jQuery에서 확인란을 켜고 끕니다.
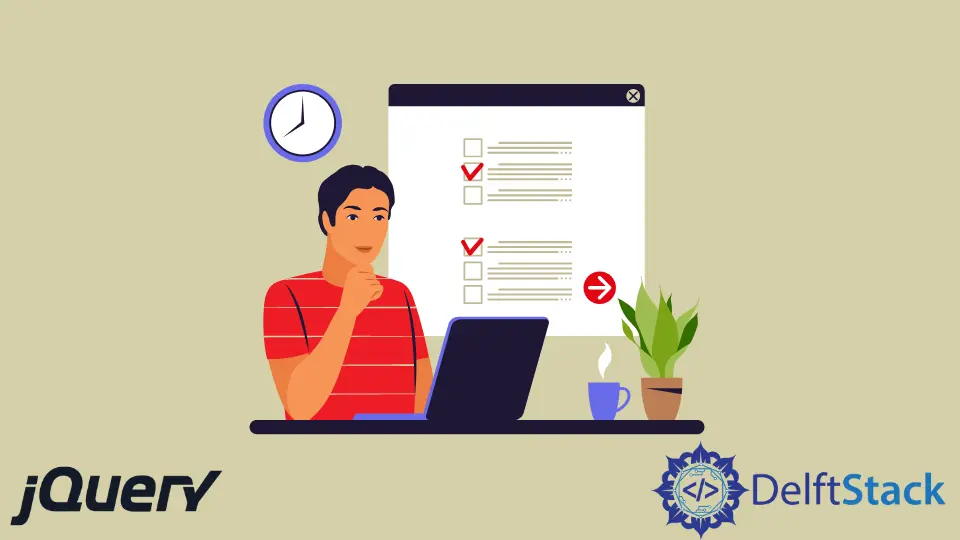
이 문서에서는 jQuery를 사용하여 한 번에 여러 확인란을 전환할 수 있는 세 가지 방법을 설명합니다. 세 가지 방법은 jQuery에서 사용할 수 있는 세 가지 방법을 사용합니다. 이들은 .prop()
, .each()
및 .trigger()
입니다.
.prop()
메서드를 사용하여 확인란 전환
jQuery에서는 .prop()
메서드를 사용하여 HTML 요소의 속성을 설정할 수 있습니다. 여기에서 코드의 체크박스에서 체크박스를 사용하여 선택됨
과 선택되지 않음
사이에서 상태를 전환할 수 있습니다.
다음 예제는 수행 방법을 보여줍니다. 그 안에는 HTML과 jQuery 코드가 있습니다. HTML에는 체크박스가 포함되어 있으며 첫 번째 체크박스를 클릭하면 모두 체크됩니다.
jQuery 코드의 첫 번째 체크박스에 click
이벤트를 추가했습니다. 그런 다음 나머지 확인란의 checked
속성을 조작합니다.
첫 번째 확인란을 처음 선택하면 모든 확인란이 선택됩니다. 선택을 취소하면 나머지 확인란이 기본 상태로 돌아갑니다. 선택 취소
.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>01-toggle-checkboxes</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.1/jquery.min.js" integrity="sha512-aVKKRRi/Q/YV+4mjoKBsE4x3H+BkegoM/em46NNlCqNTmUYADjBbeNefNxYV7giUp0VxICtqdrbqU7iVaeZNXA==" crossorigin="anonymous" referrerpolicy="no-referrer"></script>
<style>
form {
outline: 2px solid #1a1a1a;
width: 50%;
margin: 0 auto;
padding: 0.5em;
}
label {
display: block;
cursor: pointer;
font-size: 1.2em;
}
label:first-child:hover {
background-color: #aaa;
}
</style>
</head>
<body>
<main>
<form id="form">
<label>
<input type="checkbox" id="select-lang"> Select all languages
</label>
<label>
<input type="checkbox" class="tech"> Cascading Style Sheets
</label>
<label>
<input type="checkbox" class="tech"> Hypertext Markup Language
</label>
</form>
</main>
<script>
$(document).ready(function() {
$("#select-lang").click(function() {
let check_boxes = $("input.tech");
// Swap the "prop" attribute each time the
// user clicks the "select-lang" checkbox.
check_boxes.prop("checked", !check_boxes.prop("checked"));
});
});
</script>
</body>
</html>
출력:
.each()
메서드를 사용하여 확인란 전환
체크박스를 토글하는 .each()
메서드는 .prop()
에서 보여준 것과 같이 작동합니다. 그러나 차이점이 있습니다. 각 확인란을 반복하는 함수를 사용합니다.
이 반복 동안 체크박스의 상태를 선택됨
에서 선택되지 않음
으로 변경했습니다. 다음 코드는 수행 방법을 보여줍니다.
또한 첫 번째 예에서 HTML을 업데이트했습니다. 이번에는 확인란에 국가 이름이 포함됩니다.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>02-toggle-checkboxes</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.1/jquery.min.js" integrity="sha512-aVKKRRi/Q/YV+4mjoKBsE4x3H+BkegoM/em46NNlCqNTmUYADjBbeNefNxYV7giUp0VxICtqdrbqU7iVaeZNXA==" crossorigin="anonymous" referrerpolicy="no-referrer"></script>
<style>
form {
outline: 2px solid #1a1a1a;
width: 50%;
margin: 0 auto;
padding: 0.5em;
}
label {
display: block;
cursor: pointer;
font-size: 1.2em;
}
label:first-child:hover {
background-color: #aaa;
}
</style>
</head>
<body>
<main>
<form id="form">
<label>
<input type="checkbox" id="select-country"> Select all countries
</label>
<label>
<input type="checkbox" class="country"> Georgia
</label>
<label>
<input type="checkbox" class="country"> Turkmenistan
</label>
<label>
<input type="checkbox" class="country"> Azerbaijan
</label>
</form>
</main>
<script>
$(document).ready(function() {
$("#select-country").click(function() {
// Use the ".each()" API to iterate over the
// countries and alternate them between "checked"
// and "unchecked".
$('input.country').each(function () {
this.checked = !this.checked;
});
});
});
</script>
</body>
</html>
출력:
.trigger()
메서드를 사용하여 확인란 전환
.trigger()
메서드를 사용하여 확인란을 전환하는 것은 .prop()
및 .each()
를 사용하는 것과 다르게 작동합니다. 이번에는 .trigger()
메서드를 사용하여 첫 번째 체크박스를 클릭할 때 모든 체크박스에 클릭 기능을 실행합니다.
또 다른 방법은 각 체크박스에서 .click()
메서드를 호출하는 것입니다. 둘 다 잘 작동합니다.
하지만 코드에 주석으로 남겨두었고 나중에 사용해 볼 수 있습니다. HTML은 동일하게 유지되지만 확인란에는 이제 인터넷의 상위 웹사이트 이름이 포함됩니다.
아래 코드 블록에서 이 모든 것을 찾을 수 있으며 브라우저의 출력은 다음과 같습니다.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>03-toggle-checkboxes</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.1/jquery.min.js" integrity="sha512-aVKKRRi/Q/YV+4mjoKBsE4x3H+BkegoM/em46NNlCqNTmUYADjBbeNefNxYV7giUp0VxICtqdrbqU7iVaeZNXA==" crossorigin="anonymous" referrerpolicy="no-referrer"></script>
<style>
form {
outline: 2px solid #1a1a1a;
width: 50%;
margin: 0 auto;
padding: 0.5em;
}
label {
display: block;
cursor: pointer;
font-size: 1.2em;
}
label:first-child:hover {
background-color: #aaa;
}
</style>
</head>
<body>
<main>
<form id="form">
<label>
<input type="checkbox" id="select-websites"> Select all websites
</label>
<label>
<input type="checkbox" class="website"> Facebook
</label>
<label>
<input type="checkbox" class="website"> Amazon
</label>
<label>
<input type="checkbox" class="website"> Apple
</label>
<label>
<input type="checkbox" class="website"> Netflix
</label>
<label>
<input type="checkbox" class="website"> Google
</label>
</form>
</main>
<script>
$(document).ready(function() {
$("#select-websites").click(function() {
// Use the ".trigger()"" API to execute the
// click function on each checkbox
$('input.website').trigger('click');
// Another option is to execute the "click"
// API directly on the checkboxes
// $('input.website').click();
});
});
</script>
</body>
</html>
출력:
Habdul Hazeez is a technical writer with amazing research skills. He can connect the dots, and make sense of data that are scattered across different media.
LinkedIn