jQuery로 모든 클래스 제거
-
.removeClass()
메서드를 사용하여 jQuery에서 클래스 제거 -
.removeAttr()
메서드를 사용하여 jQuery에서 클래스 제거 -
.attr()
메서드를 사용하여 jQuery에서 클래스 제거 -
JavaScript
className
속성을 사용하여 jQuery에서 클래스 제거
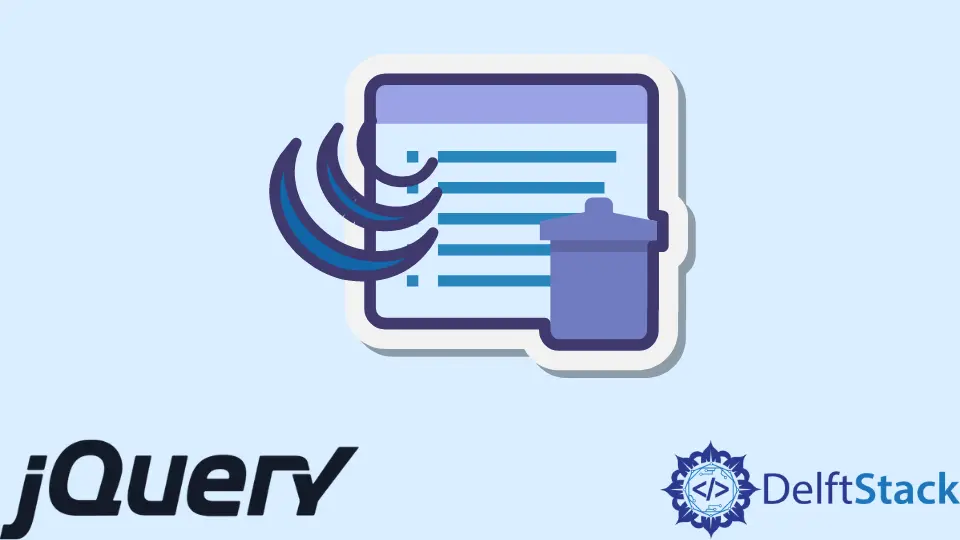
jQuery로 모든 클래스를 제거하려면 3개의 jQuery 메서드와 1개의 JavaScript 속성을 사용할 수 있습니다. jQuery 메서드는 .removeClass()
, .removeAttr()
및 .attr()
이며 JavaScript 속성은 className
속성입니다.
이 문서에서는 실용적인 코드 예제와 함께 모두 사용하는 방법을 알려줍니다.
.removeClass()
메서드를 사용하여 jQuery에서 클래스 제거
.removeClass()
메서드를 사용하면 jQuery를 사용하여 요소에서 CSS 클래스를 제거할 수 있습니다. 그러나 특정 클래스 이름을 전달하지 않으면 해당 요소에서 모든 CSS 클래스가 제거됩니다.
이것이 다음 예제의 전부입니다. 버튼을 누르면 단락에서 CSS 클래스가 제거됩니다.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>01-jQuery-remove-all-classes</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.1/jquery.min.js" integrity="sha512-aVKKRRi/Q/YV+4mjoKBsE4x3H+BkegoM/em46NNlCqNTmUYADjBbeNefNxYV7giUp0VxICtqdrbqU7iVaeZNXA==" crossorigin="anonymous" referrerpolicy="no-referrer"></script>
<style>
#btn_remove_all_classes {
padding: 1.2em;
background-color: #1a1a1a;
color: #ffffff;
cursor: pointer;
border-radius: 20px;
}
/* CSS styles for the paragraph that'll be
* removed using jQuery.
*/
.underline {
text-decoration: underline;
text-underline-position: under;
}
.bold {
font-weight: bold;
}
.font-size-3em {
font-size: 3em;
}
</style>
</head>
<body>
<main>
<button id="btn_remove_all_classes">Remove all classes from paragraph</button>
<p class="underline bold font-size-3em">
This paragraph has three CSS styles. Click the button above to remove them!
</p>
</main>
<script>
$(document).ready(function() {
$("#btn_remove_all_classes").click(function() {
// Delete the CSS classes using the removeClass method
// in jQuery.
$("main p").removeClass();
// EXTRA: Remove the click event.
$("#btn_remove_all_classes").off('click');
});
});
</script>
</body>
</html>
출력:
.removeAttr()
메서드를 사용하여 jQuery에서 클래스 제거
.removeAttr()
메서드는 요소에서 무엇을 제거하는지에 따라 다릅니다. 여기에서 요소의 class
특성을 전달할 수 있으며 모든 클래스가 삭제됩니다.
다음에서는 .removeAttr()
을 사용하여 단락에서 4개의 CSS 클래스를 제거하도록 jQuery 코드를 업데이트했습니다. 새로 스타일이 지정된 버튼을 클릭하면 웹 브라우저에서 결과를 볼 수 있습니다.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>02-jQuery-remove-all-classes</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.1/jquery.min.js" integrity="sha512-aVKKRRi/Q/YV+4mjoKBsE4x3H+BkegoM/em46NNlCqNTmUYADjBbeNefNxYV7giUp0VxICtqdrbqU7iVaeZNXA==" crossorigin="anonymous" referrerpolicy="no-referrer"></script>
<style>
#btn_remove_all_classes {
padding: 1.2em;
background-color: #ff6347;
color: #ffffff;
cursor: pointer;
border-radius: 20px;
}
/* CSS styles for the paragraph that'll be
* removed using jQuery.
*/
.italic {
font-style: italic;
}
.color-blue {
color: #1560bd;
}
.trebuchet-ms-font {
font-family: "Trebuchet MS";
}
.font-size-3em {
font-size: 3em;
}
</style>
</head>
<body>
<main>
<button id="btn_remove_all_classes">Remove all classes from paragraph</button>
<p class="italic color-blue trebuchet-ms-font font-size-3em">
Styled with CSS classes. Don't want them? Click the button above!
</p>
</main>
<script>
$(document).ready(function() {
$("#btn_remove_all_classes").click(function() {
// Delete the CSS classes using the removeAttr
// method in jQuery.
$("main p").removeAttr('class');
// EXTRA: Remove the click event.
$("#btn_remove_all_classes").off('click');
});
});
</script>
</body>
</html>
출력:
.attr()
메서드를 사용하여 jQuery에서 클래스 제거
.attr()
메소드는 .removeAttr()
메소드처럼 작동하여 요소에서 CSS 클래스를 제거합니다. 그러나 이번에는 .attr()
을 사용하여 class
속성을 빈 문자열로 설정합니다.
그 결과 일치하는 요소에서 모든 클래스가 제거됩니다. 다음은 이를 수행하는 방법을 보여줍니다. 다음은 Firefox 106.0.1
의 결과입니다.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>03-jQuery-remove-all-classes</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.1/jquery.min.js" integrity="sha512-aVKKRRi/Q/YV+4mjoKBsE4x3H+BkegoM/em46NNlCqNTmUYADjBbeNefNxYV7giUp0VxICtqdrbqU7iVaeZNXA==" crossorigin="anonymous" referrerpolicy="no-referrer"></script>
<style>
#btn_remove_all_classes {
padding: 1.2em;
background-color: #c069ff;
color: #ffffff;
cursor: pointer;
border-radius: 20px;
}
/* CSS styles for the paragraph that'll be
* removed using jQuery.
*/
.font-style-italic {
font-style: italic;
}
.writing-mode-vertical-lr {
writing-mode: vertical-lr;
}
.font-size-2em {
font-size: 2em;
}
</style>
</head>
<body>
<main>
<button id="btn_remove_all_classes">Remove all classes from paragraph</button>
<p class="font-style-italic writing-mode-vertical-lr font-size-2em">
Weird horizontal text. Click the button to reset it to normal view!
</p>
</main>
<script>
$(document).ready(function() {
$("#btn_remove_all_classes").click(function() {
// Delete the CSS classes using the attr
// method in jQuery.
$("main p").attr('class', '');
// EXTRA: Remove the click event.
$("#btn_remove_all_classes").off('click');
});
});
</script>
</body>
</html>
출력:
JavaScript className
속성을 사용하여 jQuery에서 클래스 제거
JavaScript className
속성을 빈 문자열로 설정하여 요소에서 CSS 클래스를 삭제할 수 있습니다. 예, 이것은 JavaScript 속성이지만 jQuery를 사용하여 요소를 선택합니다.
다음은 제거를 위해 className
속성을 사용할 업데이트된 jQuery 코드입니다. 또한 단락에는 이제 제거할 5개의 클래스가 있습니다.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>04-jQuery-remove-all-classes</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.1/jquery.min.js" integrity="sha512-aVKKRRi/Q/YV+4mjoKBsE4x3H+BkegoM/em46NNlCqNTmUYADjBbeNefNxYV7giUp0VxICtqdrbqU7iVaeZNXA==" crossorigin="anonymous" referrerpolicy="no-referrer"></script>
<style>
#btn_remove_all_classes {
padding: 1.2em;
background-color: #1560bd;
color: #ffffff;
cursor: pointer;
border-radius: 20px;
}
/* CSS styles for the paragraph that'll be
* removed using jQuery.
*/
.background-yellow {
background-color: #fed000;
}
.color-white {
color: #ffffff;
}
.text-align-center {
text-align: center;
}
.line-height-1618 {
line-height: 1.618
}
.font-size-2em {
font-size: 1.5em;
}
</style>
</head>
<body>
<main>
<button id="btn_remove_all_classes">Remove all classes from paragraph</button>
<p class="background-yellow color-white text-align-center line-height-1618 font-size-2em">
Just some random text with CSS styling.
</p>
</main>
<script>
$(document).ready(function() {
$("#btn_remove_all_classes").click(function() {
// Delete the CSS classes using JavaScript className
// property.
$("main p")[0].className = '';
// EXTRA: Remove the click event.
$("#btn_remove_all_classes").off('click');
});
});
</script>
</body>
</html>
출력:
Habdul Hazeez is a technical writer with amazing research skills. He can connect the dots, and make sense of data that are scattered across different media.
LinkedIn