Scala의 with 키워드
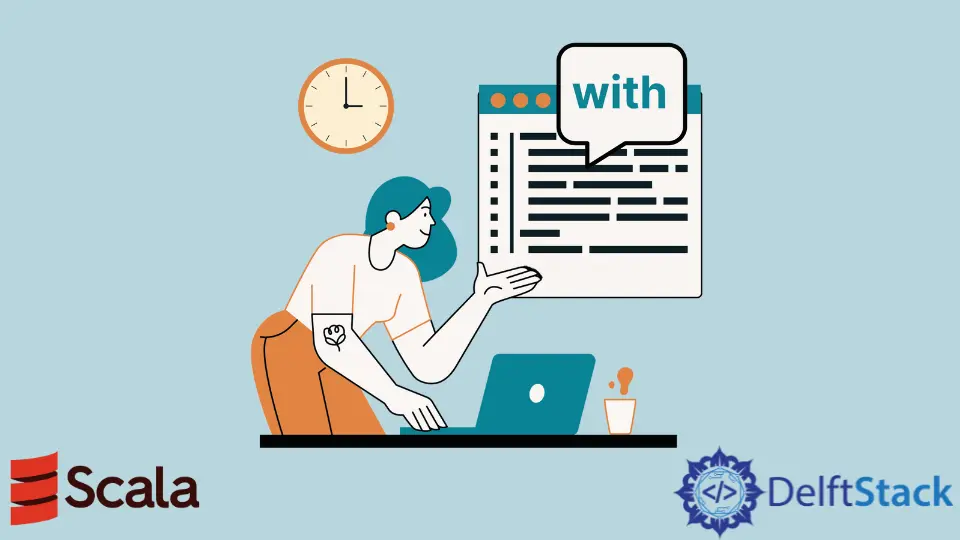
이 기사에서는 Scala에서 with
키워드를 사용하는 방법을 보여줍니다.
Scala에서 with
키워드 사용
이 키워드는 일반적으로 믹스인으로 클래스 구성을 처리할 때 사용됩니다. 믹스인은 클래스를 구성하는 데 사용되는 특성입니다.
이는 인터페이스를 구현할 수 있는 Java 클래스와 다소 유사합니다.
with
키워드를 더 잘 이해하기 위한 예를 살펴보겠습니다.
abstract class vehicle {
val message: String
}
class Car extends vehicle {
val message = "I'm an instance of class vehicle"
}
trait sportsCar extends vehicle {
def ourMessage = message.toUpperCase()
}
class temp extends Car with sportsCar
val obj = new temp
println(obj.message) // I'm an instance of class vehicle
println(obj.ourMessage) // I'M AN INSTANCE OF CLASS VEHICLE
위의 코드에서 클래스 temp
에는 상위 클래스 Car
가 있고 sportsCar
를 혼합합니다. 클래스는 하나의 수퍼클래스만 확장할 수 있지만 with
키워드를 사용하여 많은 믹스인을 확장할 수 있습니다. 슈퍼클래스와 믹스인은 동일한 슈퍼타입
을 가질 수 있습니다.
다른 예를 살펴보겠습니다. 먼저 T
유형과 몇 가지 표준 반복기 메서드를 사용하여 추상 클래스를 생성해 보겠습니다.
abstract class ourIterator {
type T
def hasNext: Boolean
def next(): T
}
이제 hasNext
및 next
인 T
의 모든 추상 멤버 구현이 있는 구체적인 클래스를 생성해 보겠습니다.
class StringIterator(str: String) extends ourIterator {
type T = Char
private var i = 0
def hasNext = i < str.length
def next() =
{
val ch = str.charAt(i)
i += 1
ch
}
}
ourIterator
클래스를 확장하는 특성을 만들어 봅시다.
trait anotherIterator extends ourIterator {
def foreach(X: T => Unit): Unit = while (hasNext) X(next())
}
특성 anotherIterator
는 while(hasNext)
요소가 더 있는 한 다음 요소 next()
에서 계속해서 X: T => Unit
함수를 호출하는 foreach()
메서드를 구현합니다.
anotherIterator
는 특성이므로 ourIterator
클래스의 추상 멤버를 구현할 필요가 없습니다. 이제 아래와 같이 anotherIterator
및 StringIterator
의 기능을 단일 클래스로 결합할 수 있습니다.
class temp extends StringIterator("Tony") with anotherIterator
val obj = new temp
obj.foreach(println)
전체 작업 코드:
abstract class ourIterator {
type T
def hasNext: Boolean
def next(): T
}
class StringIterator(str: String) extends ourIterator {
type T = Char
private var i = 0
def hasNext = i < str.length
def next() =
{
val ch = str.charAt(i)
i += 1
ch
}
}
trait anotherIterator extends ourIterator {
def foreach(X: T => Unit): Unit = while (hasNext) X(next())
}
class temp extends StringIterator("Tony") with anotherIterator
val obj = new temp
obj.foreach(println)
출력:
T
o
n
y
위의 코드에서 새 클래스 temp
에는 superclass
로 StringIterator
가 있고 mixin으로 anotherIterator
가 있습니다. 단일 상속만으로는 이러한 수준의 유연성을 달성할 수 없습니다.