React의 상위 구성 요소에서 하위 함수 호출
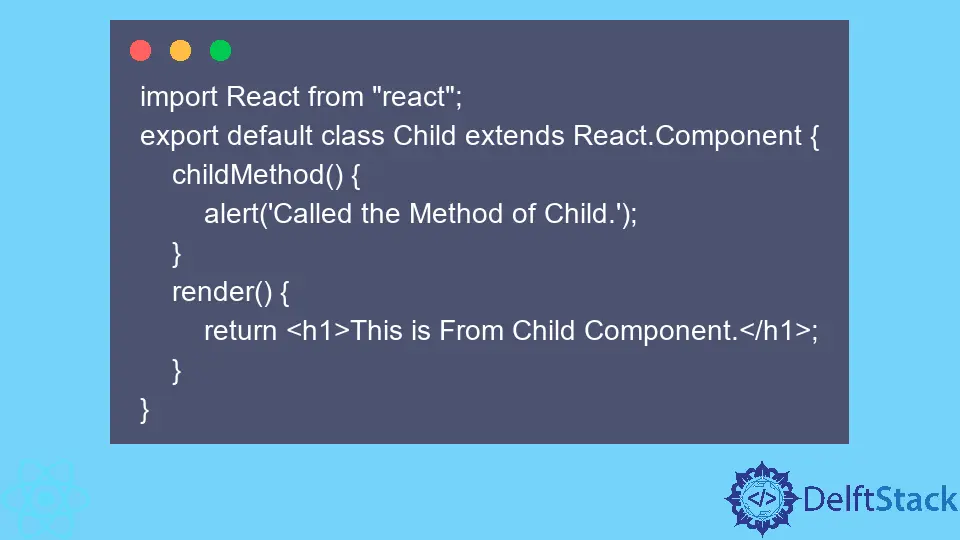
이번 글에서는 React에서 자식 컴포넌트의 메소드를 부모 컴포넌트로 호출하는 방법에 대해 알아보겠습니다.
대부분 개발자는 자식 구성 요소에서 부모 구성 요소의 메서드를 호출해야 하며 부모 구성 요소 메서드를 props
로 자식 구성 요소에 전달하여 이를 달성할 수 있습니다.
뒤집을 수도 있습니다. 여기서는 refs
를 사용하여 부모에서 자식 구성 요소의 메서드를 호출합니다.
React의 ref
소개
일반적으로 자식 구성 요소에 props를 전달하여 부모 구성 요소에서 자식 구성 요소와 상호 작용할 수 있습니다. ref
를 사용하면 일반적인 부모 및 자식 데이터 흐름 외부의 구성 요소와 상호 작용할 수 있습니다.
사용자는 아래 구문에 따라 ref
를 생성할 수 있습니다.
this.newRef = React.createRef();
ref
를 생성한 후 사용자는 이를 HTML 요소 또는 구성 요소의 ref
특성 값으로 할당할 수 있습니다. Child
구성 요소의 ref
속성에 할당하려고 합니다.
<Child ref={this.newRef} />
이제 사용자는 newRef
의 current
속성을 통해 하위 구성 요소의 마운트된 인스턴스에 액세스하고 하위 구성 요소의 메서드를 호출할 수 있습니다.
this.childRef.current.childMethod();
따라서 이러한 방식으로 ref
는 일반적인 데이터 흐름 외부의 모든 구성 요소와 상호 작용하는 데 유용합니다.
ref
를 사용하여 클래스 구성 요소의 부모에서 자식 함수 호출
아래 예에서는 App
(부모)과 Child
라는 두 가지 구성 요소를 만들었습니다. Child
구성 요소에서 렌더링할 일부 HTML과 호출 시 경고 상자를 표시하는 childMethod()
를 추가했습니다.
App
구성 요소에서 생성자에 ref
를 생성하고 해당 값을 Child
구성 요소의 ref
속성에 할당했습니다.
사용자가 click
버튼을 클릭하면 callChild()
메서드가 호출됩니다. callChild()
메서드는 ref
의 current
속성을 통해 childMethod()
에 액세스하고 호출합니다.
예제 코드:
// import required libraries
import React from "react";
import Child from "./Child";
export default class APP extends React.Component {
constructor(props) {
super(props);
// Initializing the Refs
this.childRef = React.createRef();
}
// call the child method
callChild = () => {
this.childRef.current.childMethod();
};
render() {
return (
<div>
{/* Passing Ref to CHild component */}
<Child ref={this.childRef} />
<button onClick={this.callChild}>Click</button>
</div>
);
}
}
import React from "react";
export default class Child extends React.Component {
childMethod() {
alert('Called the Method of Child.');
}
render() {
return <h1>This is From Child Component.</h1>;
}
}
출력:
후크를 사용하여 기능 구성 요소의 부모에서 자식 기능 호출
아래 예에서는 App
과 Child
라는 두 개의 함수 구성 요소를 만들었습니다. useRef
후크를 사용하여 상위 구성 요소 내부의 Child
구성 요소에 액세스하기 위해 forwardRef
후크 내부에 Child
구성 요소를 래핑했습니다.
또한 useImperativeHandle
을 사용하고 ref
를 첫 번째 매개변수로, 콜백 함수를 두 번째 매개변수로 전달했습니다. 여기에서 Child
구성 요소는 콜백 함수가 반환하는 모든 것을 확장합니다.
useRef
후크의 current
특성을 통해 상위 구성 요소의 콜백 함수에서 반환된 값에 액세스할 수 있습니다.
예제 코드 - (App.js
):
// import required libraries
import React, { useRef } from "react";
import Child from "./Child";
export default function APP() {
// use hooks to create `ref`
const childRef = useRef();
// call child method
const onClick = () => {
childRef.current.childMethod();
};
return (
<div>
{/* Passing Ref to CHild component */}
{/* use `refs` */}
<Child ref={childRef} />
<button onClick={onClick}>Click</button>
</div>
);
}
예제 코드 - (Child.js
):
import React, { forwardRef, useImperativeHandle } from "react";
// While using the hooks, it is required to wrap the component inside the forwardRef to gain access to the ref object,
//, which we create using the `useref` hooks inside the parent component.
const Child = forwardRef((props, ref) => {
// Extending the child component with the return value of the callback function passed as a second parameter of the useImperatuveHandle.
useImperativeHandle(ref, () => ({
childMethod() {
alert("Called the Method of Child.");
},
}));
return <h1>Use Refs in functional component.</h1>;
});
export default Child;
출력:
ref
를 사용하여 부모 함수에서 자식 함수를 호출하는 방법을 배웠습니다. 또한 사용자는 ref
를 사용하여 상위 구성 요소를 통해 하위 구성 요소를 변경할 수 있습니다.