Python에서 날짜 시간 빼기
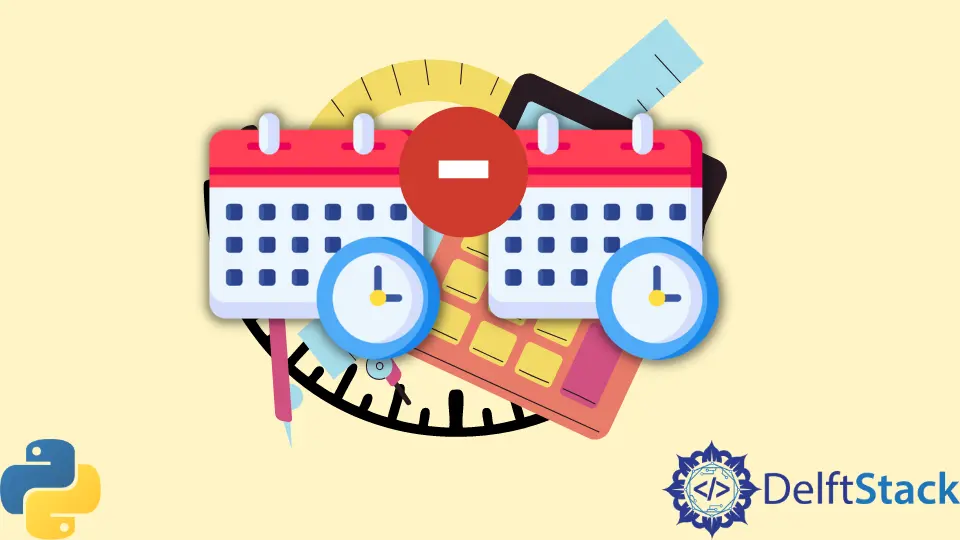
이 튜토리얼은 파이썬에서 날짜 시간 빼기를 수행하는 방법을 소개합니다.
제공된 두 datetime 객체의 차이에 대한 초, 분, 시간 또는 연도를 출력하는 것과 같이 빼기 후 다른 출력을 탐색합니다.
datetime
모듈을 사용하여 Python에서 날짜 시간 빼기
datetime
은datetime
객체를 조작하는 함수를 지원하는 Python의 모듈입니다.
datetime
객체를 초기화하려면datetime.datetime(year, month, day)
과 같은 3 개의 필수 매개 변수가 필요합니다. 또한 시간, 분, 초, 마이크로 초 및 시간대 형식의 선택적 매개 변수를 허용합니다.
datetime
에는 현재 날짜와 시간을 객체로 초기화하는now()
함수도 있습니다.
두 개의 datetime 객체를 빼면 일 수의 차이와 시간 차이 (있는 경우)가 반환됩니다.
from datetime import datetime
then = datetime(1987, 12, 30, 17, 50, 14) # yr, mo, day, hr, min, sec
now = datetime(2020, 12, 25, 23, 13, 0)
print(now - then)
출력:
12055 days, 5:10:00
결과는 두 날짜가 12055 일과 5 시간 10 분 간격이라는 것을 의미합니다.
이 결과를 다른 형식으로 변환하려면 먼저 timedelta
를 초로 변환해야합니다. datetime 객체에는 객체를 포함 된 총 초로 변환하는 내장 메소드datetime.total_seconds()
가 있습니다.
다음은 datetime
모듈과 그 기능을 사용한 날짜 시간 빼기의 전체 소스 코드입니다.
from datetime import datetime
def getDifference(then, now=datetime.now(), interval="secs"):
duration = now - then
duration_in_s = duration.total_seconds()
# Date and Time constants
yr_ct = 365 * 24 * 60 * 60 # 31536000
day_ct = 24 * 60 * 60 # 86400
hour_ct = 60 * 60 # 3600
minute_ct = 60
def yrs():
return divmod(duration_in_s, yr_ct)[0]
def days():
return divmod(duration_in_s, day_ct)[0]
def hrs():
return divmod(duration_in_s, hour_ct)[0]
def mins():
return divmod(duration_in_s, minute_ct)[0]
def secs():
return duration_in_s
return {
"yrs": int(yrs()),
"days": int(days()),
"hrs": int(hrs()),
"mins": int(mins()),
"secs": int(secs()),
}[interval]
then = datetime(1987, 12, 30, 17, 50, 14) # yr, mo, day, hr, min, sec
now = datetime(2020, 12, 25, 23, 13, 0)
print("The difference in seconds:", getDifference(then, now, "secs"))
print("The difference in minutes:", getDifference(then, now, "mins"))
print("The difference in hours:", getDifference(then, now, "hrs"))
print("The difference in days:", getDifference(then, now, "days"))
print("The difference in years:", getDifference(then, now, "yrs"))
코드가 수행하는 첫 번째 작업은 뺄셈을 위해 2 개의 개별 날짜를 초기화하고 매번 다른 출력으로 함수를 여러 번 호출하는 것입니다. all
의 경우 몇 년에서 몇 초까지 모든 경우를 기준으로 차이를 가져 오는 출력을 반환합니다.
divmod()
함수는 두 숫자를 피제수와 제수로 받아들이고 몫의 튜플과 그 사이의 나머지를 반환합니다. 몫만 필요하므로 코드를 통해 0
인덱스 만 사용됩니다.
출력:
The difference in seconds: 1041052966
The difference in minutes: 17350882
The difference in hours: 289181
The difference in days: 12049
The difference in years: 33
이제 여러 다른 시간 프레임 내에서 두 개의 datetime 객체간에 차이가 있습니다.
Skilled in Python, Java, Spring Boot, AngularJS, and Agile Methodologies. Strong engineering professional with a passion for development and always seeking opportunities for personal and career growth. A Technical Writer writing about comprehensive how-to articles, environment set-ups, and technical walkthroughs. Specializes in writing Python, Java, Spring, and SQL articles.
LinkedIn