Python 모듈의 모든 메서드 나열
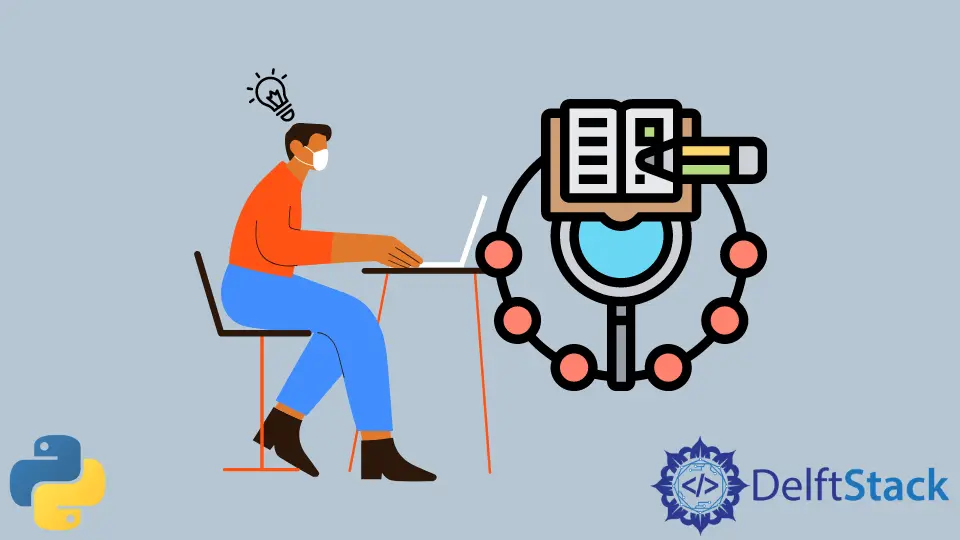
Python 모듈, 패키지 또는 라이브러리는 Python 함수, Python 클래스 및 Python 변수의 정의를 포함하는 파일 또는 파일 그룹입니다. 파이썬에서 우리는 모듈을 가져오고 그 구현을 사용하여 컴퓨터 과학 세계의 두 가지 중요한 개념을 고수할 수 있습니다. “바퀴를 재발명하지 마십시오"와 “반복하지 마십시오.”
이러한 패키지 또는 모듈은 몇 줄만큼 작을 수 있고 수백만 줄만큼 클 수 있습니다. 크기가 커질수록 모듈을 분석하거나 패키지 내용의 명확한 개요를 보기가 어려워집니다. 그러나 Python 개발자는 우리를 위해 그 문제도 해결했습니다.
파이썬에서는 파이썬 모듈의 메소드와 클래스를 나열할 수 있는 많은 방법이 있습니다. 이 기사에서는 관련 예를 통해 이러한 두 가지 관행에 대해 이야기할 것입니다. 예를 들어 NumPy
Python 모듈을 고려할 것입니다. 시스템이나 가상 환경에 NumPy
패키지가 없는 경우 pip install numpy
또는 pip3 install numpy
명령을 사용하여 다운로드할 수 있습니다.
dir()
메서드를 사용하여 Python 모듈의 모든 메서드 나열
dir()
메서드는 Python에 내장된 메서드입니다. 객체 또는 Python 패키지의 모든 속성과 메서드를 인쇄합니다. 다음 코드를 확인하여 이해하세요.
class A:
a = 10
b = 20
c = 200
def __init__(self, x):
self.x = x
def get_current_x(self):
return self.x
def set_x(self, x):
self.x = x
def __repr__(self):
return f"X: {x}"
print(dir(int))
print(dir(float))
print(dir(A))
출력:
['__abs__', '__add__', '__and__', '__bool__', '__ceil__', '__class__', '__delattr__', '__dir__', '__divmod__', '__doc__', '__eq__', '__float__', '__floor__', '__floordiv__', '__format__', '__ge__', '__getattribute__', '__getnewargs__', '__gt__', '__hash__', '__index__', '__init__', '__init_subclass__', '__int__', '__invert__', '__le__', '__lshift__', '__lt__', '__mod__', '__mul__', '__ne__', '__neg__', '__new__', '__or__', '__pos__', '__pow__', '__radd__', '__rand__', '__rdivmod__', '__reduce__', '__reduce_ex__', '__repr__', '__rfloordiv__', '__rlshift__', '__rmod__', '__rmul__', '__ror__', '__round__', '__rpow__', '__rrshift__', '__rshift__', '__rsub__', '__rtruediv__', '__rxor__', '__setattr__', '__sizeof__', '__str__', '__sub__', '__subclasshook__', '__truediv__', '__trunc__', '__xor__', 'as_integer_ratio', 'bit_length', 'conjugate', 'denominator', 'from_bytes', 'imag', 'numerator', 'real', 'to_bytes']
['__abs__', '__add__', '__bool__', '__class__', '__delattr__', '__dir__', '__divmod__', '__doc__', '__eq__', '__float__', '__floordiv__', '__format__', '__ge__', '__getattribute__', '__getformat__', '__getnewargs__', '__gt__', '__hash__', '__init__', '__init_subclass__', '__int__', '__le__', '__lt__', '__mod__', '__mul__', '__ne__', '__neg__', '__new__', '__pos__', '__pow__', '__radd__', '__rdivmod__', '__reduce__', '__reduce_ex__', '__repr__', '__rfloordiv__', '__rmod__', '__rmul__', '__round__', '__rpow__', '__rsub__', '__rtruediv__', '__set_format__', '__setattr__', '__sizeof__', '__str__', '__sub__', '__subclasshook__', '__truediv__', '__trunc__', 'as_integer_ratio', 'conjugate', 'fromhex', 'hex', 'imag', 'is_integer', 'real']
['__class__', '__delattr__', '__dict__', '__dir__', '__doc__', '__eq__', '__format__', '__ge__', '__getattribute__', '__gt__', '__hash__', '__init__', '__init_subclass__', '__le__', '__lt__', '__module__', '__ne__', '__new__', '__reduce__', '__reduce_ex__', '__repr__', '__setattr__', '__sizeof__', '__str__', '__subclasshook__', '__weakref__', 'a', 'b', 'c', 'get_current_x', 'set_x']
dir()
메서드를 사용하여 모든 모듈 메서드를 나열할 수 있습니다. 방법을 보려면 다음 코드를 참조하십시오.
import numpy
print("NumPy Module")
print(dir(numpy))
출력:
보시다시피 dir()
메소드는 모든 NumPy
메소드를 나열합니다.
inspect()
모듈을 사용하여 Python 모듈의 모든 메서드 나열
inspect
모듈은 모듈, 클래스, 클래스 개체, 함수, 역추적 및 개체에 대한 유용한 정보를 얻을 수 있는 몇 가지 유용한 기능이 있는 Python 모듈입니다. 간단한 목적으로 이 모듈을 사용할 수 있습니다. 사용법에 대해 알아보려면 다음 코드를 참조하세요.
import numpy
from inspect import getmembers, isfunction
print("NumPy Module")
print(getmembers(numpy, isfunction))
출력:
getmembers()
함수는 이름에서 알 수 있듯이 클래스 또는 모듈 내부에 있는 메서드, 변수, 클래스 등과 같은 모든 멤버를 가져옵니다. 메서드나 함수만 필요하기 때문에 멤버에서 모든 함수를 걸러내는 Python 함수인 isfunction()
을 사용했습니다. 아래와 같은 다른 기능도 있습니다.
ismodule()
: 객체가 모듈이면 True를 반환합니다.isclass()
: 객체가 클래스이면 True를 반환합니다.istraceback()
: 객체가 트레이스백이면 True를 반환합니다.isgenerator()
: 객체가 제너레이터이면 True를 반환합니다.iscode()
: 객체가 코드이면 True를 반환합니다.isframe()
: 객체가 프레임이면 True를 반환합니다.isabstract()
: 객체가 추상 기본 클래스이면 True를 반환합니다.iscoroutine()
: 객체가 코루틴이면 True를 반환합니다.
이 모듈에 대한 자세한 내용은 공식 문서를 참조하세요.