Pandas의 Datetime 열에서 월과 연도를 별도로 추출하는 방법
-
월 및 연도를 추출하는
pandas.Series.dt.year()
및pandas.Series.dt.month()
메소드 -
연도 및 월을 추출하는
strftime()
메소드 -
연도 및 월을 추출하는
pandas.DatetimeIndex.month
및pandas.DatetimeIndex.year
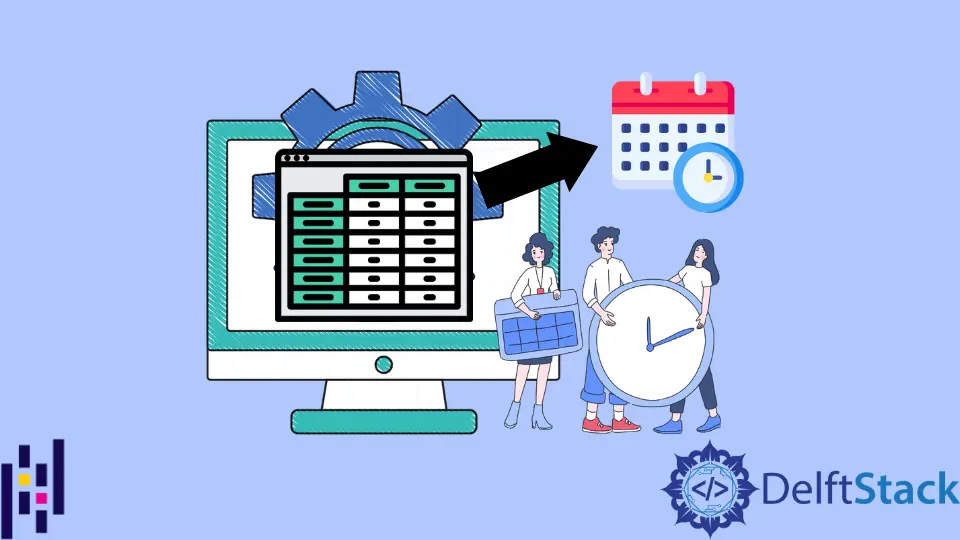
pandas.Series.dt.year()
및pandas.Series.dt.month()
메소드를 각각 사용하여Datetime
열에서 연도와 월을 추출 할 수 있습니다. 데이터가Datetime
유형이 아닌 경우 먼저 데이터를Datetime
으로 변환해야합니다. pandas.DatetimeIndex.month
와pandas.DatetimeIndex.year
및strftime()
메소드를 사용하여 년과 월을 추출 할 수도 있습니다.
월 및 연도를 추출하는 pandas.Series.dt.year()
및 pandas.Series.dt.month()
메소드
Datetime
유형에 적용된pandas.Series.dt.year()
및pandas.Series.dt.month()
메소드는 시리즈 오브젝트의Datetime
항목에 대해 NumPy
연도 및 월의 배열을 리턴합니다.
import pandas as pd
import numpy as np
import datetime
list_of_dates = ["2019-11-20", "2020-01-02", "2020-02-05", "2020-03-10", "2020-04-16"]
employees = ["Hisila", "Shristi", "Zeppy", "Alina", "Jerry"]
df = pd.DataFrame({"Joined date": pd.to_datetime(list_of_dates)}, index=employees)
df["Year"] = df["Joined date"].dt.year
df["Month"] = df["Joined date"].dt.month
print(df)
출력:
Joined date Year Month
Hisila 2019-11-20 2019 11
Shristi 2020-01-02 2020 1
Zeppy 2020-02-05 2020 2
Alina 2020-03-10 2020 3
Jerry 2020-04-16 2020 4
그러나 열이Datetime
유형이 아닌 경우에는 먼저 to_datetime()
메소드를 사용하여 열을Datetime
유형으로 변환해야합니다.
import pandas as pd
import numpy as np
import datetime
list_of_dates = ["11/20/2019", "01/02/2020", "02/05/2020", "03/10/2020", "04/16/2020"]
employees = ["Hisila", "Shristi", "Zeppy", "Alina", "Jerry"]
df = pd.DataFrame({"Joined date": pd.to_datetime(list_of_dates)}, index=employees)
df["Joined date"] = pd.to_datetime(df["Joined date"])
df["Year"] = df["Joined date"].dt.year
df["Month"] = df["Joined date"].dt.month
print(df)
출력:
Joined date Year Month
Hisila 2019-11-20 2019 11
Shristi 2020-01-02 2020 1
Zeppy 2020-02-05 2020 2
Alina 2020-03-10 2020 3
Jerry 2020-04-16 2020 4
연도 및 월을 추출하는strftime()
메소드
strftime()
메소드는 Datetime을 형식 코드로 입력하고 출력에 지정된 특정 형식을 나타내는 문자열을 리턴합니다. 우리는%Y
와%m
을 형식 코드로 사용하여 년과 월을 추출합니다.
import pandas as pd
import numpy as np
import datetime
list_of_dates = ["2019-11-20", "2020-01-02", "2020-02-05", "2020-03-10", "2020-04-16"]
employees = ["Hisila", "Shristi", "Zeppy", "Alina", "Jerry"]
df = pd.DataFrame({"Joined date": pd.to_datetime(list_of_dates)}, index=employees)
df["year"] = df["Joined date"].dt.strftime("%Y")
df["month"] = df["Joined date"].dt.strftime("%m")
print(df)
출력:
Joined date year month
Hisila 2019-11-20 2019 11
Shristi 2020-01-02 2020 01
Zeppy 2020-02-05 2020 02
Alina 2020-03-10 2020 03
Jerry 2020-04-16 2020 04
연도 및 월을 추출하는pandas.DatetimeIndex.month
및pandas.DatetimeIndex.year
Datetime
열에서 월과 연도를 추출하는 또 다른 간단한 방법은 pandas.DatetimeIndex
클래스의 객체의 연도 및 월 속성 값을 검색하는 것입니다.
import pandas as pd
import numpy as np
import datetime
list_of_dates = ["2019-11-20", "2020-01-02", "2020-02-05", "2020-03-10", "2020-04-16"]
employees = ["Hisila", "Shristi", "Zeppy", "Alina", "Jerry"]
df = pd.DataFrame({"Joined date": pd.to_datetime(list_of_dates)}, index=employees)
df["year"] = pd.DatetimeIndex(df["Joined date"]).year
df["month"] = pd.DatetimeIndex(df["Joined date"]).month
print(df)
출력:
Joined date Year Month
Hisila 2019-11-20 2019 11
Shristi 2020-01-02 2020 1
Zeppy 2020-02-05 2020 2
Alina 2020-03-10 2020 3
Jerry 2020-04-16 2020 4
pandas.DatetimeIndex
클래스는 datetime64 데이터의 불변의 ndarray입니다. year
,month
,day
등과 같은 속성이 있습니다.
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn