TypeScript에서 클래스 상수 구현
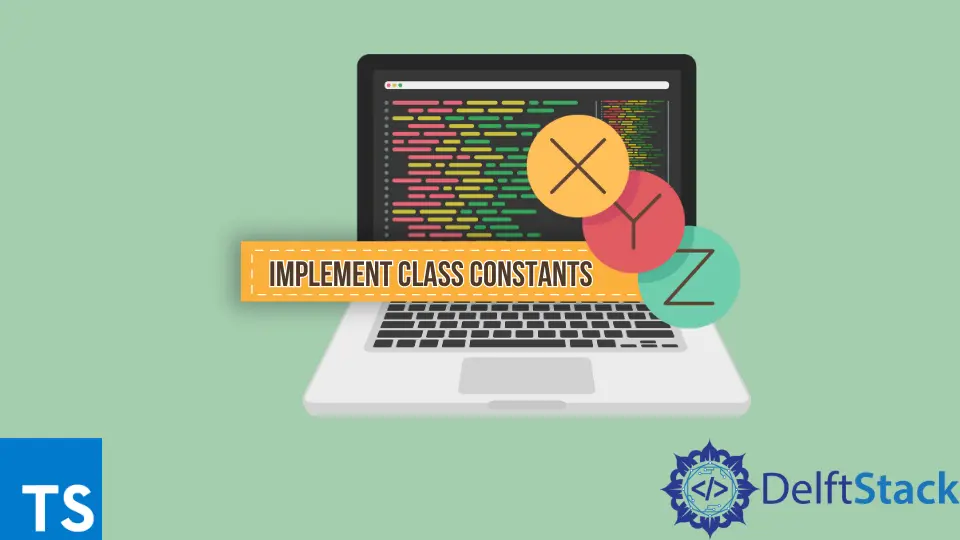
이 문서에서는 readonly
키워드 및 readonly
키워드와 함께 static
키워드를 사용하여 클래스 상수를 구현하는 방법을 설명합니다.
TypeScript의 상수
프로그래밍 언어의 상수는 한 번 할당되면 값을 변경할 수 없는 고정 값입니다. 클래스 상수는 일단 할당되면 변경할 수 없는 클래스 내부의 상수 필드입니다.
TypeScript에서 클래스를 만드는 것은 간단한 작업입니다. 다음은 TypeScript에서 클래스를 만드는 방법에 대한 구문입니다.
class className {
// Block of code
}
위는 TypeScript에서 클래스를 만드는 간단한 방법입니다. 변경할 수 없거나 변경할 수 없는 값을 가진 상수 필드 또는 속성이 있는 클래스를 만드는 몇 가지 방법을 따를 수 있습니다.
TypeScript에서 클래스 멤버는 const
키워드를 가질 수 없다는 점을 기억하는 것이 중요합니다. 클래스 멤버 상수의 인라인 선언은 TypeScript에서 불가능합니다.
그렇게 하려고 하면 컴파일러에서 컴파일 오류가 발생합니다. 클래스를 상수로 만들기 위해 다음 섹션에서 설명하는 TypeScript에서 사용할 수 있는 몇 가지 옵션을 구현할 수 있습니다.
TypeScript에서 readonly
를 사용하여 클래스 상수 구현
클래스 속성 또는 필드를 변경할 수 없도록 만든 클래스를 만든 후 TypeScript 2.0에 도입된 readonly
키워드를 사용하여 클래스 필드 또는 속성을 변경할 수 없도록 정의할 수 있습니다. 속성을 변경하려고 하면 오류 메시지가 나타납니다.
예:
class DisplayOutput{
readonly name : string = "Only using readonly";
displayOutput() : void{
console.log(this.name);
}
}
let instance = new DisplayOutput();
instance.displayOutput();
출력:
Only using readonly
readonly
만 사용하면 완전히 상수로 작동하지 않습니다. 이는 생성자에서 할당이 허용되기 때문입니다.
TypeScript에서 정적
과 함께 readonly
를 사용하여 클래스 상수 구현
클래스 필드나 속성을 변경할 수 없도록 만드는 데 사용할 수 있는 또 다른 방법은 정적
키워드와 함께 readonly
키워드를 사용하는 것입니다. readonly
를 사용하는 것과 비교할 때 이것의 장점은 할당이 생성자에서 허용되지 않으므로 한 곳에만 할당된다는 것입니다.
예:
class DisplayOutput{
static readonly username : string = "Using both static and readonly keywords";
static displayOutput() : void {
console.log(DisplayOutput.username);
}
}
DisplayOutput.displayOutput();
출력:
Using both static and readonly keywords
Nimesha is a Full-stack Software Engineer for more than five years, he loves technology, as technology has the power to solve our many problems within just a minute. He have been contributing to various projects over the last 5+ years and working with almost all the so-called 03 tiers(DB, M-Tier, and Client). Recently, he has started working with DevOps technologies such as Azure administration, Kubernetes, Terraform automation, and Bash scripting as well.