Pandas에서 열의 데이터 유형을 변경하는 방법
Asad Riaz
2023년1월30일
Pandas
Pandas Data Type
-
Pandas에서 열을 숫자 값으로 변환하는
to_numeric
메소드 -
한 유형을 다른 데이터 유형으로 변환하는
astype()
메소드 -
infer_objects()
메소드를 사용하여 열 데이터 유형을보다 구체적인 유형으로 변환
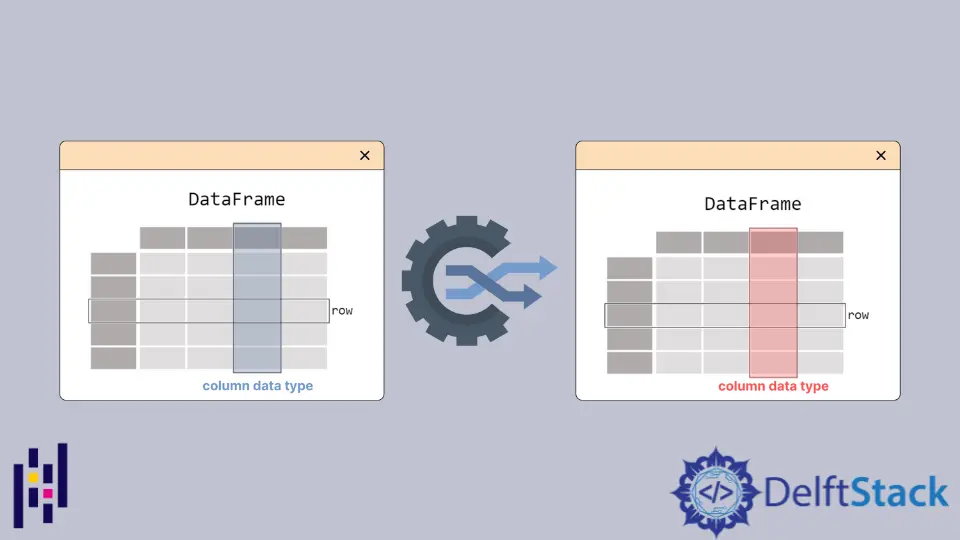
Pandas DataFrame
에서 열의 데이터 유형을 변경하는 방법과to_numaric
,as_type
및infer_objects
와 같은 옵션을 소개합니다. 또한to_numaric
과 함께downcasting
옵션을 사용하는 방법에 대해서도 논의 할 것입니다.
Pandas에서 열을 숫자 값으로 변환하는to_numeric
메소드
to_numeric()
은DataFrame
의 하나 이상의 열을 숫자 값으로 변환하는 가장 좋은 방법입니다. 또한 숫자가 아닌 객체 (예 : 문자열)를 정수 또는 부동 소수점 숫자로 적절하게 변경하려고합니다. 일부 값을 숫자 형으로 변환 할 수없는 경우,to_numeric()
을 사용하면 숫자가 아닌 값을 NaN
으로 만들 수 있습니다.
예제 코드:
# python 3.x
import pandas as pd
s = pd.Series(["12", "12", "4.7", "asad", "3.0"])
print(s)
print("------------------------------")
print(pd.to_numeric(s, errors="coerce"))
출력:
0 12
1 12
2 4.7
3 asad
4 3.0
dtype: object
------------------------------
0 12.0
1 12.0
2 4.7
3 NaN
4 3.0
dtype: float64
to_numeric()
은 기본적으로int64
또는float64
dtype을 제공합니다. 옵션을 사용하여integer
,signed
,unsigned
또는float
로 캐스트 할 수 있습니다:
# python 3.x
import pandas as pd
s = pd.Series([-3, 1, -5])
print(s)
print(pd.to_numeric(s, downcast="integer"))
출력:
0 -3
1 1
2 -5
dtype: int64
0 -3
1 1
2 -5
dtype: int8
한 유형을 다른 데이터 유형으로 변환하는astype()
메소드
astype()
메소드를 사용하면 변환하려는 dtype에 대해 명시 적으로 지정할 수 있습니다. astype()
메소드 내부에 매개 변수를 전달하여 한 데이터 유형을 다른 데이터 유형으로 변환 할 수 있습니다.
예제 코드:
# python 3.x
import pandas as pd
c = [["x", "1.23", "14.2"], ["y", "20", "0.11"], ["z", "3", "10"]]
df = pd.DataFrame(c, columns=["first", "second", "third"])
print(df)
df[["second", "third"]] = df[["second", "third"]].astype(float)
print("Converting..................")
print("............................")
print(df)
출력:
first second third
0 x 1.23 14.2
1 y 20 0.11
2 z 3 10
Converting..................
............................
first second third
0 x 1.23 14.20
1 y 20.00 0.11
2 z 3.00 10.00
infer_objects()
메소드를 사용하여 열 데이터 유형을보다 구체적인 유형으로 변환
infer_objects()
메소드는dataFrame
의 열을보다 구체적인 데이터 유형 (소프트 변환)으로 변환하기 위해 Pandas 버전 0.21.0에서 도입되었습니다.
예제 코드:
# python 3.x
import pandas as pd
df = pd.DataFrame({"a": [3, 12, 5], "b": [3.0, 2.6, 1.1]}, dtype="object")
print(df.dtypes)
df = df.infer_objects()
print("Infering..................")
print("............................")
print(df.dtypes)
출력:
a object
b object
dtype: object
Infering..................
............................
a int64
b float64
dtype: object
튜토리얼이 마음에 드시나요? DelftStack을 구독하세요 YouTube에서 저희가 더 많은 고품질 비디오 가이드를 제작할 수 있도록 지원해주세요. 구독하다