Python에서 이메일 주소 확인
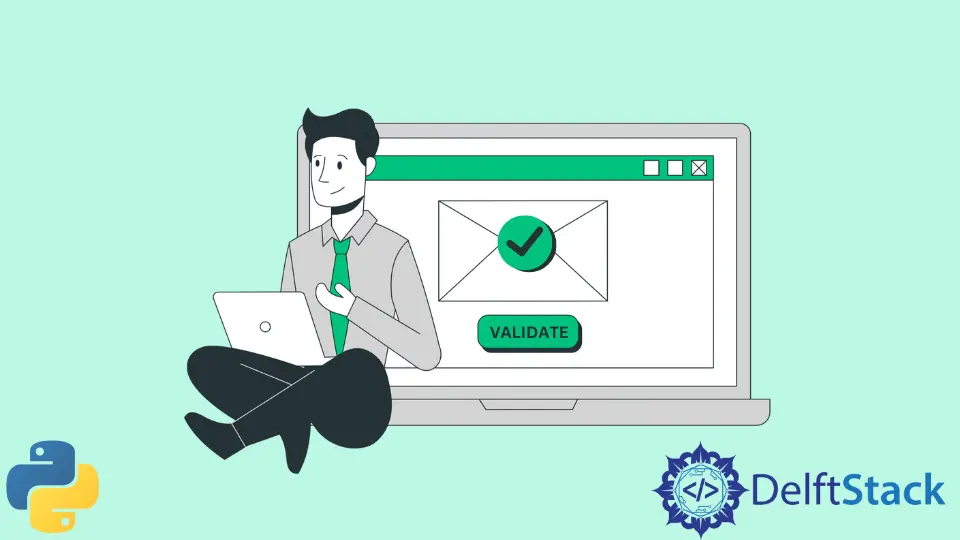
이메일 유효성 검사는 폐쇄형 애플리케이션 내 사용자 여정의 중요한 부분이며 특히 보안 및 사용자 이탈 감소에 중요합니다. 이메일을 검증하는 방법에는 여러 가지가 있으며 일반적인 이메일 주소의 구조를 이해하는 것이 중요합니다.
이러한 프로세스에 도움이 되는 regex
와 같은 내장 기능과 dnspython
과 같은 라이브러리가 있습니다. 이 기사에서는 이러한 기능과 라이브러리를 사용하여 Python에서 이메일의 유효성을 검사하는 방법을 설명합니다.
regex
를 사용하여 Python에서 이메일 유효성 검사
일반적으로 정규식
이라고 하는 정규식은 텍스트에서 검색 패턴을 지정하는 일련의 문자이며 문자열에서 검색 알고리즘과 찾기
및 찾기 및 바꾸기
를 수행하는 데 유용합니다.
regex
를 사용하면 사용자로부터 받은 이메일 주소 내에서 특정 패턴을 찾을 수 있습니다. 모든 이메일 주소의 패턴은 [username]@[smtp server].com
입니다.
정규식
을 사용하여 사용자 이름
부분과 SMTP 서버
부분을 찾을 수 있습니다. 정규식
을 사용하는 방법을 이해하려면 메타문자, 특수 시퀀스 및 집합에 대해 알아야 합니다.
이 지식을 사용하여 compile
메서드를 사용하여 regex
패턴을 만들고 컴파일하고 match
메서드를 사용하여 사용자 이메일 주소의 문자열을 비교하여 확인합니다. Python에서 regex
를 사용하려면 정규식을 지원하는 re
라이브러리가 필요합니다.
import re
user_email = input("Enter your Email Address: ")
pattern = re.compile(r"\"?([-a-zA-Z0-9.`?{}]+@\w+\.\w+)\"?")
if not re.match(pattern, user_email):
print(f"{user_email} is not a valid email address")
else:
print(f"Your {user_email} is a valid email address")
출력:
PS C:\Users\akinl\Documents\Python> python email.py
Enter your Email Address: akin@gm.com
Your akin@gm.com is a valid email address
PS C:\Users\akinl\Documents\Python> python email.py
Enter your Email Address: dx@s
dx@s is not a valid email address
\"?([-a-zA-Z0-9.?{}]+@\w+\.\w+)\"?
문자열에는 사용자의 이메일 주소와 비교할 정규식 패턴이 포함되어 있습니다.
첫 번째 입력인 akin@gm.com
을 사용하면 전자 메일에는 사용자 이름에서 SMTP 서버로 유효한 전자 메일을 만드는 데 필요한 모든 패턴과 구조가 포함됩니다. 그러나 dx@s
의 경우 SMTP 서버가 존재하지 않습니다.
Python 3.x에서 가장 선호되는 fullmatch
방법을 사용하여 일치 여부를 확인할 수도 있습니다. 코드는 비슷하지만 match
메서드를 fullmatch
로 변경하기만 하면 됩니다.
match
와 fullmatch
의 차이점은 match
는 처음에만 일치하는 반면 fullmatch
는 문자열 텍스트 전체를 끝까지 일치시킨다는 것입니다.
import re
user_email = input("Enter your Email Address: ")
pattern = re.compile(r"\"?([-a-zA-Z0-9.`?{}]+@\w+\.\w+)\"?")
if not re.fullmatch(pattern, user_email):
print(f"{user_email} is not a valid email address")
else:
print(f"Your {user_email} is a valid email address")
출력:
PS C:\Users\akinl\Documents\Python> python email.py
Enter your Email Address: akin@gmail.com
Your akin@gmail.com is a valid email address
이를 통해 유효한 이메일 구조를 확인할 수 있지만 똑같이 중요한 SMTP 서버의 존재 여부는 확인할 수 없습니다. 다음 기능이 들어오는 곳입니다.
dnspython
을 사용하여 Python에서 이메일 유효성 검사
dnspython
라이브러리를 사용하면 이메일의 SMTP 부분을 검증하여 실제인지 구성되지 않았는지 확인할 수 있습니다. 이메일을 확인하고 유효성을 검사한 후 SMTP 서버의 일부를 분할하고 resolve
메서드를 사용하여 확인할 수 있습니다.
dnspython
은 내장 라이브러리가 아니므로 pip
를 통해 설치해야 합니다.
pip install dnspython
dnspython
을 설치한 후 이를 코드로 가져오고 resolve
방법을 사용하여 유효한 SMTP 서버를 확인할 수 있습니다.
import dns.resolver
try:
answers = dns.resolver.resolve("google.com", "MX")
for rdata in answers:
print("Host", rdata.exchange, "has preference", rdata.preference)
print(bool(answers))
except dns.exception.DNSException as e:
print("Email Doesn't Exist")
출력:
Host smtp.google.com. has preference 10
True
SMTP 서버를 확인하기 위해 resolve
메서드에 MX
속성을 전달하여 mail exchange
레코드를 확인하고 반환된 rdata
는 exchange
및 preference
속성 값을 확인하는 데 유용합니다.
그러나 중요한 것은 bool
함수를 사용하여 answer
바인딩 내에 값이 있는지 확인하는 것입니다. 값이 없으면 SMTP 서버
가 유효하지 않음을 의미합니다.
포괄적인 이메일 유효성 검사를 위해 regex
및 dnspython
코드를 결합할 수 있습니다.
import dns.resolver
import re
user_email = input("Enter your Email Address: ")
pattern = re.compile(r"\"?([-a-zA-Z0-9.`?{}]+@\w+\.\w+)\"?")
if not re.fullmatch(pattern, user_email):
print(f"{user_email} is not a valid email address.")
else:
domain = user_email.rsplit("@", 1)[-1]
try:
answers = dns.resolver.resolve(domain, "MX")
if bool(answers):
print(f"Your {user_email} is a valid email address.")
except dns.exception.DNSException as e:
print("Not a valid email server.")
출력:
PS C:\Users\akinl\Documents\Python> python testEmail.py
Enter your Email Address: akin@gmail.com
Your akin@gmail.com is a valid email address.
PS C:\Users\akinl\Documents\Python> python testEmail.py
Enter your Email Address: ds.cem@fex.co
Your ds.cem@fex.co is a valid email address.
PS C:\Users\akinl\Documents\Python> python testEmail.py
Enter your Email Address: wer@fghj.com
Your wer@fghj.com is a valid email address.
PS C:\Users\akinl\Documents\Python> python testEmail.py
Enter your Email Address: df@werabsjaksa.com
Not a valid email server.
PS C:\Users\akinl\Documents\Python> python testEmail.py
Enter your Email Address: des@python.exe
Not a valid email server.
try/except
섹션은 SMTP 서버
가 존재하지 않을 때 특정 예외를 처리하는 데 도움이 됩니다.
Olorunfemi is a lover of technology and computers. In addition, I write technology and coding content for developers and hobbyists. When not working, I learn to design, among other things.
LinkedIn