Java에서 스레드 ID 가져 오기
-
Java에서
Thread.getId()
를 사용하여 스레드 ID 가져 오기 -
Java에서
Thread.currentThread().getId()
를 사용하여 현재 스레드 풀 ID 가져 오기
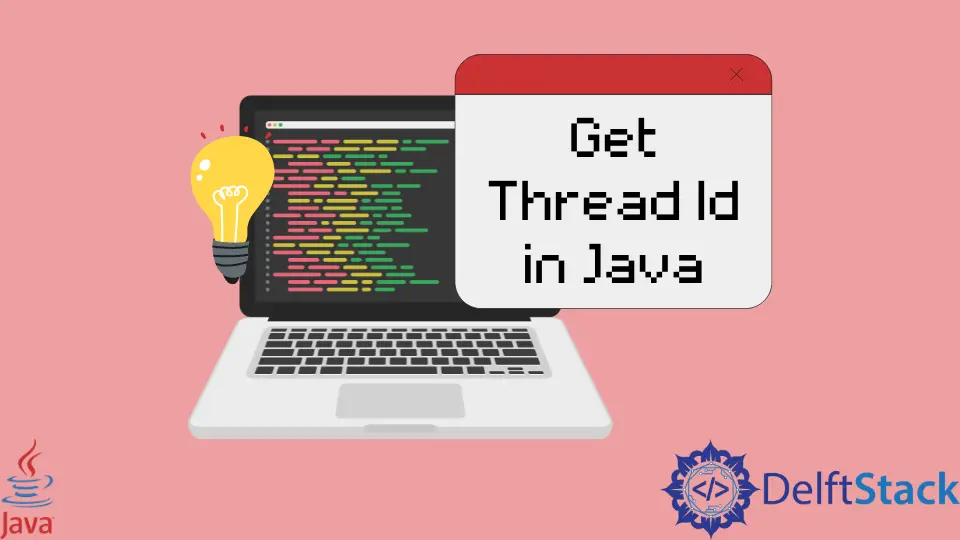
이 튜토리얼에서는 Java에서 스레드 ID를 얻는 방법을 소개합니다. 또한 스레드 풀에서 현재 스레드의 ID를 얻는 방법도 살펴볼 것입니다.
Java에서Thread.getId()
를 사용하여 스레드 ID 가져 오기
이 예제에서는 스레드를 실행하기 위해run()
메서드가 필요하기 때문에Runnable
클래스를 구현하는Task
클래스를 만들었습니다. Task
클래스는 생성자에서 스레드 이름을 가져오고run()
메소드는 실행될 때 콘솔에이를 인쇄합니다.
main()
메서드에서 생성자에 두 개의Task
객체를 만든 다음task1
과task2
을 전달하여 작업을 할당하는 두 개의 스레드 객체를 만듭니다.
스레드를 실행하기 위해thread1
과thread2
를 사용하여start()
메소드를 호출합니다. 마지막으로 스레드가 실행되면 ID를 long
으로 반환하는 thread.getId()
를 사용하여 각 스레드의 ID를 가져올 수 있습니다.
public class GetThreadID {
public static void main(String[] args) {
Task task1 = new Task("Task 1");
Task task2 = new Task("Task 2");
Thread thread1 = new Thread(task1);
Thread thread2 = new Thread(task2);
thread1.start();
thread2.start();
System.out.println("Thread1's ID is: " + thread1.getId());
System.out.println("Thread2's ID is: " + thread2.getId());
}
}
class Task implements Runnable {
private String name;
Task(String name) {
this.name = name;
}
@Override
public void run() {
System.out.println("Executing " + name);
}
}
출력:
Thread1's ID is: 13
Thread2's ID is: 14
Executing Task 2
Executing Task 1
Java에서Thread.currentThread().getId()
를 사용하여 현재 스레드 풀 ID 가져 오기
스레드 풀은 과중한 작업 실행에 유용합니다. 아래 예에서는Executors.newFixedThreadPool(numberOfThreads)
를 사용하여 스레드 풀을 생성합니다. 풀에서 원하는 스레드 수를 지정할 수 있습니다.
Task
클래스는run()
메소드에서 스레드를 실행합니다. 생성자에 전달 된 스레드의 이름을 설정하고 가져 오는 간단한 클래스입니다. 여러 작업을 생성하기 위해 5 개의task
객체가 생성되고 5 개의 스레드가 풀에서 실행되는for
루프를 사용합니다.
우리의 목표는 현재 실행중인 모든 스레드의 ID를 가져 오는 것입니다. 이를 위해 현재 스레드의 ID를 반환하는Thread.currentThread().getId()
를 사용합니다. 출력에서 개별 작업을 실행하는 모든 스레드의 ID를 볼 수 있습니다.
작업이 완료되면threadExecutor.shutdown()
을 사용하여 스레드 풀 실행을 중지해야합니다. !threadExecutor.isTerminated()
는threadExecutor
가 종료 될 때까지 기다리는 데 사용됩니다.
package com.company;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
public class GetThreadID {
public static void main(String[] args) {
int numberOfThreads = 5;
ExecutorService threadExecutor = Executors.newFixedThreadPool(numberOfThreads);
for (int i = 0; i < 5; i++) {
Task task = new Task("Task " + i);
System.out.println("Created Task: " + task.getName());
threadExecutor.execute(task);
}
threadExecutor.shutdown();
while (!threadExecutor.isTerminated()) {
}
System.out.println("All threads have completed their tasks");
}
}
class Task implements Runnable {
private String name;
Task(String name) {
this.name = name;
}
public String getName() {
return name;
}
@Override
public void run() {
System.out.println("Executing: " + name);
System.out.println(name + " is on thread id #" + Thread.currentThread().getId());
}
}
출력:
Created Task: Task 0
Created Task: Task 1
Created Task: Task 2
Created Task: Task 3
Created Task: Task 4
Executing: Task 0
Executing: Task 2
Executing: Task 1
Executing: Task 4
Executing: Task 3
Task 0 is on thread id #13
Task 1 is on thread id #14
Task 4 is on thread id #17
Task 2 is on thread id #15
Task 3 is on thread id #16
All threads have completed their tasks
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn