Python에서 목록의 모든 순열을 생성하는 방법
Hassan Saeed
2023년1월30일
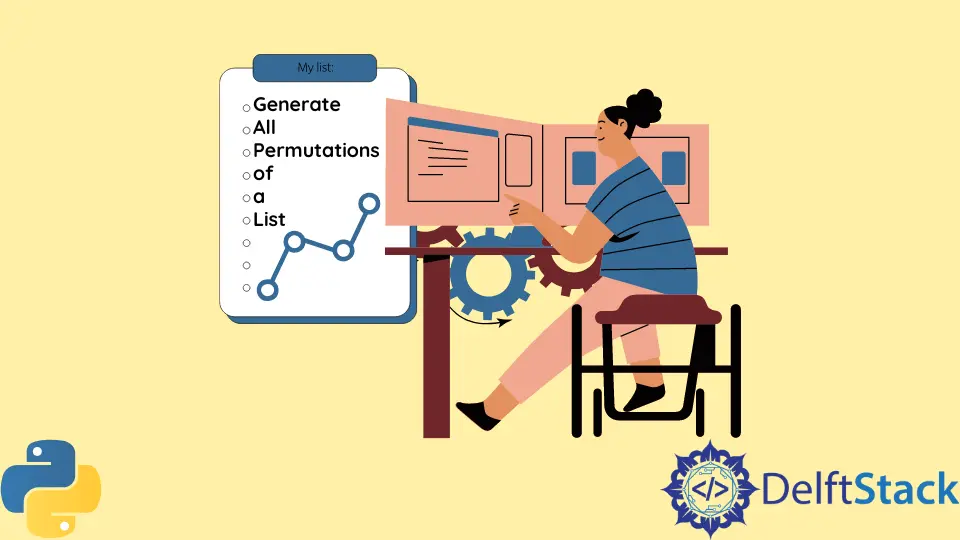
이 자습서에서는 Python에서 목록의 모든 순열을 생성하는 방법에 대해 설명합니다.
itertools.permutations
를 사용하여 Python에서 목록의 모든 순열 생성
Python은 순열을 생성하는 표준 라이브러리 도구를 제공합니다. itertools.permutation
. 아래 예제는 이것을 사용하여 목록의 모든 순열을 생성하는 방법을 보여줍니다.
import itertools
inp_list = [4, 5, 6]
permutations = list(itertools.permutations(inp_list))
print(permutations)
출력:
[(4, 5, 6), (4, 6, 5), (5, 4, 6), (5, 6, 4), (6, 4, 5), (6, 5, 4)]
순열의 기본 길이는 입력 목록의 길이로 설정됩니다. 그러나 itertools.permutations
함수 호출에서 순열의 길이를 지정할 수 있습니다. 아래 예는이를 설명합니다.
import itertools
inp_list = [1, 2, 3]
permutations = list(itertools.permutations(inp_list, r=2))
print(permutations)
출력:
[(4, 5), (4, 6), (5, 4), (5, 6), (6, 4), (6, 5)]
아래 예는 주어진 목록에서 가능한 모든 길이의 모든 순열을 생성하는 방법을 보여줍니다.
import itertools
inp_list = [1, 2, 3]
permutations = []
for i in range(1, len(inp_list) + 1):
permutations.extend(list(itertools.permutations(inp_list, r=i)))
print(permutations)
출력:
[(4,), (5,), (6,), (4, 5), (4, 6), (5, 4), (5, 6), (6, 4), (6, 5), (4, 5, 6), (4, 6, 5), (5, 4, 6), (5, 6, 4), (6, 4, 5), (6, 5, 4)]
재귀를 사용하여 Python에서 목록의 모든 순열 생성
또한 재귀를 사용하여 Python에서 목록의 모든 순열을 생성 할 수 있습니다. 아래 예는이를 설명합니다.
def permutations(start, end=[]):
if len(start) == 0:
print(end)
else:
for i in range(len(start)):
permutations(start[:i] + start[i + 1 :], end + start[i : i + 1])
permutations([4, 5, 6])
출력:
[4, 5, 6]
[4, 6, 5]
[5, 4, 6]
[5, 6, 4]
[6, 4, 5]
[6, 5, 4]