JavaScript에서 클래스 확장
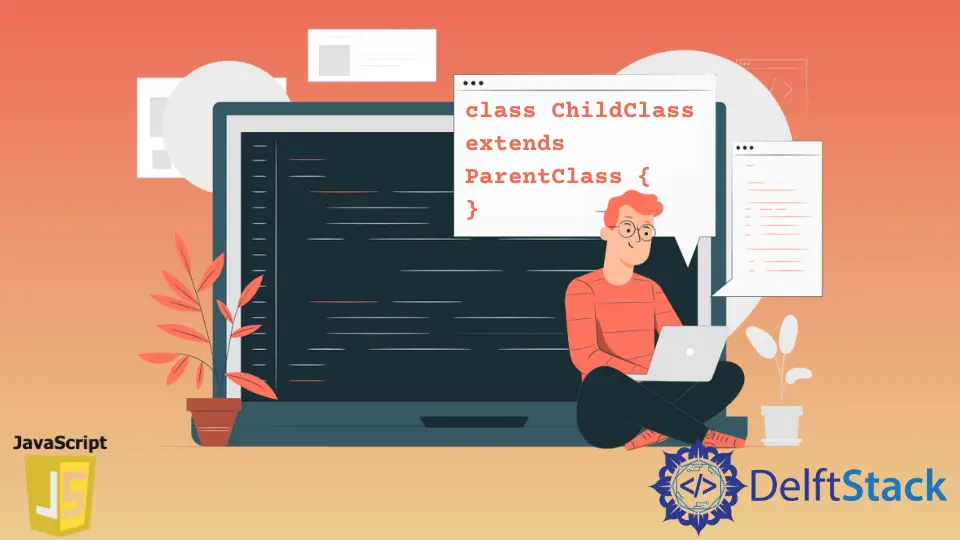
클래스는 개체를 만들기 위한 템플릿 또는 청사진입니다. 데이터를 코드로 캡슐화하여 해당 데이터로 작업할 수 있습니다.
JS의 클래스는 프로토타입을 기반으로 하지만 ES5의 클래스 의미와 공유되지 않는 구문 및 의미도 있습니다.
오늘 포스팅에서는 자바스크립트에서 클래스를 확장하는 방법에 대해 알아보겠습니다.
extends
키워드를 사용하여 JavaScript에서 클래스 확장
extends
키워드는 기본 클래스에서 사용자 지정 클래스 및 기본 제공 개체를 파생시킬 수 있습니다. 다른 클래스의 자식인 클래스를 만들기 위해 클래스 식이나 클래스 선언에 사용됩니다.
통사론:
class ChildClass extends ParentClass { /* Logic goes here */
}
문서 여기에서 extends
에 대한 자세한 정보를 찾을 수 있습니다.
예를 들어 부모 클래스의 이름 속성과 숫자 값을 저장하는 카운터로 구성된 ParentClass
가 있습니다. ParentClass
에는 부모 클래스 이름 속성의 현재 인스턴스를 표시하는 정적 메서드도 포함되어 있습니다.
ChildClass
는 ParentClass
의 속성을 확장합니다. 부모 클래스를 확장하면 자식 클래스는 모든 부모 클래스 속성에 액세스할 수 있습니다.
예약된 키워드 super
는 슈퍼 생성자 호출을 실행할 수 있으며 부모 메서드에 대한 액세스를 제공합니다.
예:
class ParentClass {
constructor(counter) {
this.name = 'ParentClass';
this.counter = counter;
}
sayClassName() {
console.log('Hi, I am a ', this.name + '.');
}
}
const parent = new ParentClass(300);
parent.sayClassName();
console.log('The counter of this parent class is ' + parent.counter);
class ChildClass extends ParentClass {
constructor(counter) {
super(counter);
this.name = 'ChildClass';
}
get counter() {
return this.counter;
}
set counter(value) {
this.childCounter = value;
}
}
const child = new ChildClass(5);
child.sayClassName();
console.log('The counter of this parent class is ' + child.childCounter);
카운터를 300으로 설정하는 부모 클래스 인스턴스를 만들었습니다. 자식 클래스가 생성되자마자 카운터를 5로 업데이트합니다.
하위 클래스에는 카운터 속성이 없습니다. 부모 클래스에서 상속됩니다.
출력:
"Hi, I am a ", "ParentClass."
"The counter of this parent class is 300"
"Hi, I am a ", "ChildClass."
"The counter of this parent class is 5"
여기에서 예제의 전체 코드에 액세스할 수도 있습니다.
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn