Python 파일에서 문자열 찾기
-
File
readlines()
메서드를 사용하여 Python의 파일에서 문자열 찾기 -
File
read()
메서드를 사용하여 Python에서 파일의 문자열 검색 -
find
메서드를 사용하여 Python에서 파일의 문자열 검색 -
mmap
모듈을 사용하여 Python에서 파일의 문자열 검색
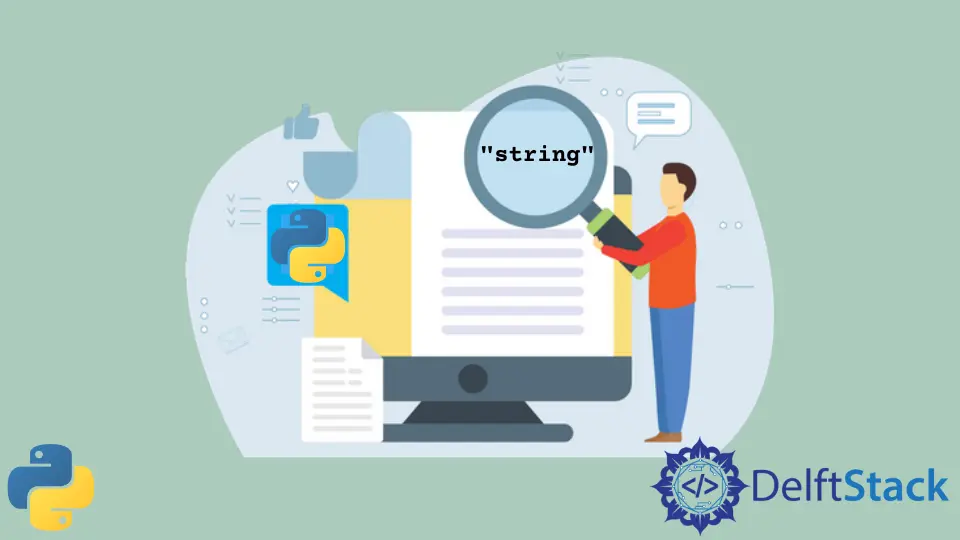
이 자습서에서는 Python의 텍스트 파일에서 특정 문자열을 찾는 방법을 설명합니다.
File readlines()
메서드를 사용하여 Python의 파일에서 문자열 찾기
Pyton 파일readlines()
메서드는 새 줄에 의해 목록으로 분할 된 파일 내용을 반환합니다. for
루프를 사용하여 목록을 반복하고 in
연산자를 사용하여 문자열이 모든 반복에서 줄에 있는지 확인할 수 있습니다.
줄에서 문자열이 발견되면 True
를 반환하고 루프를 중단합니다. 모든 행을 반복 한 후에도 문자열이 발견되지 않으면 결국 False
를 반환합니다.
이 접근 방식에 대한 예제 코드는 다음과 같습니다.
file = open("temp.txt", "w")
file.write("blabla is nothing.")
file.close()
def check_string():
with open("temp.txt") as temp_f:
datafile = temp_f.readlines()
for line in datafile:
if "blabla" in line:
return True # The string is found
return False # The string does not exist in the file
if check_string():
print("True")
else:
print("False")
출력:
True
File read()
메서드를 사용하여 Python에서 파일의 문자열 검색
파일read()
메서드는 파일의 내용을 전체 문자열로 반환합니다. 그런 다음in
연산자를 사용하여 문자열이 반환 된 문자열에 있는지 확인할 수 있습니다.
예제 코드는 다음과 같습니다.
file = open("temp.txt", "w")
file.write("blabla is nothing.")
file.close()
with open("temp.txt") as f:
if "blabla" in f.read():
print("True")
출력:
True
find
메서드를 사용하여 Python에서 파일의 문자열 검색
간단한find
메서드를read()
메서드와 함께 사용하여 파일에서 문자열을 찾을 수 있습니다. find
메소드에 필수 문자열이 전달됩니다. 문자열을 찾으면 0
을 반환하고 문자열이 없으면 -1
을 반환합니다.
예제 코드는 다음과 같습니다.
file = open("temp.txt", "w")
file.write("blabla is nothing.")
file.close()
print(open("temp.txt", "r").read().find("blablAa"))
출력:
-1
mmap
모듈을 사용하여 Python에서 파일의 문자열 검색
또한 mmap
모듈을 사용하여 Python의 파일에서 문자열을 찾을 수 있으며 파일 크기가 상대적으로 크면 성능을 향상시킬 수 있습니다. mmap.mmap()
메서드는 암시 적 파일 만 확인하고 전체 파일을 읽지 않는 Python 2에서 문자열과 유사한 객체를 만듭니다.
Python 2의 예제 코드는 다음과 같습니다.
# python 2
import mmap
file = open("temp.txt", "w")
file.write("blabla is nothing.")
file.close()
with open("temp.txt") as f:
s = mmap.mmap(f.fileno(), 0, access=mmap.ACCESS_READ)
if s.find("blabla") != -1:
print("True")
출력:
True
그러나 Python3 이상에서mmap
은 문자열과 유사한 객체처럼 동작하지 않고bytearray
객체를 생성합니다. 따라서find
메소드는 문자열이 아닌 바이트를 찾습니다.
이에 대한 예제 코드는 다음과 같습니다.
import mmap
file = open("temp.txt", "w")
file.write("blabla is nothing.")
file.close()
with open("temp.txt") as f:
s = mmap.mmap(f.fileno(), 0, access=mmap.ACCESS_READ)
if s.find(b"blabla") != -1:
print("True")
출력:
True
Syed Moiz is an experienced and versatile technical content creator. He is a computer scientist by profession. Having a sound grip on technical areas of programming languages, he is actively contributing to solving programming problems and training fledglings.
LinkedIn관련 문장 - Python String
- Python의 문자열에서 쉼표 제거
- 파이썬 방식으로 문자열이 비어 있는지 확인하는 방법
- Python에서 문자열을 변수 이름으로 변환
- 파이썬에서 문자열에서 공백을 제거하는 방법
- Python의 문자열에서 숫자 추출
- 파이썬에서 문자열을 날짜 / 시간으로 변환하는 방법