Pandas를 사용하여 DataFrame에서 중복 행 찾기
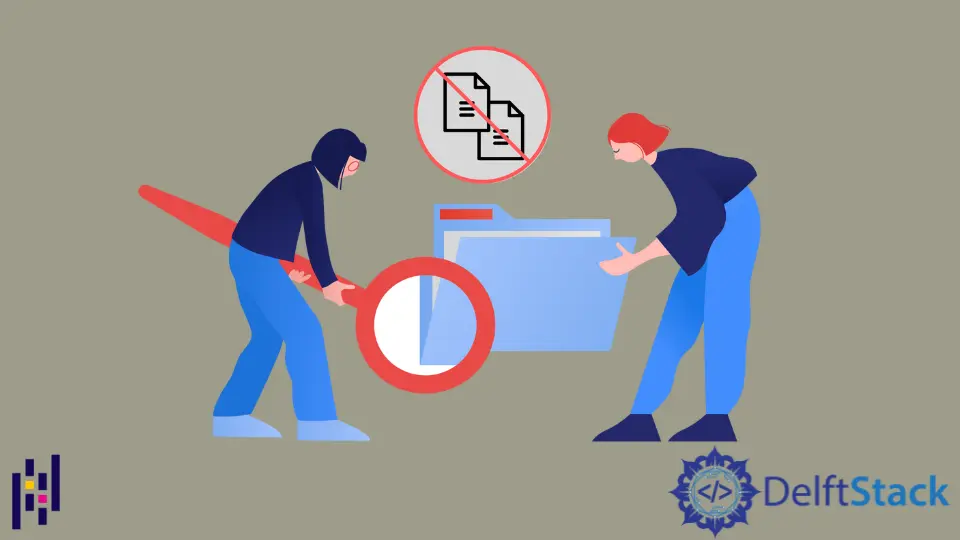
정리 절차의 일부로 데이터 세트에서 중복 값을 식별해야 합니다. 중복 데이터는 불필요한 저장 공간을 소비하고 최소한 계산 속도를 늦춥니다. 그러나 최악의 시나리오에서 중복 데이터는 분석 결과를 왜곡하고 데이터 세트의 무결성을 손상시킬 수 있습니다.
Pandas라는 오픈 소스 Python 패키지는 구조화된 데이터의 처리 및 저장을 향상시킵니다. 또한 프레임워크는 중복 행 및 열 찾기 및 삭제와 같은 데이터 정리 절차에 대한 기본 제공 지원을 제공합니다.
이 문서에서는 열 전체 또는 일부 열을 사용하여 Pandas 데이터 프레임에서 중복 항목을 찾는 방법을 설명합니다. 이를 위해 Pandas의 Dataframe.duplicated()
메서드를 사용합니다.
DataFrame.duplicated()
메서드를 사용하여 DataFrame에서 중복 행 찾기
Python의 DataFrame
클래스용 Pandas 라이브러리는 다음과 같이 모든 열 또는 해당 열의 하위 집합을 기반으로 중복 행을 검색하는 멤버 메서드를 제공합니다.
DataFrame.duplicated(subset=None, keep="first")
행이 중복인지 고유한지 여부를 나타내는 일련의 부울을 반환합니다.
매개변수:
하위 집합
: 여기에는 열 또는 열 레이블 모음이 필요합니다. 없음이 기본값입니다. 열을 전달한 후에는 중복 항목만 고려합니다.keep
: 중복 값 처리를 규제합니다.first
가 기본값인 세 가지 값만 있습니다.첫 번째
인 경우 첫 번째 항목은 고유한 항목으로 처리되고 나머지 값은 중복 항목으로 처리됩니다.최신
인 경우 최종 항목은 고유한 항목으로 처리되고 나머지 값은 중복 항목으로 처리됩니다.False
인 경우 모든 동일한 값은 중복으로 간주됩니다.- 부울 계열로 표시된 중복 행을 반환합니다.
중복 행이 있는 DataFrame 만들기
목록 모음으로 기본 Dataframe을 만들고 Name
, Age
및 City
열의 이름을 지정해 보겠습니다.
예제 코드:
# Import pandas library
import pandas as pd
# List of Tuples
employees = [
("Joe", 28, "Chicago"),
("John", 32, "Austin"),
("Melvin", 25, "Dallas"),
("John", 32, "Austin"),
("John", 32, "Austin"),
("John", 32, "Houston"),
("Melvin", 40, "Dehradun"),
("Hazel", 32, "Austin"),
]
df = pd.DataFrame(employees, columns=["Name", "Age", "City"])
print(df)
출력:
Name Age City
0 Joe 28 Chicago
1 John 32 Austin
2 Melvin 25 Dallas
3 John 32 Austin
4 John 32 Austin
5 John 32 Houston
6 Melvin 40 Dehradun
7 Hazel 32 Austin
모든 열을 기준으로 중복 행 선택
subset
매개변수 없이 Dataframe.duplicate()
를 호출하여 모든 열에 따라 모든 행에 대한 중복 항목을 찾아 선택합니다. 그러나 중복 행이 있는 경우 첫 번째 인스턴스 위치에서 True
가 포함된 부울 시리즈만 반환합니다(유지 인수의 기본값은 first
임).
그런 다음 이 부울 시리즈를 DataFrame의 []
연산자에 제공하여 중복 행을 선택합니다.
예제 코드:
# Import pandas library
import pandas as pd
# List of Tuples
employees = [
("Joe", 28, "Chicago"),
("John", 32, "Austin"),
("Melvin", 25, "Dallas"),
("John", 32, "Austin"),
("John", 32, "Austin"),
("John", 32, "Houston"),
("Melvin", 40, "Dehradun"),
("Hazel", 32, "Austin"),
]
df = pd.DataFrame(employees, columns=["Name", "Age", "City"])
duplicate = df[df.duplicated()]
print("Duplicate Rows :")
print(duplicate)
출력:
Duplicate Rows :
Name Age City
3 John 32 Austin
4 John 32 Austin
마지막 항목을 제외한 모든 중복 항목을 고려하려면 retain = "last"
를 인수로 전달하십시오.
예제 코드:
# Import pandas library
import pandas as pd
# List of Tuples
employees = [
("Joe", 28, "Chicago"),
("John", 32, "Austin"),
("Melvin", 25, "Dallas"),
("John", 32, "Austin"),
("John", 32, "Austin"),
("John", 32, "Houston"),
("Melvin", 40, "Dehradun"),
("Hazel", 32, "Austin"),
]
df = pd.DataFrame(employees, columns=["Name", "Age", "City"])
duplicate = df[df.duplicated(keep="last")]
print("Duplicate Rows :")
print(duplicate)
출력:
Duplicate Rows :
Name Age City
1 John 32 Austin
3 John 32 Austin
그런 다음 지정된 몇 개의 열에 따라 중복 행만 선택하려는 경우 subset
의 열 이름 목록을 매개변수로 제공하십시오.
예제 코드:
# Import pandas library
import pandas as pd
# List of Tuples
employees = [
("Joe", 28, "Chicago"),
("John", 32, "Austin"),
("Melvin", 25, "Dallas"),
("John", 32, "Austin"),
("John", 32, "Austin"),
("John", 32, "Houston"),
("Melvin", 40, "Dehradun"),
("Hazel", 32, "Austin"),
]
df = pd.DataFrame(employees, columns=["Name", "Age", "City"])
# on 'City' column
duplicate = df[df.duplicated("City")]
print("Duplicate Rows based on City:")
print(duplicate)
출력:
Duplicate Rows based on City:
Name Age City
3 John 32 Austin
4 John 32 Austin
7 Hazel 32 Austin
Name
및 Age
와 같은 둘 이상의 열 이름을 기준으로 중복 행을 선택합니다.
예제 코드:
# Import pandas library
import pandas as pd
# List of Tuples
employees = [
("Joe", 28, "Chicago"),
("John", 32, "Austin"),
("Melvin", 25, "Dallas"),
("John", 32, "Austin"),
("John", 32, "Austin"),
("John", 32, "Houston"),
("Melvin", 40, "Dehradun"),
("Hazel", 32, "Austin"),
]
df = pd.DataFrame(employees, columns=["Name", "Age", "City"])
# list of the column names
duplicate = df[df.duplicated(["Name", "Age"])]
print("Duplicate the rows based on Name and Age:")
print(duplicate)
출력:
Duplicate Rows based on Name and Age:
Name Age City
3 John 32 Austin
4 John 32 Austin
5 John 32 Houston
결론
DataFrame에서 중복 행을 찾으려면 Pandas에서 dataframe.duplicated()
메서드를 사용하세요. 행이 중복인지 고유한지 여부를 나타내는 일련의 부울을 반환합니다.
이 기사가 여기에서 논의한 모든 예를 확인하여 열의 전체 또는 하위 집합을 사용하여 Dataframe에서 중복 행을 찾는 데 도움이 되었기를 바랍니다. 그런 다음 위에서 설명한 쉬운 단계를 사용하여 Pandas를 사용하여 중복 항목을 찾는 방법을 신속하게 결정할 수 있습니다.
Zeeshan is a detail oriented software engineer that helps companies and individuals make their lives and easier with software solutions.
LinkedIn