Pandas 데이터 프레임을 Excel 파일로 내보내기
-
to_excel()
함수를 사용하여 PandasDataFrame
을 Excel 파일로 내보내기 -
ExcelWriter()
메서드를 사용하여 PandasDataFrame
내보내기 -
여러 Pandas
DataFrame
를 여러 Excel 시트로 내보내기
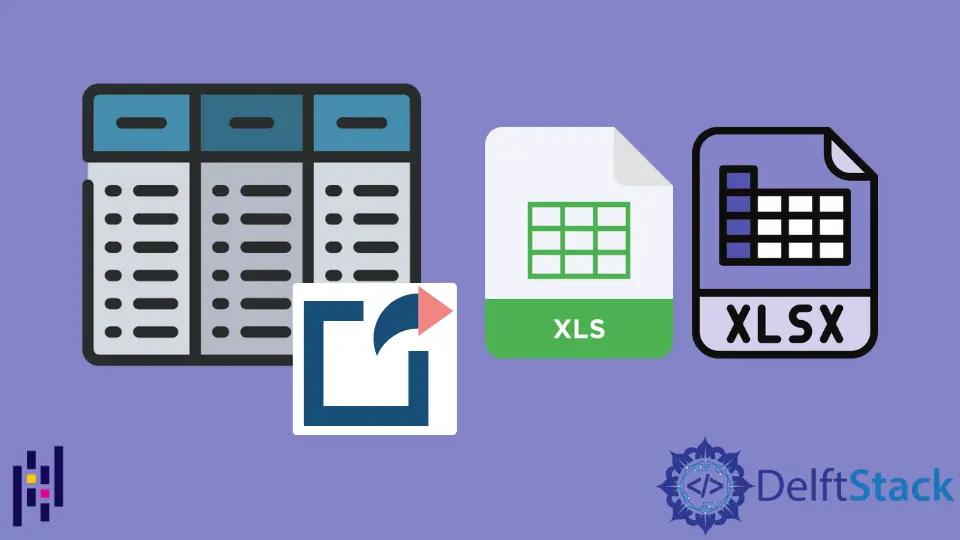
이 튜토리얼에서는 두 가지 다른 방법을 사용하여 팬더 DataFrame
을 Excel 파일로 내보내는 방법을 보여줍니다. 첫 번째 방법은 파일명으로 to_excel()
함수를 호출하여 pandas DataFrame
을 Excel 파일로 내보내는 것입니다. 이 기사에서 논의된 다른 방법은 ExcelWriter()
방법입니다. 이 방법은 개체를 Excel 시트에 작성한 다음 to_excel
기능을 사용하여 Excel 파일로 내보냅니다.
이 가이드에서는 ExcelWriter()
메서드를 사용하여 여러 Pandas dataframes
를 여러 Excel 시트에 추가하는 방법도 설명합니다. 또한 각 방법을 자세히 설명하기 위해 시스템에서 여러 예제를 실행했습니다.
to_excel()
함수를 사용하여 Pandas DataFrame
을 Excel 파일로 내보내기
pandas DataFrame
을 dataframe.to_excel()
함수를 사용하여 Excel 시트로 내보내면 Excel 시트에 개체를 직접 씁니다. 이 방법을 구현하려면 DataFrame
을 만든 다음 Excel 파일의 이름을 지정합니다. 이제 dataframe.to_excel()
함수를 사용하여 pandas DataFrame
을 Excel 파일로 내보냅니다.
다음 예에서는 Products_ID
, Product_Names
, Product_Prices
, Product_Sales
열을 포함하는 sales_record
라는 DataFrame
을 만들었습니다. 그런 다음 Excel 파일 ProductSales_sheet.xlsx
의 이름을 지정했습니다. sales_record.to_excel()
메서드를 사용하여 모든 데이터를 Excel 시트에 저장했습니다.
아래 예제 코드를 참조하십시오.
import pandas as pd
# DataFrame Creation
sales_record = pd.DataFrame(
{
"Products_ID": {
0: 101,
1: 102,
2: 103,
3: 104,
4: 105,
5: 106,
6: 107,
7: 108,
8: 109,
},
"Product_Names": {
0: "Mosuse",
1: "Keyboard",
2: "Headphones",
3: "CPU",
4: "Flash Drives",
5: "Tablets",
6: "Android Box",
7: "LCD",
8: "OTG Cables",
},
"Product_Prices": {
0: 700,
1: 800,
2: 200,
3: 2000,
4: 100,
5: 1500,
6: 1800,
7: 1300,
8: 90,
},
"Product_Sales": {0: 5, 1: 13, 2: 50, 3: 4, 4: 100, 5: 50, 6: 6, 7: 1, 8: 50},
}
)
# Specify the name of the excel file
file_name = "ProductSales_sheet.xlsx"
# saving the excelsheet
sales_record.to_excel(file_name)
print("Sales record successfully exported into Excel File")
출력:
Sales record successfully exported into Excel File
위 소스를 실행하면 현재 실행 중인 프로젝트 폴더에 ProductSales_sheet.xlsx
엑셀 파일이 저장됩니다.
ExcelWriter()
메서드를 사용하여 Pandas DataFrame
내보내기
Excelwrite()
메서드는 팬더 DataFrame
을 Excel 파일로 내보낼 때도 유용합니다. 먼저 Excewriter()
메서드를 사용하여 개체를 Excel 시트에 작성한 다음 dataframe.to_excel()
함수를 사용하여 DataFrame
을 Excel 파일로 내보낼 수 있습니다.
아래 예제 코드를 참조하십시오.
import pandas as pd
students_data = pd.DataFrame(
{
"Student": ["Samreena", "Ali", "Sara", "Amna", "Eva"],
"marks": [800, 830, 740, 910, 1090],
"Grades": ["B+", "B+", "B", "A", "A+"],
}
)
# writing to Excel
student_result = pd.ExcelWriter("StudentResult.xlsx")
# write students data to excel
students_data.to_excel(student_result)
# save the students result excel
student_result.save()
print("Students data is successfully written into Excel File")
출력:
Students data is successfully written into Excel File
여러 Pandas DataFrame
를 여러 Excel 시트로 내보내기
위의 방법에서 단일 팬더 DataFrame
을 Excel 시트로 내보냈습니다. 그러나 이 방법을 사용하면 여러 팬더 dataframes
를 여러 Excel 시트로 내보낼 수 있습니다.
여러 dataframes
를 여러 Excel 시트로 개별적으로 내보낸 다음 예를 참조하십시오.
import pandas as pd
import numpy as np
import xlsxwriter
# Creating records or dataset using dictionary
Science_subject = {
"Name": ["Ali", "Umar", "Mirha", "Asif", "Samreena"],
"Roll no": ["101", "102", "103", "104", "105"],
"science": ["88", "60", "66", "94", "40"],
}
Computer_subject = {
"Name": ["Ali", "Umar", "Mirha", "Asif", "Samreena"],
"Roll no": ["101", "102", "103", "104", "105"],
"computer_science": ["73", "63", "50", "95", "73"],
}
Art_subject = {
"Name": ["Ali", "Umar", "Mirha", "Asif", "Samreena"],
"Roll no": ["101", "102", "103", "104", "105"],
"fine_arts": ["95", "63", "50", "60", "93"],
}
# Dictionary to Dataframe conversion
dataframe1 = pd.DataFrame(Science_subject)
dataframe2 = pd.DataFrame(Computer_subject)
dataframe3 = pd.DataFrame(Art_subject)
with pd.ExcelWriter("studentsresult.xlsx", engine="xlsxwriter") as writer:
dataframe1.to_excel(writer, sheet_name="Science")
dataframe2.to_excel(writer, sheet_name="Computer")
dataframe3.to_excel(writer, sheet_name="Arts")
print("Please check out subject-wise studentsresult.xlsx file.")
출력:
Please check out subject-wise studentsresult.xlsx file.