Java에서 객체 유형 가져 오기
-
Java에서
getClass()
를 사용하여 객체 유형 가져 오기 -
Java에서
instanceOf
를 사용하여 객체 유형 가져 오기 - Java에서 수동으로 생성 된 클래스 개체의 유형 가져 오기
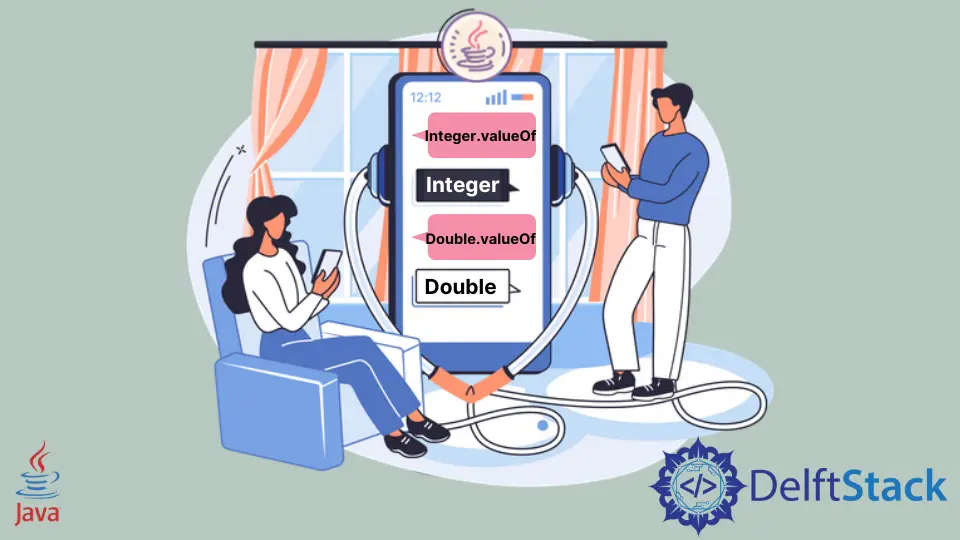
이 기사에서는 Java에서 객체 유형을 얻는 방법을 배웁니다. 개체가 소스에서 가져온 경우 개체 유형을 확인하는 것이 유용합니다. API 또는 액세스 권한이없는 개인 클래스와 같이 객체 유형을 확인할 수없는 곳입니다.
Java에서getClass()
를 사용하여 객체 유형 가져 오기
첫 번째 방법에서는Integer
및String
과 같은 래퍼 클래스의 Object 유형을 확인합니다. 유형을 확인하기 위해var1
및var2
라는 두 개의 객체가 있습니다. Java에있는 모든 객체의 상위 클래스 인Object
클래스의getClass()
메소드를 사용합니다.
if
조건을 사용하여 클래스를 확인합니다. 래퍼 클래스에는 유형을 반환하는 필드 클래스도 포함되어 있으므로var1
및var2
와 일치하는 유형을 확인할 수 있습니다. 아래에서는 세 가지 유형의 두 개체를 모두 확인합니다.
public class ObjectType {
public static void main(String[] args) {
Object var1 = Integer.valueOf("15");
Object var2 = String.valueOf(var1);
if (var1.getClass() == Integer.class) {
System.out.println("var1 is an Integer");
} else if (var1.getClass() == String.class) {
System.out.println("var1 is a String");
} else if (var1.getClass() == Double.class) {
System.out.println("var1 is a Double");
}
if (var2.getClass() == Integer.class) {
System.out.println("var2 is an Integer");
} else if (var2.getClass() == String.class) {
System.out.println("var2 is a String");
} else if (var2.getClass() == Double.class) {
System.out.println("var2 is a Double");
}
}
}
출력:
var1 is an Integer
var2 is a String
Java에서instanceOf
를 사용하여 객체 유형 가져 오기
Java에서 객체 유형을 가져 오는 또 다른 방법은instanceOf
함수를 사용하는 것입니다. 객체의 인스턴스가 주어진 클래스와 일치하면 반환합니다. 이 예에서는Integer
,String
및Double
의 세 가지 유형으로 검사되는var1
및var2
오브젝트가 있습니다. 조건 중 하나라도 충족되면 다른 코드를 실행할 수 있습니다.
var1
이Integer
유형이므로var1 instanceOf Integer
조건이 true가되고var2
가Double
이므로var2 instanceOf Double
이 true가됩니다.
public class ObjectType {
public static void main(String[] args) {
Object var1 = Integer.valueOf("15");
Object var2 = Double.valueOf("10");
if (var1 instanceof Integer) {
System.out.println("var1 is an Integer");
} else if (var1 instanceof String) {
System.out.println("var1 is a String");
} else if (var1 instanceof Double) {
System.out.println("var1 is a Double");
}
if (var2 instanceof Integer) {
System.out.println("var2 is an Integer");
} else if (var2 instanceof String) {
System.out.println("var2 is a String");
} else if (var2 instanceof Double) {
System.out.println("var2 is a Double");
}
}
}
출력:
var1 is an Integer
var2 is a Double
Java에서 수동으로 생성 된 클래스 개체의 유형 가져 오기
래퍼 클래스의 유형을 확인했지만이 예제에서는 수동으로 생성 된 세 가지 클래스의 세 개체 유형을 얻습니다. 세 가지 클래스 :ObjectType2
,ObjectType3
및ObjectType4
를 생성합니다.
ObjectType3
은ObjectType4
를 상속하고ObjectType2
는ObjectType3
을 상속합니다. 이제 우리는 이러한 모든 클래스의 객체 유형을 알고 싶습니다. 세 개의 객체,obj
,obj2
,obj3
이 있습니다. 위의 예제에서 논의한getClass()
와instanceOf
메서드를 모두 사용합니다.
그러나obj2
유형에는 차이가 있습니다. obj2
변수는 클래스가ObjectType3
인 동안ObjectType4
유형을 리턴했습니다. 이는ObjectType3
에서ObjectType4
클래스를 상속하고instanceOf
가 모든 클래스와 하위 클래스를 확인하기 때문에 발생합니다. getClass()
함수는 직접 클래스 만 확인하므로obj
는ObjectType3
을 반환했습니다.
public class ObjectType {
public static void main(String[] args) {
Object obj = new ObjectType2();
Object obj2 = new ObjectType3();
Object obj3 = new ObjectType4();
if (obj.getClass() == ObjectType4.class) {
System.out.println("obj is of type ObjectType4");
} else if (obj.getClass() == ObjectType3.class) {
System.out.println("obj is of type ObjectType3");
} else if (obj.getClass() == ObjectType2.class) {
System.out.println("obj is of type ObjectType2");
}
if (obj2 instanceof ObjectType4) {
System.out.println("obj2 is an instance of ObjectType4");
} else if (obj2 instanceof ObjectType3) {
System.out.println("obj2 is an instance of ObjectType3");
} else if (obj2 instanceof ObjectType2) {
System.out.println("obj2 is an instance of ObjectType2");
}
if (obj3 instanceof ObjectType4) {
System.out.println("obj3 is an instance of ObjectType4");
} else if (obj3 instanceof ObjectType3) {
System.out.println("obj3 is an instance of ObjectType3");
} else if (obj3 instanceof ObjectType2) {
System.out.println("obj3 is an instance of ObjectType2");
}
}
}
class ObjectType2 extends ObjectType3 {
int getAValue3() {
System.out.println(getAValue2());
a = 300;
return a;
}
}
class ObjectType3 extends ObjectType4 {
int getAValue2() {
System.out.println(getAValue1());
a = 200;
return a;
}
}
class ObjectType4 {
int a = 50;
int getAValue1() {
a = 100;
return a;
}
}
출력:
obj is of type ObjectType2
obj2 is an instance of ObjectType4
obj3 is an instance of ObjectType4
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn