범위를 벗어난 Java Lang 색인에 대한 솔루션
-
java.lang.IndexOutOfBoundsException
의 원인 -
ArrayList를 고려한
java.lang.IndexOutOfBoundsException
에 대한 솔루션 -
배열을 고려한
java.lang.ArrayIndexOutOfBoundsException
에 대한 솔루션
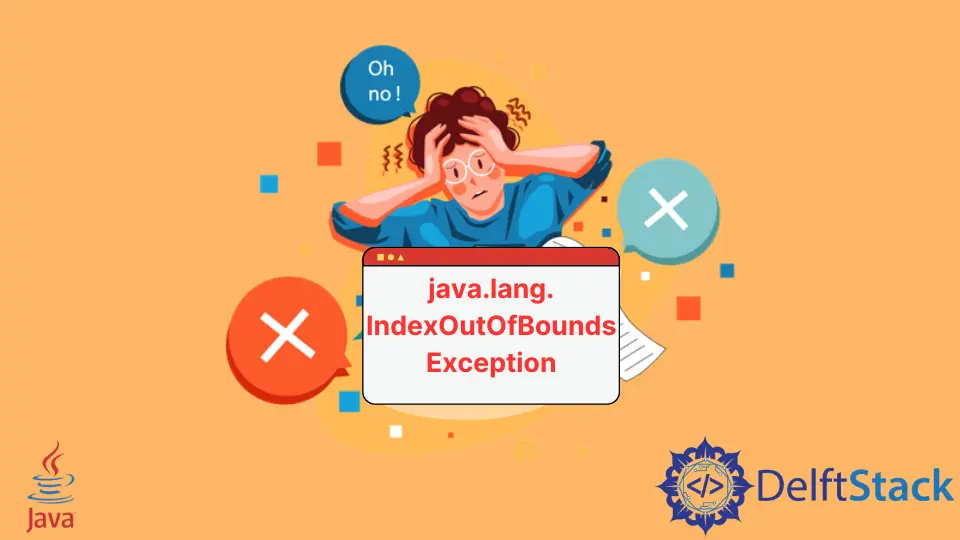
오늘은 Array와 ArrayList를 고려한 IndexOutOfBoundsException
오류에 대해 알아보겠습니다. 또한 이 오류가 발생하는 이유와 마지막으로 해결 방법을 이해할 것입니다.
java.lang.IndexOutOfBoundsException
의 원인
IndexOutOfBoundsException
오류가 발생하는 이유는 Arrays 및 ArrayList에서 비슷하지만 오류 설명이 다른 한 가지 차이점이 있습니다. Arrays 및 ArrayList에서 각각 java.lang.ArrayIndexOutOfBoundsException
및 java.lang.IndexOutOfBoundsException
으로 이 오류가 발생합니다.
이 예외가 발생하는 이유는 다음과 같습니다.
- ArrayList가 비어 있고 첫 번째 인덱스의 값인 0에 액세스하려고 시도하는 경우.
- 음수 인덱스에서 Array 또는 ArrayList의 항목에 액세스하려고 할 때.
- Array 또는 ArrayList의 잘못된 인덱스에 액세스하려고 할 때 음수이거나 Array 또는 ArrayList의 크기보다 크거나 같을 수 있습니다.
IndexOutOfBoundsException
은 컴파일 시간에 Java 컴파일러에 의해 감지되지 않는 런타임 예외임을 기억하십시오. 따라서 Array 또는 ArrayList에 액세스하는 방법을 알아야 합니다.
경험 법칙에 따르면 Array 또는 ArrayList는 액세스하기 전에 채워져야 합니다. 또한 0 <= index < (Array/ArrayList 크기)
조건을 만족하는 인덱스에만 액세스할 수 있습니다.
코드 예제를 통해 배워봅시다.
ArrayList를 고려한 java.lang.IndexOutOfBoundsException
에 대한 솔루션
예제 코드:
import java.util.ArrayList;
public class TestArrayList {
public static void main(String[] args) {
ArrayList<String> list = new ArrayList<>();
// we try to get value at index 0 which is
// empty right now
System.out.println(list.get(0));
list.add("Mehvish"); // saves at index 0
list.add("Ashiq"); // saves at index 1
}
}
위의 코드는 아직 채워지지 않은 인덱스 0
에 액세스하기 때문에 IndexOutOfBoundsException
오류를 발생시킵니다.
이 오류를 해결하려면 먼저 ArrayList에 항목을 추가한 다음 유효하지 않은 인덱스에 액세스하지 않는지 확인하여 액세스해야 합니다. 다음 코드에서는 IndexOutOfBoundsException
을 유발할 수 있는 모든 상황을 처리했습니다.
예제 코드:
import java.util.ArrayList;
public class TestArrayList {
// create array list object of String type
static ArrayList<String> list = new ArrayList<>();
// populate array list
static void populateList() {
list.add("Mehvish"); // saves at index 0
list.add("Ashiq"); // saves at index 1
}
/*
Access the index only if the index
is valid and the list is not empty. Otherwise, print
the message to inform the user
*/
static void getElement(int index) {
if (list.size() != 0 && index >= 0 && index < list.size()) {
System.out.println(list.get(index));
} else {
System.out.println("The list is empty or you have entered an invalid index");
}
}
// main method
public static void main(String[] args) {
populateList();
getElement(0);
}
}
다양한 입력에서 이 코드를 테스트해 보겠습니다.
테스트 1: 사용자가 0
을 getElement()
함수에 전달하면 출력은 다음과 같습니다.
Mehvish
테스트 2: 사용자가 1
을 getElement()
함수에 전달하면 출력은 다음과 같습니다.
Ashiq
테스트 3: 사용자가 2
를 getElement()
함수에 전달하면 출력은 다음과 같습니다.
The list is empty or you have entered an invalid index
테스트 4: 사용자가 -1
을 getElement()
함수에 전달하면 출력은 다음과 같습니다.
The list is empty or you have entered an invalid index
테스트 5: 사용자가 populateList()
함수에 대해 설명하고 유효한 인덱스를 전달합니다. 출력은 다음과 같습니다.
The list is empty or you have entered an invalid index
배열을 고려한 java.lang.ArrayIndexOutOfBoundsException
에 대한 솔루션
예제 코드:
public class TestArray {
public static void main(String[] args) {
int array[] = {1, 2, 3, 4, 5};
for (int i = 0; i <= array.length; i++) System.out.println(array[i]);
}
}
이 코드 예제는 유효하지 않은 인덱스 5
에 액세스를 시도하기 때문에 ArrayIndexOfBoundsException
을 발생시킵니다. 유효하지 않은 색인에는 액세스할 수 없습니다.
가능한 모든 상황을 처리하고 ArrayIndexOutOfBoundsException
오류를 피한 다음 코드를 테스트해 보겠습니다.
예제 코드:
import java.util.ArrayList;
public class TestArray {
// required size of an array
static int size = 2;
// declare an int type array of specified size
static int array[] = new int[size];
// populate array
static void populateArray() {
for (int i = 0; i < size; i++) array[i] = i + 1;
}
// get element if a valid index is passed
static void getElement(int index) {
if (array.length != 0 && index >= 0 && index < array.length) {
System.out.println(array[index]);
} else {
System.out.println("The array is empty or you have entered an invalid index");
}
}
// main method
public static void main(String[] args) {
populateArray();
getElement(1);
}
}
다른 사용자 입력에서 이 코드를 테스트해 보겠습니다.
테스트 1: 사용자가 0
을 getElement()
함수에 전달하면 출력은 다음과 같습니다.
1
테스트 2: 사용자가 1
을 getElement()
함수에 전달하면 출력은 다음과 같습니다.
2
테스트 3: 사용자가 2
를 getElement()
함수에 전달하면 출력은 다음과 같습니다.
The array is empty or you have entered an invalid index
테스트 4: 사용자가 -1
을 getElement()
함수에 전달하면 출력은 다음과 같습니다.
The array is empty or you have entered an invalid index
테스트 5: 사용자가 populateArray()
함수에 대해 설명하고 유효한 인덱스를 전달합니다. 출력은 다음과 같습니다.
0
왜 0
입니까? int 유형의 기본값인 0
이 빈 배열을 초기화했기 때문입니다. 요소를 초기화하지 않으면 숫자 배열 요소의 기본값은 0
으로 설정되고 참조 요소는 null
로 설정됩니다.
관련 문장 - Java Error
- $' ' 수정: Java에서 명령을 찾을 수 없음 오류
- Android Java.Lang.IllegalStateException 수정: 활동의 메서드를 실행할 수 없음
- Eclipse 오류: 선택 항목을 실행할 수 없습니다.
- Eclipse에서 'Java Virtual Machine을 찾을 수 없음' 오류 수정
- Eclipse에서 Java Runtime Environment를 계속하기 위한 메모리가 부족합니다.
- Fix Class X Is Public은 X.java 오류라는 파일에서 선언해야 합니다.