JavaScript를 사용하여 이미지 업로드
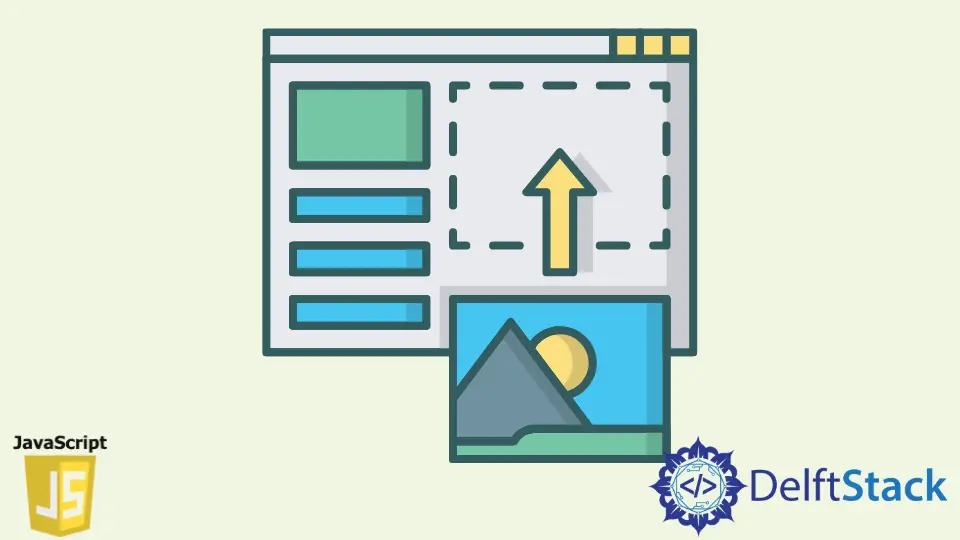
사용자는 본문 섹션에 파일 형식의 입력 필드를 생성하여 HTML 파일에서 시작하여 이미지를 업로드할 수 있습니다. 이 기사에서는 JavaScript를 사용하여 이미지를 업로드하는 방법의 예를 보여줍니다.
자바스크립트 업로드 이미지
정의된 너비와 높이가 있는 출력으로 업로드될 이미지를 표시하기 위해 display_image
라는 id와 함께 div 태그가 사용됩니다.
통사론:
<input type ="file" id ="image_input" accept ="image/jpeg, image/png, image/jpg">
<div id="display_image"></div>
업로드된 이미지가 내부에 완벽하게 맞도록 이미지 속성을 적절한 값으로 CSS 파일에 추가해야 합니다. 여기서 테두리는 반드시 추가해야 하는 필수 속성은 아니지만 사용자가 이미지가 나타나는 위치를 쉽게 볼 수 있도록 합니다.
#display-image{
width: 500px;
height: 325px;
border: 2px solid black;
background-position: center;
background-size: cover;
}
다음으로 입력 필드에 대한 액세스 권한을 얻고 사용자가 요소의 값을 변경했을 때 실행되는 이벤트인 "change"
이벤트 리스너를 첨부하기 위해 JavaScript 파일을 생성해야 합니다. 이 리스너 아래에서 사용자는 웹 애플리케이션이 사용자의 로컬 시스템에 저장된 파일 또는 원시 데이터 버퍼의 내용을 읽고 "load"
이벤트 리스너를 첨부할 수 있도록 하는 FileReader
개체에 대한 액세스 권한을 얻어야 합니다. 이미지, CSS 파일 및 JavaScript 파일과 같은 모든 종속 소스를 포함하여 전체 페이지가 완전히 로드됩니다.
간단히 말해서 업로드된 이미지는 표시 가능한 형식으로 변환됩니다. 업로드된 이미지는 uploadImage
변수에 저장되며 이 변수는 CSS에서 backgroundImage
속성을 초기화하는 데 사용됩니다.
const image_input = document.querySelector('#image_input');
image_input.addEventListener('change', function() {
const file_reader = new FileReader();
file_reader.addEventListener('load', () => {
const uploaded_image = file_reader.result;
document.querySelector('#display_image').style.backgroundImage =
`url(${uploaded_image})`;
});
file_reader.readAsDataURL(this.files[0]);
});
전체 소스 코드:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>JavaScript Upload Image </title>
<style>
#display_image{
width: 500px;
height: 325px;
border: 2px solid black;
background-position: center;
background-size: cover;
}
</style>
</head>
<body>
<input type="file" id="image_input" accept="image/jpeg, image/png, image/jpg">
<div id="display_image"></div>
<script>
const image_input = document.querySelector("#image_input");
image_input.addEventListener("change", function() {
const file_reader = new FileReader();
file_reader.addEventListener("load", () => {
const uploaded_image = file_reader.result;
document.querySelector("#display_image").style.backgroundImage = `url(${uploaded_image})`;
});
file_reader.readAsDataURL(this.files[0]);
});
</script>
</body>
</html>
출력:
정의된 너비 또는 높이가 없는 JavaScript 업로드 이미지
JavaScript에서 정의된 너비 또는 높이 없이 이미지를 원래 크기로 업로드할 수도 있습니다. 아래 단계를 따르십시오.
-
HTML 파일을 생성하여 시작하고 업로드된 이미지를 호출할 onchange 함수
filevalidation()
를 사용하여 ID를 파일로 할당하는 입력 유형 파일을 설정합니다. -
다음으로
var image = document.getElementById(‘uploaded image’);
를 통해 ID로 요소를 선택하기 위해filevaildation()
함수 내에서 문서 메소드.getElementById()
를 사용합니다. -
정적 메서드
URL.createObjectURL()
을 프로그램에 추가하여 URL을uploaded_image.src
로 전달하여 브라우저에서 사용자가 업로드한 이미지를uploaded_image.src=URL.createObjectURL(event.target.files[0]);
. -
사용자가 어떤 파일을 선택했는지 확인하기 위해
if(selected_file.files.length>0)
로 루프를 만들고 선택한 파일의 크기를 얻기 위해const file_size=selected_file.files.item(i).size;
사용할 수 있습니다. -
다음으로 파일 크기가 바이트로 변환됩니다.
Math.round((file_size/1024));
사용 기능 그것은 어떤 단위로 변환될 수 있습니다.
전체 소스 코드:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>JavaScript Upload Image</title>
</head>
<body>
<input type="file" id="file" onchange="FileValidation(event)" >
<br><br><br>
<h2>Uploaded Image by the User</h2>
<img id="uploaded image">
</body>
<script>
FileValidation = (event) => {
var uploaded_image = document.getElementById('uploaded image');
uploaded_image.src = URL.createObjectURL(event.target.files[0]);
const selected_file = document.getElementById("file");
if (selected_file.files.length > 0) {
for (const i = 0; i <= selected_file.files.length - 1; i++) {
const file_size = selected_file.files.item(i).size;
const file = Math.round((file_size / 1024));
}
}
}
</script>
</html>
출력:
위의 코드에서 사용자가 모든 파일 형식을 선택하여 업로드할 수 있지만 표시할 수는 없습니다. 추가 구현을 통해 요구 사항에 따라 위의 프로그램에 더 많은 코딩을 추가하여 여러 이미지 업로드를 수행할 수 있습니다.
Nimesha is a Full-stack Software Engineer for more than five years, he loves technology, as technology has the power to solve our many problems within just a minute. He have been contributing to various projects over the last 5+ years and working with almost all the so-called 03 tiers(DB, M-Tier, and Client). Recently, he has started working with DevOps technologies such as Azure administration, Kubernetes, Terraform automation, and Bash scripting as well.