Pandas DataFrame 에서 부동 소수점을 정수로 변환하는 방법
Asad Riaz
2023년1월30일
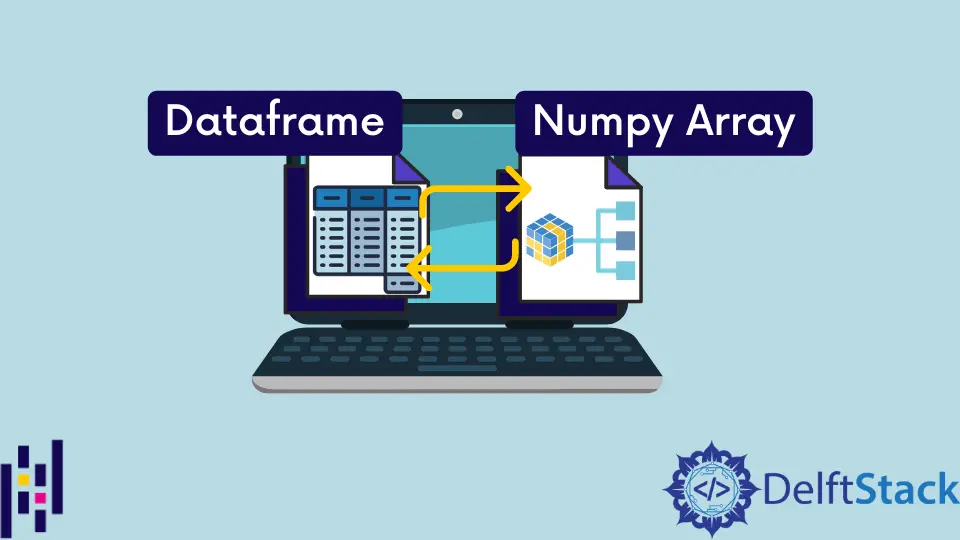
Pandas DataFrame.astype(int)
및 to_numeric()
메소드에서 float 를 정수로 변환하는 메소드를 시연합니다.
먼저,NumPy
라이브러리를 사용하여 무작위 배열을 만든 다음이를 DataFrame
으로 변환합니다.
이 코드를 실행하면 float
에 값이있는 다음과 같은 출력이 나타납니다.
012 34
00.3024483.5519583.8786602.3803524.741592
14.0541870.9409520.4590584.3148010.524993
# python 3.x
import pandas as pd
import numpy as np
df = pd.DataFrame(data=np.random.randint(0, 10, (6, 4)), columns=["a", "b", "c", "d"])
nmp = df.to_numpy()
print(nmp)
print(type(nmp))
22.8917334.9268854.9557732.6263734.144166
[[5 5 1 3]
[1 6 6 0]
[9 1 2 0]
[9 3 5 3]
[7 9 4 9]
[8 1 8 9]]
<class 'numpy.ndarray'>
31.1276393.1968234.1440201.3506320.401138
# python 3.x
import pandas as pd
import numpy as np
df = pd.DataFrame(data=np.random.randint(0, 10, (6, 4)), columns=["a", "b", "c", "d"])
nmp = df.values
print(nmp)
print(type(nmp))
41.4235372.0194553.0389450.4366573.823888
[[8 8 5 0]
[1 7 7 5]
[0 2 4 2]
[6 8 0 7]
[6 4 5 1]
[1 8 4 7]]
<class 'numpy.ndarray'>
astype(int)
-Pandas 에서 float
를 int
로 변환
# python 3.x
import pandas as pd
import numpy as np
df = pd.DataFrame(data=np.random.randint(0, 10, (6, 4)), columns=["a", "b", "c", "d"])
nmp = df.reset_index().values
print(nmp)
print(type(nmp))
float
를 INT
로 변환하기 위해 Pandas
패키지에서 제공하는 astype(int)
메소드를 사용합니다. 코드는
[[0 1 0 3 7]
[1 8 2 5 1]
[2 2 2 7 3]
[3 3 4 3 7]
[4 5 4 4 3]
[5 2 9 7 6]]
<class 'numpy.ndarray'>
위의 코드를 실행하면 다음과 같은 결과가 나타납니다
*********** 랜덤 플로트 데이터 프레임 ************
# python 3.x
import pandas as pd
import numpy as np
df = pd.DataFrame(data=np.random.randint(0, 10, (6, 4)), columns=["a", "b", "c", "d"])
nmp = df.to_records()
print(nmp)
print(type(nmp))
012 34
[(0, 0, 4, 6, 1)
(1, 3, 1, 7, 1)
(2, 9, 1, 6, 4)
(3, 1, 4, 6, 9)
(4, 9, 1, 3, 9)
(5, 2, 5, 7, 9)]
<class 'numpy.recarray'>