 또는 인수 값이 원하는 모양을 가지고 있는지 여부에 중점을 둡니다.
이 기술을 TypeScript에서 덕 타이핑이라고 합니다. 덕 타이핑의 결과 TypeScript 컴파일러는 두 개체가 동일한지 확인합니다.
TypeScript 프로그램에서 형식 안전성을 보장합니다.
유형 검사는 지정된 두 객체에 동일한 속성과 메서드가 포함되어 있는지 확인합니다. 그렇지 않으면 TypeScript 컴파일러에서 오류가 발생합니다.
다음과 같이 TypeScript 클래스가 세 개 있다고 가정해 보겠습니다.
class Person {
gender: string;
}
class Employee {
gender: string;
empId: string;
}
class Manager {
gender: string;
empId: string;
}
새로운 Person
및 Employee
개체를 생성해 보겠습니다.
let person1: Person = new Person();
let employee1: Employee = new Employee();
이 코드는 유형 검사 규칙을 위반하지 않기 때문에 성공적으로 컴파일됩니다.
employee1
개체를 Person
유형 변수에 할당해 보겠습니다.
let person2: Person = employee1;
employee1
개체가 Person
개체 모양을 가지므로 성공적으로 컴파일되었습니다. Person
개체에는 gender
라는 속성이 하나만 있어야 하므로 employee1
개체를 따릅니다.
추가 empId
속성은 전혀 문제가 없습니다.
다음과 같이 Employee
유형 변수에 person1
객체를 할당해 보겠습니다.
let employee2: Employee = person1;
TypeScript 오리 타이핑 규칙은 이것을 허용하지 않습니다. 따라서 TypeScript 컴파일러에서 오류가 발생합니다.
일반적으로 TypeScript 인터페이스는 TypeScript 코드에서 유형과 계약을 정의하는 역할을 합니다.
TypeScript 인터페이스
TypeScript 인터페이스는 클래스의 모양을 제공합니다. TypeScript 클래스가 인터페이스에서 파생될 때마다 인터페이스에서 정의한 구조를 구현해야 합니다.
실제 계약과 같습니다. 클래스가 인터페이스를 받아들이는 한 인터페이스 구조를 따라야 합니다. 인터페이스는 비즈니스 로직을 구현하지 않습니다.
다음과 같이 속성과 메서드만 선언합니다.
interface IVehicle {
vehicleNumber: string;
vehicleBrand: string;
vehicleCapacity: number;
getTheCurrentGear: () => number;
getFuelLevel(): string;
}
TypeScript 인터페이스는 유형, 함수 유형, 배열 유형 등으로 사용할 수 있습니다. 다음 섹션에서 설명하는 것처럼 클래스에 대한 계약으로 사용할 수 있습니다.
TypeScript의 implements
절
TypeScript implements
키워드는 클래스 내에서 인터페이스를 구현하는 데 사용됩니다. IVehicle
인터페이스에는 구현 수준 세부 정보가 포함되어 있지 않습니다.
따라서 IVehicle
인터페이스는 새로운 VipVehicle
클래스로 구현할 수 있습니다.
class VipVehicle implements IVehicle {
}
VipVehicle
클래스는 위의 예와 같이 IVehicle
인터페이스와 계약을 맺습니다. 이 TypeScript 코드를 컴파일하면 다음과 같이 오류가 발생합니다.
TypeScript 컴파일러는 VipVehicle
클래스 내에서 세 가지 속성과 두 가지 메서드가 구현되지 않았다고 불평합니다. 따라서 IVehicle
인터페이스의 구조를 준수하는 것이 필수입니다.
세 가지 속성을 추가하고 그에 따라 두 가지 메서드를 구현해야 합니다.
class VipVehicle implements IVehicle {
vehicleNumber: string;
vehicleBrand: string;
vehicleCapacity: number;
getTheCurrentGear():number {
return 3; // dummy numebr returning here for demo purposes
}
getFuelLevel():string {
return 'full'; // dummy string returning here for demo purposes
}
}
코드를 다시 컴파일하면 성공적으로 컴파일됩니다.
Nimesha is a Full-stack Software Engineer for more than five years, he loves technology, as technology has the power to solve our many problems within just a minute. He have been contributing to various projects over the last 5+ years and working with almost all the so-called 03 tiers(DB, M-Tier, and Client). Recently, he has started working with DevOps technologies such as Azure administration, Kubernetes, Terraform automation, and Bash scripting as well.