React 형식 전화번호
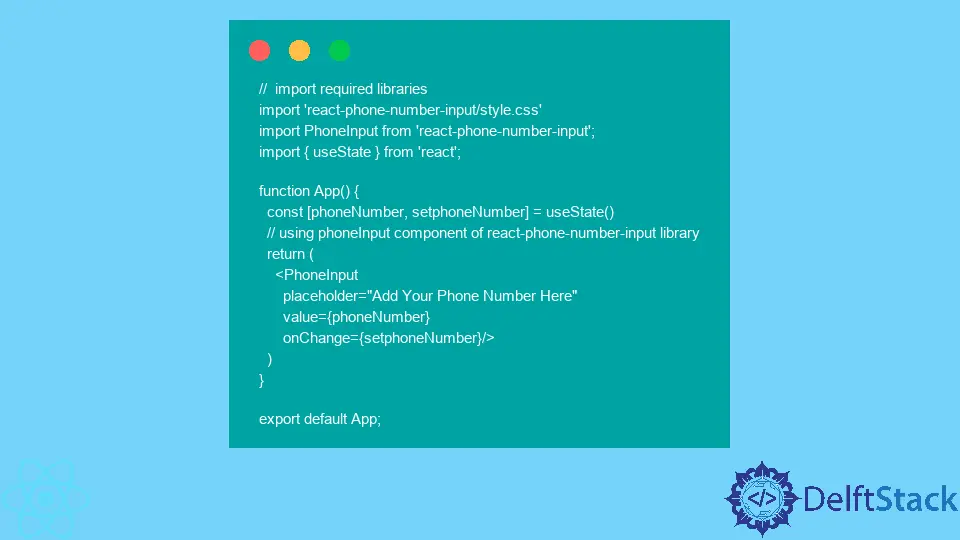
이 기사에서는 React에서 전화번호 형식을 지정하는 방법을 배웁니다. 개발자는 종종 React 애플리케이션을 개발하는 동안 입력 값을 형식화하는 문제에 직면합니다.
여기서는 (###) ### - ####
형식으로 10자리 전화 번호를 형식화합니다. 우리의 목표를 달성하기 위해 첫 번째 예에서 사용자 지정 논리를 만들고 두 번째 예에서 React 내장 라이브러리를 사용했습니다.
전화번호 형식을 지정하는 사용자 지정 논리 만들기
요구 사항에 따라 전화 번호의 형식을 지정하는 사용자 지정 논리를 만들 수 있습니다. 사용자는 아래 단계에 따라 전화번호의 형식을 지정할 수 있습니다.
-
입력이 비어 있으면 빈 문자열 또는 값을 반환합니다.
if (!input) return input;
-
입력에서 숫자를 필터링하고 다른 문자를 제거합니다.
const numberInput = input.replace(/[^\d]/g, "");
-
전화번호 입력 값의 길이를 취합니다.
const numberInputLength = numberInput.length;
-
입력한 길이가 4보다 작으면 전화번호를 그대로 돌려준다.
if (numberInputLength < 4) { return numberInput; }
-
입력 값의 길이가 4에서 7 사이인 경우
(###) #__
과 같이 형식을 지정합니다.if (numberInputLength < 7) { return `(${numberInput.slice(0, 3)}) ${numberInput.slice(3)}`; }
-
그리고 입력 값의 길이가 10보다 크면
(###) ### - #___
와 같이 형식을 지정합니다.if{ return `(${numberInput.slice(0, 3)}) ${numberInput.slice(3,6)}-${numberInput.slice(6, 10)}`; }
아래 예제 코드에서 전화번호 입력을 받기 위해 <input>
을 생성했습니다. 입력 값이 변경될 때마다 handlephoneNumber()
함수를 호출합니다.
handlephoneNumber()
함수는 formatPhoneNumber()
함수를 호출하여 위의 단계에 따라 전화번호의 형식을 지정합니다. 전화번호를 포맷한 후 handlephoneNumber()
함수는 전화번호의 포맷된 값을 입력으로 설정합니다.
예제 코드:
// import react and usestate
import React, { useState } from "react";
function formatPhoneNumber(input) {
// if the input is null, return a null value
if (!input) return input;
// remove all characters from the input except number input.
const numberInput = input.replace(/[^\d]/g, "");
// take the length of the value of the input
const numberInputLength = numberInput.length;
// if the number length is 1, 2, or 3, then return it as it is.
if (numberInputLength < 4) {
return numberInput;
} else if (numberInputLength < 7) {
// if the number input length is 4, 5, or 6, format it accordingly.
return `(${numberInput.slice(0, 3)}) ${numberInput.slice(3)}`;
} else {
// if the number input length is 7, 8, 9, 10, or more, format it like the below.
return `(${numberInput.slice(0, 3)}) ${numberInput.slice(
3,
6
)}-${numberInput.slice(6, 10)}`;
}
// return empty string in case any condition doesn't satisfy.
return "";
}
export default function App() {
// Binded phoneNumber with an input value and setPhoneNUmber is used to make changes to the value of phoneNumber
const [phoneNumber, setphoneNumber] = useState("");
// whenever input will change, handlephoneNumber() function will invoke.
const handlephoneNumber = (e) => {
// format phone number
const formattedPhoneNumber = formatPhoneNumber(e.target.value);
// set the formatted phone number to the input value
setphoneNumber(formattedPhoneNumber);
};
return (
<div style={{ textAlign: "center", marginTop: "30px" }}>
{/* number input to take phone numbetr */}
<input onChange={(e) => handlephoneNumber(e)} value={phoneNumber} />
</div>
);
}
출력:
위의 출력에서 사용자는 입력 값의 길이에 따라 형식이 지정된 전화 번호를 볼 수 있습니다. 또한 사용자가 숫자를 제외한 다른 문자를 입력에 추가하는 것을 허용하지 않습니다.
또한 사용자는 길이가 10을 초과하는 숫자를 입력할 수 없습니다.
react-phone-number-input
라이브러리를 사용하여 국가에 따라 전화번호 형식 지정
React의 react-phone-number-input
라이브러리를 사용하면 다른 국가에 따라 전화번호 형식을 지정할 수 있습니다. 코드에서 라이브러리를 가져오고 <phoneInput>
구성 요소를 사용하여 자동으로 전화 번호 형식을 지정하는 전화 번호 입력을 가져올 수 있습니다.
예제를 살펴보기 전에 사용자는 React 앱에 라이브러리를 설치해야 합니다. 이를 위해 React 앱이 있는 동일한 디렉터리에서 터미널을 열고 다음 명령을 입력합니다.
npm install react-phone-number-input
이제 사용자는 아래와 같이 앱에서 <phoneInput>
컴포넌트를 사용해야 합니다. 여기에서 phoneNumber
변수는 입력 값을 추적하고 setPhoneNumber()
함수는 사용자가 입력 값을 변경할 때 값을 phoneNumber
로 설정합니다.
<PhoneInput value={phoneNumber} onChange={setphoneNumber}/>
한 줄의 코드로 전화 번호 형식을 구현했습니다. 사용자는 아래에서 전체 작업 코드를 볼 수 있습니다.
예제 코드:
// import required libraries
import 'react-phone-number-input/style.css'
import PhoneInput from 'react-phone-number-input';
import { useState } from 'react';
function App() {
const [phoneNumber, setphoneNumber] = useState()
// using phoneInput component of react-phone-number-input library
return (
<PhoneInput
placeholder="Add Your Phone Number Here"
value={phoneNumber}
onChange={setphoneNumber}/>
)
}
export default App;
출력:
위 출력에서 사용자는 드롭다운에서 선택한 국가에 따라 전화번호의 형식을 지정하는 것을 볼 수 있습니다. 또한 전화번호의 길이에 따라 국가를 자동으로 선택합니다.
두 번째 방법은 코드 작성이 더 간단하지만 사용자가 숫자를 입력하는 것을 막지는 않습니다. 사용자는 원하는 길이의 전화번호를 입력할 수 있습니다. 이는 애플리케이션을 개발하는 동안 좋은 습관이 아닙니다.
첫 번째 방법에서 작성한 것처럼 사용자 지정 논리를 작성하여 요구 사항에 따라 전화 번호를 사용자 지정할 수 있습니다. 따라서 프로그래머는 사용자 지정 논리를 사용하는 것이 좋습니다.