Java에서 int 배열의 ArrayList 가져오기
-
int 유형의
ArrayList
생성 -
ArrayList
의 인덱스에 정수 요소 추가 -
인덱스를 사용하여
ArrayList
요소에 액세스 - int 배열의 ArrayList
-
ArrayList
에서 int 배열 요소에 액세스 - ArrayList의 ArrayList
- 요약
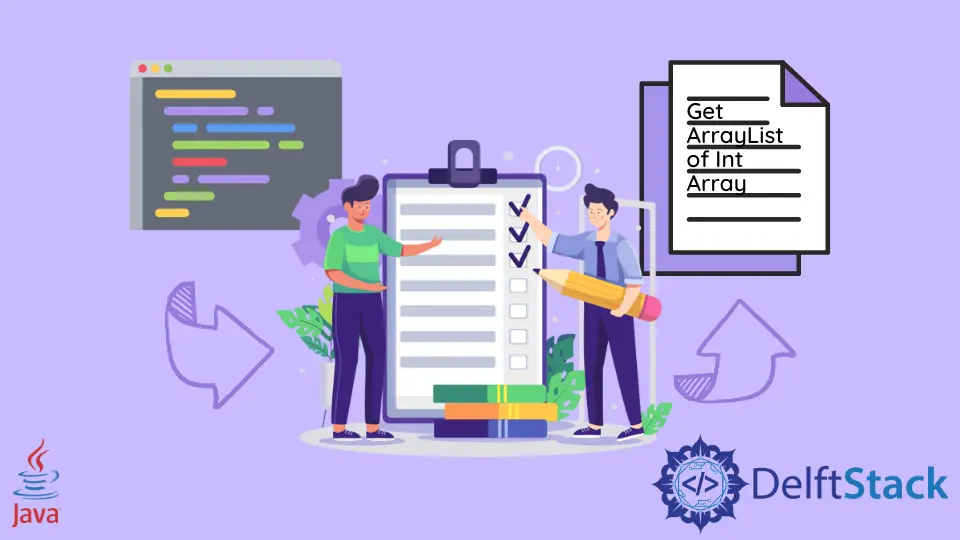
이 튜토리얼은 Java에서 int의 ArrayList
를 얻는 방법을 소개하고 주제를 이해하기 위한 몇 가지 예제 코드를 나열합니다.
ArrayList
는 동적 또는 크기 조정 가능한 배열입니다. Java의 Collection Framework의 일부입니다. ArrayList
는 일반 배열의 고정 크기 문제를 해결하는 데 사용됩니다. 이 자습서에서는 int의 ArrayList
를 만드는 방법을 배웁니다.
int 유형의 ArrayList
생성
ArrayList
또는 다른 컬렉션은 int와 같은 기본 데이터 유형을 저장할 수 없습니다. 아래에 표시된 코드를 작성하면 컴파일 오류가 발생합니다.
public class Demo {
public static void main(String[] args) {
ArrayList<int> arrList;
}
}
출력:
Exception in thread "main" java.lang.Error: Unresolved compilation problem:
Syntax error, insert "Dimensions" to complete ReferenceType
at snippet.Demo.main(Demo.java:7)
대신에 래퍼 클래스를 사용하여 ArrayList
에 프리미티브를 저장합니다. 각 기본 데이터 유형에는 동일한 유형의 개체를 나타내는 해당 래퍼 클래스가 있습니다. int 데이터 유형의 경우 래퍼 클래스를 정수
라고 합니다. 따라서 int의 ArrayList
를 생성하려면 Integer
래퍼 클래스를 해당 유형으로 사용해야 합니다.
import java.util.ArrayList;
public class Demo {
public static void main(String[] args) {
ArrayList<Integer> arrList = new ArrayList<Integer>();
}
}
이제 클래스의 add()
메서드를 사용하여 ArrayList
에 정수를 추가할 수 있습니다.
import java.util.ArrayList;
public class Demo {
public static void main(String[] args) {
ArrayList<Integer> arrList = new ArrayList<Integer>();
arrList.add(5);
arrList.add(7);
arrList.add(12);
}
}
ArrayList
는 일반 배열과 마찬가지로 0부터 시작하는 인덱싱을 따릅니다. 우리는 add()
메소드에서 객체를 추가할 인덱스를 지정할 수 있습니다. 해당 인덱스에 있는 요소와 그 오른쪽에 있는 모든 요소는 오른쪽으로 한 자리 이동합니다.
ArrayList
의 인덱스에 정수 요소 추가
import java.util.ArrayList;
public class Demo {
public static void main(String[] args) {
ArrayList<Integer> arrList = new ArrayList<Integer>();
arrList.add(5);
arrList.add(7);
arrList.add(12);
arrList.add(1, 199); // Inserting 199 at index 1.
}
}
인덱스를 사용하여 ArrayList
요소에 액세스
인덱스를 사용하여 개별 ArrayList
항목을 가져올 수 있습니다. 인덱스 값을 get()
메서드에 전달하여 필요한 요소를 가져옵니다.
import java.util.ArrayList;
public class Demo {
public static void main(String[] args) {
ArrayList<Integer> arrList = new ArrayList<Integer>();
arrList.add(5);
arrList.add(7);
arrList.add(12);
arrList.add(1, 199); // Inserting 199 at index 1.
System.out.println("Element at index 1: " + arrList.get(1));
System.out.println("Element at index 2: " + arrList.get(2));
}
}
출력:
Element at index 1: 199
Element at index 2: 7
단일 인쇄 문을 사용하여 전체 ArrayList
를 인쇄할 수도 있습니다.
import java.util.ArrayList;
public class Demo {
public static void main(String[] args) {
ArrayList<Integer> arrList = new ArrayList<Integer>();
arrList.add(5);
arrList.add(7);
arrList.add(12);
System.out.println("Printing the ArrayList: " + arrList);
arrList.add(1, 199); // Inserting 199 at index 1.
System.out.println("Printing the ArrayList: " + arrList);
}
}
출력:
Printing the ArrayList: [5, 7, 12]
Printing the ArrayList: [5, 199, 7, 12]
int 배열의 ArrayList
각 요소 자체가 배열인 ArrayList
를 만들 수 있습니다. 데이터 유형과 대괄호를 사용하여 새 배열을 만듭니다.
마찬가지로 int[]
를 사용하여 ArrayList
의 유형을 정의했습니다. ArrayList
유형으로 int와 같은 프리미티브를 사용할 수 없지만 int[]는 사용할 수 있습니다. Java의 배열은 기본 요소가 아니라 개체이기 때문입니다. 그리고 ArrayList
는 모든 객체 유형(이 경우 배열)으로 생성할 수 있습니다.
ArrayList<int[]> arrList = new ArrayList<int[]>();
위에서 논의한 모든 기본 작업을 수행할 수 있습니다. 어레이를 콘솔에 올바르게 인쇄하려면 Arrays
의 toString()
메서드를 사용해야 합니다.
import java.util.ArrayList;
import java.util.Arrays;
public class Demo {
public static void main(String[] args) {
ArrayList<int[]> arrList = new ArrayList<int[]>();
int[] arr1 = {2, 4, 6};
int[] arr2 = {3, 6, 9};
int[] arr3 = {5, 10, 15};
// Adding int arrays to the ArrayList
arrList.add(arr1);
arrList.add(arr2);
arrList.add(arr3);
// Fetching the array from the List
int[] arrAtIdx1 = arrList.get(1);
// Printing the fetched array using the toString() method of Arrays
System.out.println("The Second array in the List is: " + Arrays.toString(arrAtIdx1));
}
}
출력:
The Second array in the List is: [3, 6, 9]
ArrayList
에서 int 배열 요소에 액세스
ArrayList
에 있는 int 배열의 개별 요소에 액세스할 수도 있습니다. 이를 위해 배열 인덱스를 사용할 것입니다. 예를 들어, 세 번째 배열의 두 번째 요소에 액세스하려면 다음 코드를 사용합니다.
import java.util.ArrayList;
public class Demo {
public static void main(String[] args) {
ArrayList<int[]> arrList = new ArrayList<int[]>();
int[] arr1 = {2, 4, 6};
int[] arr2 = {3, 6, 9};
int[] arr3 = {5, 10, 15};
// Adding int arrays to the ArrayList
arrList.add(arr1);
arrList.add(arr2);
arrList.add(arr3);
// Fetching the second element of the third array
int[] thirdArr = arrList.get(2);
int secondElement = thirdArr[1];
System.out.println("Second Element of the Third Array is: " + secondElement);
}
}
출력:
Second Element of the Third Array is: 10
그러나 어레이의 전체 ArrayList
를 인쇄하려면 추가 코드가 필요합니다.
import java.util.ArrayList;
import java.util.Arrays;
public class Demo {
public static void main(String[] args) {
ArrayList<int[]> arrList = new ArrayList<int[]>();
int[] arr1 = {2, 4, 6};
int[] arr2 = {3, 6, 9};
int[] arr3 = {5, 10, 15};
// Adding int arrays to the ArrayList
arrList.add(arr1);
arrList.add(arr2);
arrList.add(arr3);
for (int i = 0; i < arrList.size(); i++) {
int[] currArr = arrList.get(i);
System.out.println("Array at index " + i + " is: " + Arrays.toString(currArr));
}
}
}
출력:
Array at index 0 is: [2, 4, 6]
Array at index 1 is: [3, 6, 9]
Array at index 2 is: [5, 10, 15]
ArrayList의 ArrayList
위에서 논의한 바와 같이 배열은 고정 길이이지만 ArrayLists
는 동적입니다. int 배열의 ArrayList
를 만드는 대신 Integer
ArrayLists
의 ArrayList
를 만들 수 있습니다. 이렇게 하면 배열의 공간 부족에 대해 걱정할 필요가 없습니다.
ArrayList<ArrayList<Integer> > arrListOfarrLists = new ArrayList<ArrayList<Integer> >();
이전과 마찬가지로 add()
메소드와 get()
메소드를 사용할 수 있습니다. 그러나 각 ArrayList
요소를 인쇄하려면 루프가 필요합니다.
import java.util.ArrayList;
public class Demo {
public static void main(String[] args) {
ArrayList<ArrayList<Integer> > arrListOfarrLists = new ArrayList<ArrayList<Integer> >();
// Creating individual ArrayLists
ArrayList<Integer> arrList1 = new ArrayList<>();
arrList1.add(2);
arrList1.add(4);
arrList1.add(6);
ArrayList<Integer> arrList2 = new ArrayList<>();
arrList2.add(3);
arrList2.add(6);
arrList2.add(9);
ArrayList<Integer> arrList3 = new ArrayList<>();
arrList3.add(5);
arrList3.add(10);
arrList3.add(15);
// Adding ArrayLists to the ArrayList
arrListOfarrLists.add(arrList1);
arrListOfarrLists.add(arrList2);
arrListOfarrLists.add(arrList3);
// Fetching ArrayList
ArrayList<Integer> listAtIdx1 = arrListOfarrLists.get(1);
System.out.println("ArrayList present at index 1 is: " + listAtIdx1 + "\n");
// Printing the entire ArrayList
for (int i = 0; i < arrListOfarrLists.size(); i++)
System.out.println("ArrayList at index " + i + " is " + arrListOfarrLists.get(i));
}
}
출력:
ArrayList present at index 1 is: [3, 6, 9]
ArrayList at index 0 is [2, 4, 6]
ArrayList at index 1 is [3, 6, 9]
ArrayList at index 2 is [5, 10, 15]
ArrayList
의 개별 요소에 액세스하려면 get()
메소드를 두 번 사용하십시오. 예를 들어 세 번째 ArrayList
의 두 번째 요소를 원하면 다음을 사용합니다.
import java.util.ArrayList;
public class Demo {
public static void main(String[] args) {
ArrayList<ArrayList<Integer> > arrListOfarrLists = new ArrayList<ArrayList<Integer> >();
// Creating individual ArrayLists
ArrayList<Integer> arrList1 = new ArrayList<>();
arrList1.add(2);
arrList1.add(4);
arrList1.add(6);
ArrayList<Integer> arrList2 = new ArrayList<>();
arrList2.add(3);
arrList2.add(6);
arrList2.add(9);
ArrayList<Integer> arrList3 = new ArrayList<>();
arrList3.add(5);
arrList3.add(10);
arrList3.add(15);
// Adding ArrayLists to the ArrayList
arrListOfarrLists.add(arrList1);
arrListOfarrLists.add(arrList2);
arrListOfarrLists.add(arrList3);
// Fetching second element of the third ArrayList
ArrayList<Integer> thirdList = arrListOfarrLists.get(2);
int secondElement = thirdList.get(1);
System.out.print("second element of the third ArrayList is: " + secondElement);
}
}
출력:
second element of the third ArrayList is: 10
요약
ArrayList
는 아마도 Java에서 가장 일반적으로 사용되는 컬렉션일 것입니다. 동일한 유형의 요소를 저장하는 데 사용되는 간단한 데이터 구조입니다. int와 같은 기본 유형의 ArrayList
를 만들 수 없습니다. 이러한 프리미티브의 래퍼 클래스를 사용해야 합니다. ArrayList
클래스는 목록에서 요소를 추가하고 가져오는 편리한 메소드를 제공합니다. 배열의 ArrayList
또는 ArrayLists
의 ArrayList
를 만들 수도 있습니다. 그들은 주로 2D 매트릭스 형식 또는 표 형식으로 데이터를 나타내는 데 사용됩니다. 크기를 제한하지 않으므로 ArrayLists
의 ArrayList
를 사용하는 것이 좋습니다.