파일을 만들고 Java로 데이터를 쓰는 방법
Hassan Saeed
2023년10월12일
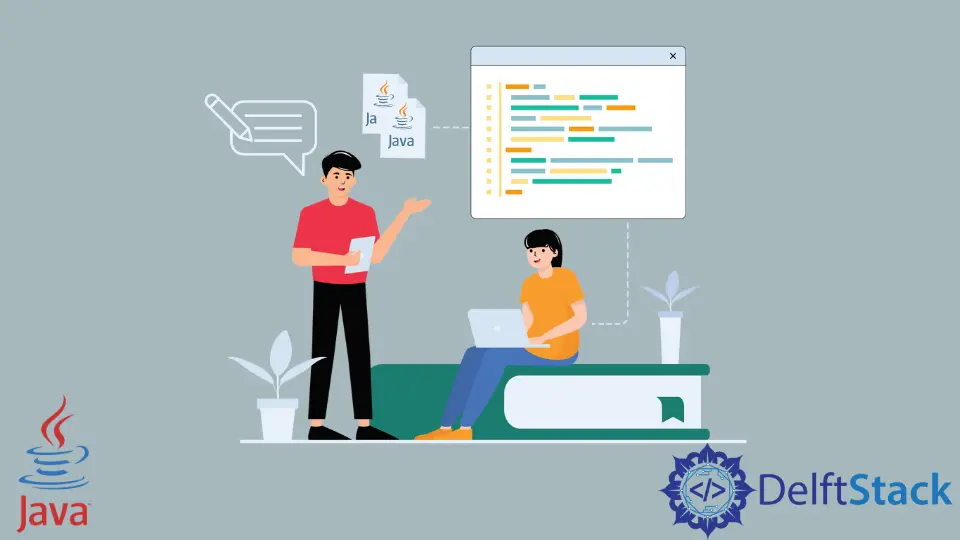
이 자습서에서는 텍스트 파일을 만들고 Java로 데이터를 쓰는 방법에 대해 설명합니다.
Java에는 파일을 만드는 여러 방법이 있습니다. 기억해야 할 한 가지는 거의 모든 파일 생성 방법에try-catch
블록이 필요하므로 모든 IO 예외가 정상적으로 처리됩니다. 그것을 사용하는 것을 잊지 마십시오.
Java로 파일을 만드는PrintWriter
Java의PrintWriter
를 사용하면 지정된 인코딩으로 새 파일을 만들 수 있습니다. 여기에 예가 있습니다.
import java.io.*;
public class Main {
public static void main(String[] args) {
String fileName = "my-file.txt";
String encoding = "UTF-8";
try {
PrintWriter writer = new PrintWriter(fileName, encoding);
writer.println("The first line");
writer.println("The second line");
writer.close();
} catch (IOException e) {
System.out.println("An error occurred.");
e.printStackTrace();
}
}
}
Java로 파일을 만드는파일
java.nio.file
을 사용하여 파일을 생성하고 데이터를 쓸 수도 있습니다. 아래 예는이 접근 방식을 보여줍니다.
import java.io.*;
import java.nio.charset.*;
import java.nio.file.*;
import java.util.*;
public class Main {
public static void main(String[] args) {
String fileName = "my-file.txt";
try {
List<String> lines = Arrays.asList("The first line", "The second line");
Path file = Paths.get(fileName);
Files.write(file, lines, StandardCharsets.UTF_8);
} catch (IOException e) {
System.out.println("An error occurred.");
e.printStackTrace();
}
}
}
이 예제에서는ArrayList
의 모든 요소가 출력 파일의 새 줄에 기록되도록ArrayList
의 데이터를 파일에 씁니다. 출력 파일은 다음과 같습니다.
> The first line
> The second line
Java로 파일을 만드는BufferedWriter
아래 예제는BufferedWriter
를 사용하여 파일을 만들고 쓰는 방법을 보여줍니다.
import java.io.*;
import java.nio.charset.*;
import java.util.*;
public class Main {
public static void main(String[] args) {
Charset utf8 = StandardCharsets.UTF_8;
List<String> list = Arrays.asList("This is the first line", "This is the second line");
String content = "This is my content";
try {
// File name with path
String fileName = "my-file.txt";
File myFile = new File(fileName);
FileOutputStream fos = new FileOutputStream(myFile);
OutputStreamWriter osw = new OutputStreamWriter(fos);
Writer writer = new BufferedWriter(osw);
// Write data using a String variable
writer.write(content + "\n");
// Write data from an ArrayList
for (String s : list) {
writer.write(s + "\n");
}
} catch (IOException e) {
System.out.println("An error occurred.");
e.printStackTrace();
}
}
}
출력 파일은 다음과 같습니다.
> This is my content
> This is the first line
> This is the second line
Java로 파일을 만드는FileWriter
새 파일을 만들고 Java로 작성하는 또 다른 일반적인 방법은FileWriter
를 사용하는 것입니다.
import java.io.*;
public class Main {
public static void main(String[] args) {
try {
FileWriter myWriter = new FileWriter("my-file.txt");
myWriter.write("We can also create a file and write to it using FileWriter");
myWriter.close();
} catch (IOException e) {
System.out.println("An error occurred.");
e.printStackTrace();
}
}
}
출력 파일은 다음과 같습니다.
> We can also create a file and write to it using FileWriter