Pandas DataFrame에 한 행을 추가하는 방법
Asad Riaz
2023년1월30일
Pandas
Pandas DataFrame
-
목록이있는 Pandas 데이터 프레임에 행을 추가하는
.loc[index]
메소드 - 사전을 행으로 추가하여 Pandas 데이터 프레임에 추가하십시오
-
행을 추가하는 데이터 프레임
append()
메소드
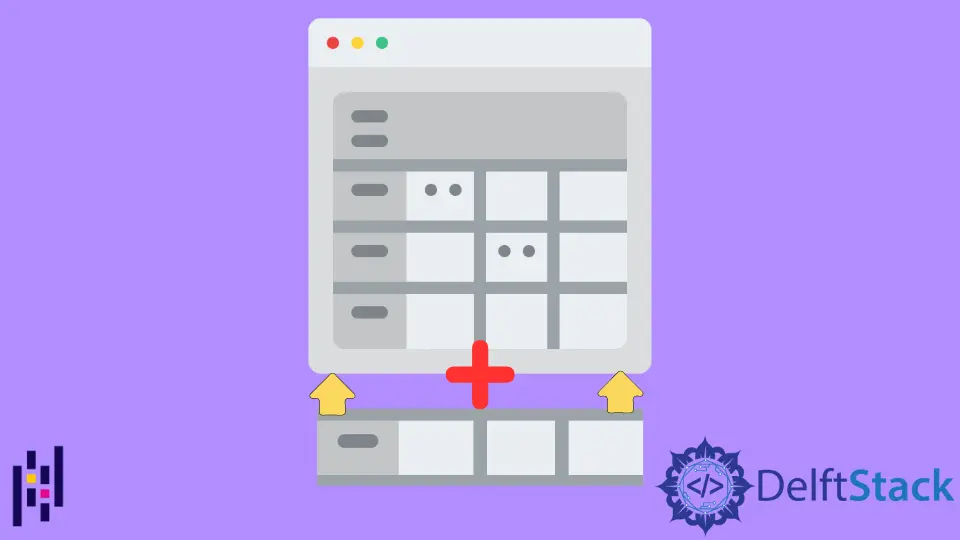
Pandas는 완전히 채워진 ‘데이터 프레임’을로드하도록 설계되었습니다. .loc
,dictionaries
,pandas.concat()
또는DataFrame.append()
와 같은 다양한 접근 방식을 사용하여pandas.Dataframe
에 행을 하나씩 추가 할 수 있습니다.
목록이있는 Pandas 데이터 프레임에 행을 추가하는.loc[index]
메소드
loc[index]
는 새로운리스트를 새로운 행으로 가져 와서 주어진pandas.Dataframe
의index
에 추가합니다.
예제 코드:
# python 3.x
import pandas as pd
# List of Tuples
fruit_list = [("Orange", 34, "Yes")]
# Create a DataFrame object
df = pd.DataFrame(fruit_list, columns=["Name", "Price", "Stock"])
# Add new ROW
df.loc[1] = ["Mango", 4, "No"]
df.loc[2] = ["Apple", 14, "Yes"]
print(df)
출력:
Name Price Stock
0 Orange 34 Yes
1 Mango 4 No
2 Apple 14 Yes
경고
ignore_index
는 사전을append()
함수에 전달할 때 True
으로 설정됩니다. 그렇지 않으면 오류가 발생합니다.사전을 행으로 추가하여 Pandas 데이터 프레임에 추가하십시오
append()
메소드는 사전의 값을 행으로 직접 가져 와서 Pandas DataFrame
에 추가 할 수 있습니다.
예제 코드:
# python 3.x
import pandas as pd
# List of Tuples
fruit_list = [("Orange", 34, "Yes")]
# Create a DataFrame object
df = pd.DataFrame(fruit_list, columns=["Name", "Price", "Stock"])
# Add new ROW
df = df.append({"Name": "Apple", "Price": 23, "Stock": "No"}, ignore_index=True)
df = df.append({"Name": "Mango", "Price": 13, "Stock": "Yes"}, ignore_index=True)
print(df)
출력:
Name Price Stock
0 Orange 34 Yes
1 Apple 23 No
2 Mango 13 Yes
행을 추가하는 데이터 프레임append()
메소드
append()
메소드는 다른DataFrame
의 행을 원래DataFrame
의 끝에 추가하고 새로운DataFrame
을 리턴 할 수 있습니다. 원래datafarme
에없는 새DataFrame
의 열도 기존DataFrame
에 추가되고 새 셀 값은NaN
으로 채워집니다.
예제 코드:
import pandas as pd
fruit_list = [("Orange", 34, "Yes")]
df = pd.DataFrame(fruit_list, columns=["Name", "Price", "Stock"])
print("Original DataFrame:")
print(df)
print(".............................")
print(".............................")
new_fruit_list = [("Apple", 34, "Yes", "small")]
dfNew = pd.DataFrame(new_fruit_list, columns=["Name", "Price", "Stock", "Type"])
print("Newly Created DataFrame:")
print(dfNew)
print(".............................")
print(".............................")
# append one dataframe to othher
df = df.append(dfNew, ignore_index=True)
print("Copying DataFrame to orignal...")
print(df)
ignore_index=True
는 새로운DataFrame
의index
를 무시하고 원래DataFrame
에서 새로운 인덱스를 할당합니다.
출력:
Original DataFrame:
Name Price Stock
0 Orange 34 Yes
.............................
.............................
Newly Created DataFrame:
Name Price Stock Type
0 Apple 34 Yes small
.............................
.............................
Copying DataFrame to original..:
Name Price Stock Type
0 Orange 34 Yes NaN
1 Apple 34 Yes small
튜토리얼이 마음에 드시나요? DelftStack을 구독하세요 YouTube에서 저희가 더 많은 고품질 비디오 가이드를 제작할 수 있도록 지원해주세요. 구독하다