TypeScript의 Axios 응답
- TypeScript에서 Axios 사용
- Axios 라이브러리의 유형
- Axios를 사용하여 TypeScript에서 REST API 호출 만들기
-
TypeScript에서 Axios
구성
파일 생성
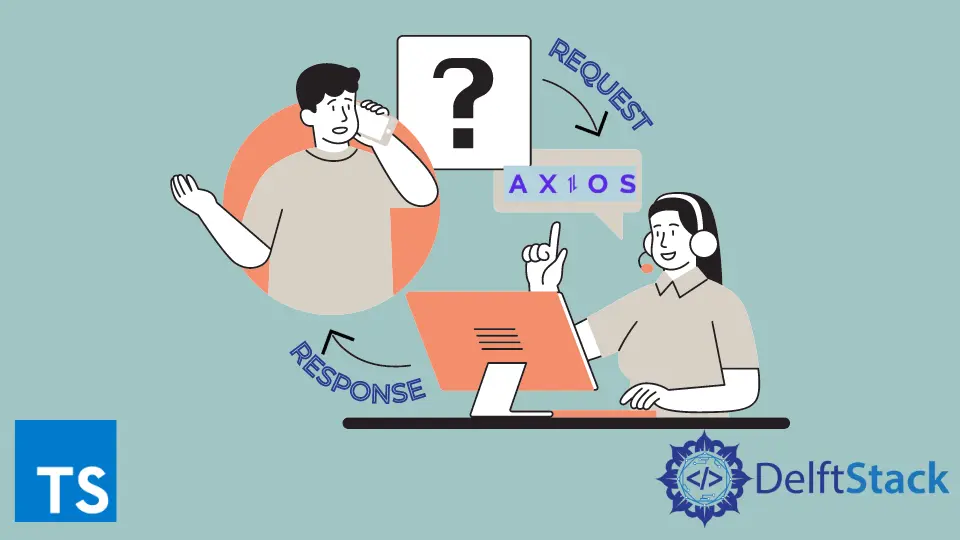
Axios는 백엔드 리소스에 대한 요청을 관리하기 위한 널리 사용되는 JavaScript 라이브러리입니다. TypeScript에 대한 수요가 증가함에 따라 Axios 라이브러리에 유형이 추가되었습니다.
이 튜토리얼에서는 Axios를 사용하여 TypeScript에서 REST API를 호출합니다.
TypeScript에서 Axios 사용
첫 번째 단계는 프로젝트에 Axios를 설치하는 것입니다. Axios는 NodeJs 또는 React 프로젝트에 설치할 수 있습니다.
npm install axios
// or
yarn install axios
이제 Axios를 다른 패키지와 함께 프로젝트에서 사용할 수 있습니다.
Axios 라이브러리의 유형
Axios Github 리포지토리에서 미리 빌드된 여러 유형을 사용할 수 있습니다. 이 튜토리얼은 Axios의 몇 가지 중요한 유형에 초점을 맞출 것입니다.
export interface AxiosResponse<T = never> {
data: T;
status: number;
statusText: string;
headers: Record<string, string>;
config: AxiosRequestConfig<T>;
request?: any;
}
AxiosResponse
는 GET
또는 POST
와 같은 REST API 호출로 인해 Promise로 반환되는 응답 객체입니다.
get<T = never, R = AxiosResponse<T>>(url: string, config?: AxiosRequestConfig<T>): Promise<R>;
post<T = never, R = AxiosResponse<T>>(url: string, data?: T, config?: AxiosRequestConfig<T>): Promise<R>;
위의 코드는 Axios의 두 가지 REST API 메소드와 그 유형을 보여줍니다. AxiosRequestConfig
는 여기에 정의된 상당히 큰 인터페이스입니다.
인터페이스의 중요한 필드 중 일부는 다음과 같습니다.
export interface AxiosRequestConfig<T = any> {
url?: string;
method?: Method;
baseURL?: string;
data?: T;
headers?: Record<string, string>;
params?: any;
...
}
따라서 baseURL 또는 전체 URL을 요청의 일부로 넣을 수 있습니다. 여기서 주목해야 할 중요한 사항 중 하나는 AxiosRequestConfig
및 AxiosResponse
의 data
필드로, 일반 유형 T
이며 모든 유형을 허용할 수 있습니다.
Axios를 사용하여 TypeScript에서 REST API 호출 만들기
위 유형은 TypeScript에서 유형이 지정된 REST API 호출을 만들 수 있습니다. 전자 상거래 웹사이트가 백엔드에 대한 REST API 호출을 만들어 프런트엔드에서 사용할 수 있는 모든 책을 표시한다고 가정합니다.
interface Book {
isbn : string;
name : string;
price : number;
author : string;
}
axios.get<Book[]>('/books', {
baseURL : 'https://ecom-backend-example/api/v1',
}).then( response => {
console.log(response.data);
console.log(response.status);
})
React를 사용하면 반환된 데이터를 AxiosResponse
의 일부로 다음과 같은 상태로 저장할 수도 있습니다.
const [books, setBooks] = React.useState<Book[]>([]);
axios.get<Book[]>('/books', {
baseURL : 'https://ecom-backend-example/api/v1',
}).then( response => {
setBooks(response.data);
})
TypeScript에서 Axios 구성
파일 생성
Axios는 많은 유용한 유형을 제공하며 애플리케이션 전체에서 REST API 호출을 만드는 데 추가로 사용할 수 있는 config
파일을 만드는 데 사용할 수 있습니다.
interface Book {
isbn : string;
name : string;
price : number;
author : string;
}
const instance = axios.create({
baseURL: 'https://ecom-backend-example/api/v1',
timeout: 15000,
});
const responseBody = (response: AxiosResponse) => response.data;
const bookRequests = {
get: (url: string) => instance.get<Book>(url).then(responseBody),
post: (url: string, body: Book) => instance.post<Book>(url, body).then(responseBody),
delete: (url: string) => instance.delete<Book>(url).then(responseBody),
};
export const Books {
getBooks : () : Promise<Book[]> => bookRequests.get('/books'),
getSingleBook : (isbn : string) : Promise<Book> => bookRequests.get(`/books/${isbn}`),
addBook : (book : Book) : Promise<Book> => bookRequests.post(`/books`, book),
deleteBook : (isbn : string) : Promise<Book> => bookRequests.delete(`/books/${isbn}`)
}
따라서 애플리케이션 전체에서 config
를 다음과 같이 사용할 수 있습니다.
import Books from './api' // config added in api.ts file
const [books, setBooks] = React.useState<Book[]>([]);
Books.getPosts()
.then((data) => {
setBooks(data);
})
.catch((err) => {
console.log(err);
});
따라서 Axios를 사용하면 REST API 호출을 명확하고 강력한 형식으로 구현할 수 있습니다.