Python에서 defaultdict 사용
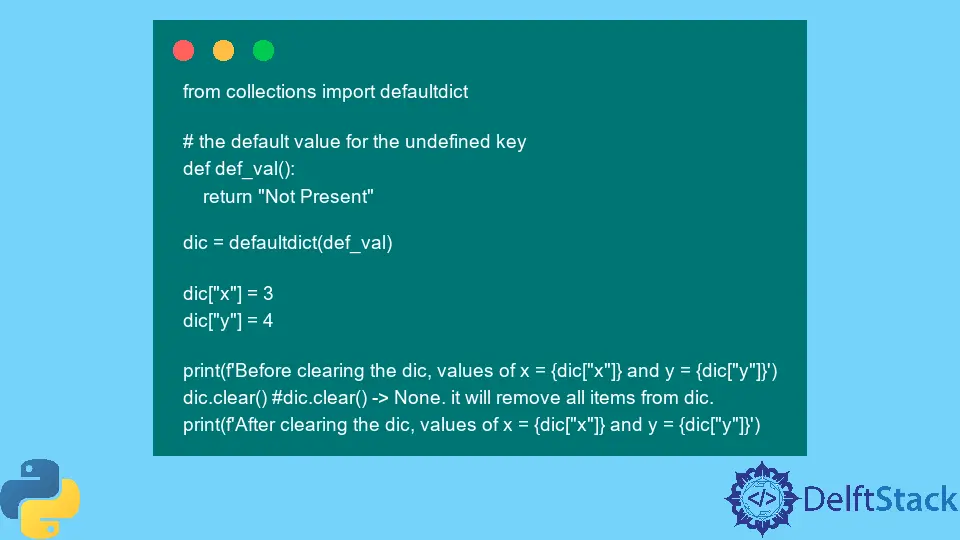
오늘의 기사에서는 defaultdict
컨테이너에 대해 설명하고 코드 예제를 사용하여 사용법을 보여줍니다.
Python의 defaultdict
대 dict
defaultdict
는 collections 모듈에 속하는 사전과 같은 컨테이너입니다. 사전의 하위 클래스입니다. 따라서 사전
의 모든 기능이 있습니다. 그러나 defaultdict
의 유일한 목적은 KeyError
를 처리하는 것입니다.
# return true if the defaultdict is a subclass of dict (dictionary)
from collections import defaultdict
print(issubclass(defaultdict, dict))
출력:
True
사용자가 사전
에서 항목을 검색했는데 검색된 항목이 존재하지 않는다고 가정해 보겠습니다. 일반 사전에서는 항목이 존재하지 않음을 의미하는 KeyError
가 발생합니다. 이 문제를 극복하기 위해 Python 개발자는 defaultdict
라는 개념을 제시했습니다.
코드 예:
# normal dictionary
ord_dict = {"x": 3, "y": 4}
ord_dict["z"] # --> KeyError
출력:
KeyError: 'z'
일반 사전은 알 수 없는 키
를 처리할 수 없으며 위의 코드에서 설명한 것처럼 알 수 없는 키
를 검색할 때 KeyError
를 발생시킵니다.
반면 defaultdict
모듈은 Python 사전과 유사하게 작동합니다. 그럼에도 불구하고 유용하고 사용자 친화적인 고급 기능이 있으며 사용자가 사전에서 정의되지 않은 키를 검색할 때 오류가 발생하지 않습니다.
그러나 대신 사전에 항목을 만들고 키
에 대한 기본값을 반환합니다. 이 개념을 이해하기 위해 아래의 실제 부분을 살펴보겠습니다.
코드 예:
from collections import defaultdict
# the default value for the undefined key
def def_val():
return "The searched Key Not Present"
dic = defaultdict(def_val)
dic["x"] = 3
dic["y"] = 4
# search 'z' in the dictionary 'dic'
print(dic["z"]) # instead of a KeyError, it has returned the default value
print(dic.items())
출력:
The searched Key Not Present
dict_items([('x', 3), ('y', 4), ('z', 'The searched Key Not Present')])
defaultdict
는 사전에 정의되지 않은 키로 액세스하려는 모든 항목을 생성합니다.
그리고 이러한 default
항목을 생성하면 defaultdict
의 생성자에게 전달하는 함수 객체를 호출하며, 더 정확하게 말하면 객체는 유형 객체와 함수를 포함하는 호출 가능한
객체여야 합니다.
위의 예에서 기본 항목은 사전의 정의되지 않은 키에 대해 문자열 The searched Key Not Present
를 반환하는 def_val
함수로 생성됩니다.
Python의 defaultdict
default_factory
는 __missing__()
메서드에서 사용되는 defaultdict
생성자의 첫 번째 인수이며 생성자의 인수가 누락된 경우 default_factory
는 None
으로 초기화됩니다. KeyError
가 발생합니다.
그리고 default_factory
가 None
이외의 것으로 초기화되면 위의 예에서 볼 수 있듯이 검색된 key
에 value
로 할당됩니다.
파이썬에서 defaultdict
의 유용한 기능
dictionary
및 defaultdict
기능이 많이 있습니다. 아시다시피 defaultdict
는 dictionary
의 모든 기능에 액세스할 수 있습니다. 그러나 이들은 defaultdict
에 특정한 가장 유용한 기능 중 일부입니다.
Python의 defaultdict.clear()
코드 예:
from collections import defaultdict
# the default value for the undefined key
def def_val():
return "Not Present"
dic = defaultdict(def_val)
dic["x"] = 3
dic["y"] = 4
print(f'Before clearing the dic, values of x = {dic["x"]} and y = {dic["y"]}')
dic.clear() # dic.clear() -> None. it will remove all items from dic.
print(f'After clearing the dic, values of x = {dic["x"]} and y = {dic["y"]}')
출력:
Before clearing the dic, values of x = 3 and y = 4
After clearing the dic, values of x = Not Present and y = Not Present
위의 예에서 볼 수 있듯이 사전 dic
에는 x=3
및 y=4
인 두 쌍의 데이터가 있습니다. 그러나 clear()
함수를 사용한 후 데이터가 제거되고 x
및 y
의 값이 더 이상 존재하지 않으므로 x
및 y
에 대해 없음
이 표시됩니다.
Python의 defaultdict.copy()
코드 예:
from collections import defaultdict
# the default value for the undefined key
def def_val():
return "Not Present"
dic = defaultdict(def_val)
dic["x"] = 3
dic["y"] = 4
dic_copy = dic.copy() # dic.copy will give you a shallow copy of dic.
print(f"dic = {dic.items()}")
print(f"dic_copy = {dic_copy.items()}")
출력:
dic = dict_items([('x', 3), ('y', 4)])
dic_copy = dict_items([('x', 3), ('y', 4)])
defaultdict.copy()
함수는 사전의 얕은 복사본을 그에 따라 사용할 수 있는 다른 변수에 복사하는 데 사용됩니다.
Python의 defaultdict.default_factory()
코드 예:
from collections import defaultdict
# the default value for the undefined key
def def_val():
return "Not present"
dic = defaultdict(def_val)
dic["x"] = 3
dic["y"] = 4
print(f"The value of z = {dic['Z']}")
print(
dic.default_factory()
) # default_factory returns the default value for defaultdict.
출력:
The value of z = Not present
Not present
default_factory()
함수는 정의된 클래스의 속성에 기본값을 제공하는 데 사용되며 일반적으로 default_factory
의 값은 함수가 반환하는 값입니다.
Python의 defaultdict.get(키, 기본값)
코드 예:
from collections import defaultdict
# the default value for the undefined key
def def_val():
return "Not present"
dic = defaultdict(def_val)
dic["x"] = 3
dic["y"] = 4
# search the value of Z in the dictionary dic; if it exists, return the value; otherwise, display the message
print(dic.get("Z", "Value doesn't exist")) # default value is None
출력:
Value doesn't exist
defaultdict.get()
함수는 두 개의 인수를 사용합니다. 첫 번째는 키이고 두 번째는 값이 존재하지 않는 경우 키에 대한 기본값입니다.
그러나 두 번째 인수는 선택 사항입니다. 따라서 어떤 메시지나 값도 지정할 수 있습니다. 그렇지 않으면 없음
이 기본값으로 표시됩니다.
Zeeshan is a detail oriented software engineer that helps companies and individuals make their lives and easier with software solutions.
LinkedIn