Python의 Smith-Waterman 알고리즘
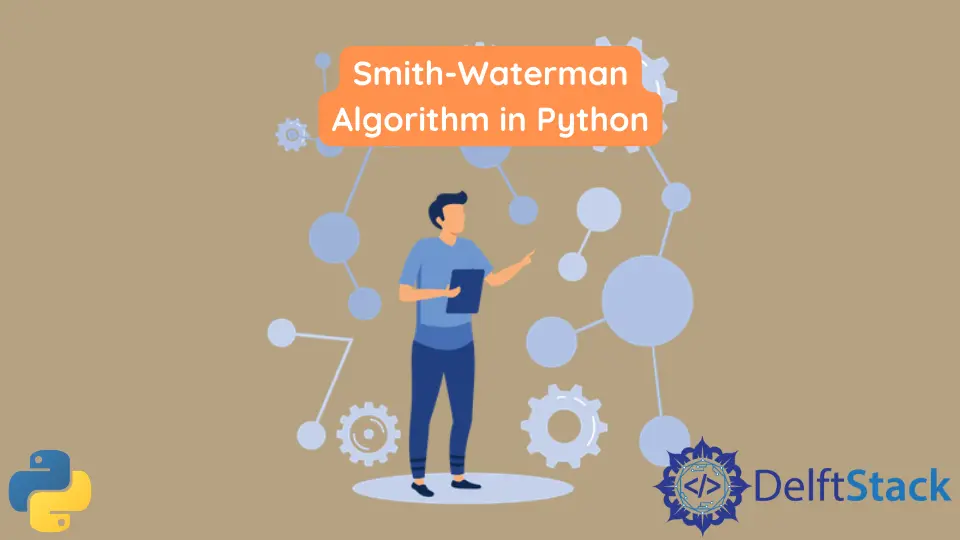
Smith-Waterman 알고리즘은 문자열의 로컬 시퀀스 정렬을 수행하는 데 사용됩니다. 문자열은 대부분 DNA 가닥 또는 단백질 서열을 나타냅니다.
이 기사에서는 Python에서 Smith-Waterman 알고리즘을 구현하는 방법에 대해 설명합니다.
Python의 Smith-Waterman 알고리즘
swalign
모듈에는 Python에서 Smith-Waterman 알고리즘을 구현하는 여러 함수가 포함되어 있습니다. 명령줄에서 다음 명령문을 실행하여 PIP
를 사용하여 swalign
모듈을 설치할 수 있습니다.
pip3 install swalign
위의 명령문은 Python 버전 3용 모듈을 설치합니다. Python 버전 2에 모듈을 설치하려면 다음 명령을 사용할 수 있습니다.
pip install swalign
swalign
모듈을 설치한 후 다음 단계를 사용하여 Python 프로그램에서 Smith-Waterman 알고리즘을 구현합니다.
-
먼저
import
문을 사용하여swalign
모듈을 가져옵니다. -
정렬을 수행하려면 뉴클레오티드 스코어링 매트릭스를 만들어야 합니다. 매트릭스에서 각 일치 및 불일치에 대한 점수를 제공합니다.
일반적으로 일치 점수에는 2를 사용하고 불일치에는 -1을 사용합니다.
-
뉴클레오티드 스코어링 매트릭스를 생성하기 위해
NucleotideScoringMatrix()
방법을 사용합니다.NucleotideScoringMatrix()
는 첫 번째 입력 인수로 일치 점수를 사용하고 두 번째 입력 인수로 불일치 점수를 사용합니다.실행 후
IdentityScoringMatrix
개체를 반환합니다. -
뉴클레오타이드 매트릭스를 얻으면
LocalAlignment()
메소드를 사용하여LocalAlignment
객체를 생성합니다.LocalAlignment()
메소드는 뉴클레오티드 스코어링 매트릭스를 입력으로 사용하고LocalAlignment
객체를 반환합니다. -
LocalAlignment
객체를 가져오면align()
메서드를 사용하여 Smith-Waterman 알고리즘을 실행할 수 있습니다. -
LocalAlignment
객체에서 호출되는align()
메소드는 첫 번째 입력 인수로 DNA 가닥을 나타내는 문자열을 사용합니다. 참조 DNA 가닥을 나타내는 또 다른 문자열이 필요합니다. -
실행 후
align()
메서드는Alignment
객체를 반환합니다.Alignment
개체에는 입력 문자열의 일치 세부 정보 및 불일치 및 기타 여러 세부 정보가 포함되어 있습니다.
다음 예제에서 전체 프로세스를 관찰할 수 있습니다.
import swalign
dna_string = "ATCCACAGC"
reference_string = "ATGCAGCGC"
match_score = 2
mismatch_score = -1
matrix = swalign.NucleotideScoringMatrix(match_score, mismatch_score)
lalignment_object = swalign.LocalAlignment(matrix)
alignment_object = lalignment_object.align(dna_string, reference_string)
alignment_object.dump()
출력:
Query: 1 ATGCAGC-GC 9
||.|| | ||
Ref : 1 ATCCA-CAGC 9
Score: 11
Matches: 7 (70.0%)
Mismatches: 3
CIGAR: 5M1I1M1D2M
결론
이 기사에서는 Python의 swalign
모듈을 사용하여 Smith-Waterman 알고리즘을 구현하는 방법에 대해 설명합니다.
Aditya Raj is a highly skilled technical professional with a background in IT and business, holding an Integrated B.Tech (IT) and MBA (IT) from the Indian Institute of Information Technology Allahabad. With a solid foundation in data analytics, programming languages (C, Java, Python), and software environments, Aditya has excelled in various roles. He has significant experience as a Technical Content Writer for Python on multiple platforms and has interned in data analytics at Apollo Clinics. His projects demonstrate a keen interest in cutting-edge technology and problem-solving, showcasing his proficiency in areas like data mining and software development. Aditya's achievements include securing a top position in a project demonstration competition and gaining certifications in Python, SQL, and digital marketing fundamentals.
GitHub