Java의 push() 함수
-
Java에서
stack.push()
함수 사용 -
Java에서
LinkedList.push()
함수 사용 -
Java에서
ArrayList.add()
함수 사용 -
Java의 배열에 사용자 정의
push()
함수 사용
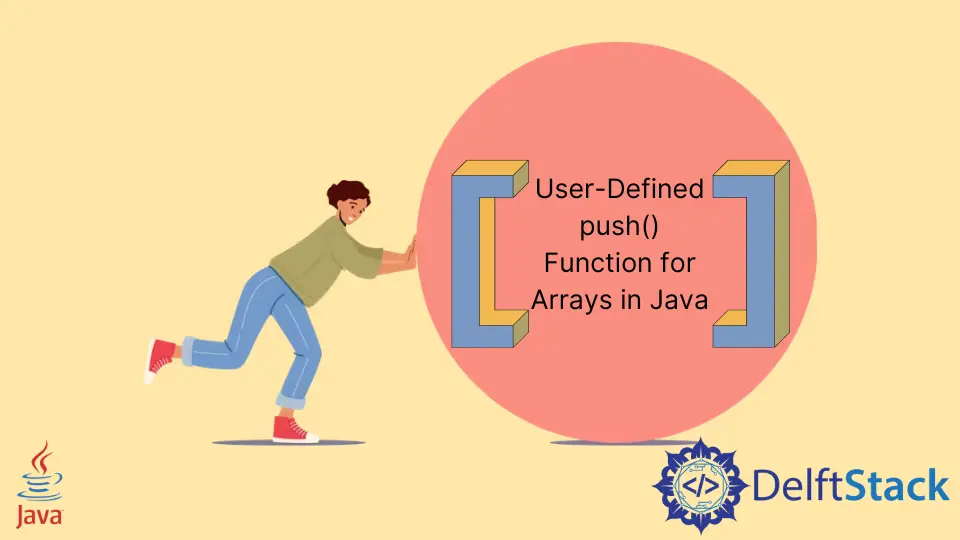
push()
함수의 기본 정의에 대해 이야기하면 어떤 구조의 끝에 요소를 삽입하는 함수가 될 것입니다. 이 함수는 스택, 연결 목록 등과 같은 후입선출 구조와 연결됩니다. Java에는 배열에 대한 push()
함수가 없습니다.
push()
함수는 배열과 연관되지 않으므로 이 함수를 이미 지원하는 다른 데이터 구조를 사용할 수 있습니다.
이 기사에서는 Java의 push()
함수에 대해 설명합니다.
Java에서 stack.push()
함수 사용
스택 클래스에서 push()
함수를 사용할 수 있습니다. 이를 위해 스택 클래스를 사용하기 위해 java.util
패키지를 가져올 것입니다.
이 함수를 사용하여 스택 끝에 요소를 추가할 수 있습니다. 스택은 원하는 유형일 수 있습니다.
스트링 타입의 스택 메소드를 생성할 것입니다. push()
함수를 사용하여 요소를 하나씩 추가합니다.
아래 코드를 참조하십시오.
import java.util.*;
public class Push_Example {
public static void main(String args[]) {
Stack<String> st = new Stack<String>();
st.push("Ram");
st.push("shayam");
st.push("sharma");
System.out.println("Stack Elements: " + st);
st.push("monu");
st.push("sonu");
// Stack after adding new elements
System.out.println("Stack after using the push function: " + st);
}
}
출력:
Stack Elements: [Ram, shayam, sharma]
Stack after using the push function: [Ram, shayam, sharma, monu, sonu]
Java에서 LinkedList.push()
함수 사용
Java에서 push()
함수는 Linked List와도 연관됩니다. 이를 위해 java.util
패키지도 가져올 것입니다.
LinkedList
메서드를 사용하여 새 연결 목록을 정의할 수 있습니다. 이제 push()
함수를 사용하여 요소를 하나씩 추가할 수 있습니다.
예를 들어,
import java.util.*;
public class Push_Example {
public static void main(String args[]) {
LinkedList<Integer> li = new LinkedList<>();
li.push(10);
li.push(11);
li.push(12);
li.push(13);
li.push(14);
System.out.println("LinkedList Elements: " + li);
// Push new elements
li.push(100);
li.push(101);
System.out.println("LinkedList after using the push function: " + li);
}
}
출력:
LinkedList Elements: [14, 13, 12, 11, 10]
LinkedList after using the push function: [101, 100, 14, 13, 12, 11, 10]
Java에서 ArrayList.add()
함수 사용
ArrayList의 경우 add()
함수를 사용하여 push()
함수를 에뮬레이트할 수 있습니다. 이 함수는 주어진 ArrayList의 끝에 요소를 추가합니다.
예를 들어,
import java.util.*;
public class Push_Example {
public static void main(String args[]) {
ArrayList<Integer> li = new ArrayList<>();
li.add(10);
li.add(11);
li.add(12);
li.add(13);
li.add(14);
System.out.println("ArrayList Elements: " + li);
// Push new elements
li.add(100);
li.add(101);
System.out.println("ArrayList after using the add function: " + li);
}
}
출력:
ArrayList Elements: [10, 11, 12, 13, 14]
ArrayList after using the add function: [10, 11, 12, 13, 14, 100, 101]
Java의 배열에 사용자 정의 push()
함수 사용
Java에는 배열에 대한 push()
함수가 없습니다. 그러나 이것을 에뮬레이트하는 함수를 만들 수 있습니다. 이 함수는 배열 내용을 더 긴 길이의 새 배열로 복사하고 이 배열에 새 요소를 추가합니다.
아래 코드를 참조하십시오.
import java.util.*;
public class Push_Arr {
private static String[] push(String[] array, String push) {
String[] longer = new String[array.length + 1];
for (int i = 0; i < array.length; i++) longer[i] = array[i];
longer[array.length] = push;
return longer;
}
public static void main(String args[]) {
String[] arr = new String[] {"a", "b", "c"};
arr = Push_Arr.push(arr, "d");
System.out.println("Array after using the push function: ");
for (int i = 0; i < arr.length; i++) System.out.println(arr[i]);
}
}
출력:
ArrayList after using the add function:
a
b
c
d
이 방법은 원하는 출력을 제공하지만 배열 요소를 복사하는 루프를 실행하기 때문에 거의 사용되지 않으므로 더 큰 배열을 처리할 때 많은 시간과 메모리가 소요됩니다.