상속에서 Java 생성자의 실행
- 상속에서 Java 생성자의 실행
- 상속에서 기본 Java 생성자의 실행
- 상위 클래스에 기본 생성자 및 매개변수화된 생성자가 있는 경우 상속에서 Java 생성자 실행
-
super
를 사용하여 상위 클래스와 모든 하위 클래스의 매개변수화된 생성자를 호출합니다
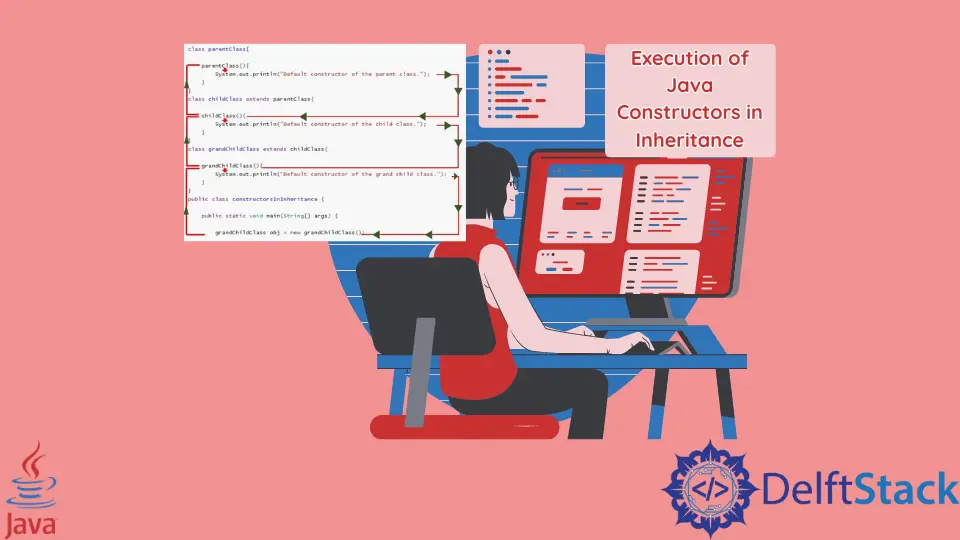
오늘은 상속에서 자바 생성자의 실행에 대해 알아보겠습니다. 파생 클래스(자식 클래스 및 하위 클래스라고도 함)에서 기본 및 매개 변수화된 생성자의 코드 예제를 볼 수 있습니다.
상속에서 Java 생성자의 실행
이 기사를 계속하려면 상속에 대한 충분한 지식이 필요합니다. 이 튜토리얼을 읽고 있다면 Java 상속에 대해 확실히 이해하고 있다고 가정합니다.
extens
키워드를 사용하여 상위 클래스(기본 클래스 또는 수퍼 클래스라고도 함)를 확장하는 동안 Java 생성자의 실행 프로세스에 대해 알아보겠습니다.
상속에서 기본 Java 생성자의 실행
예제 코드:
class parentClass {
parentClass() {
System.out.println("Default constructor of the parent class.");
}
}
class childClass extends parentClass {
childClass() {
System.out.println("Default constructor of the child class.");
}
}
class grandChildClass extends childClass {
grandChildClass() {
System.out.println("Default constructor of the grand child class.");
}
}
public class constructorsInInheritance {
public static void main(String[] args) {
grandChildClass obj = new grandChildClass();
}
}
출력:
Default constructor of the parent class.
Default constructor of the child class.
Default constructor of the grand child class.
여기에 grandChildClass
객체를 생성하는 constructorsInInheritance
라는 테스트 클래스가 하나 있습니다.
parentClass
, childClass
, grandChildClass
라는 세 개의 다른 클래스가 있습니다. 여기서 grandChildClass
는 childClass
를 상속하고 childClass
는 parentClass
를 확장합니다.
여기에서 parentClass
기본 생성자는 childClass
생성자에 의해 자동으로 호출됩니다. 자식 클래스를 인스턴스화할 때마다 부모 클래스의 생성자가 자동으로 실행되고 그 다음에 자식 클래스의 생성자가 실행됩니다.
위에 주어진 출력을 관찰하십시오. 여전히 혼란스럽다면 다음 시각적 설명을 참조하십시오.
main
메소드에서 childClass
의 객체를 생성하면 어떻게 될까요? 이제 기본 생성자가 어떻게 실행됩니까?
parentClass
의 생성자가 먼저 실행되고 childClass
의 생성자가 다음 결과를 생성합니다.
출력:
Default constructor of the parent class.
Default constructor of the child class.
상위 클래스에 기본 생성자 및 매개변수화된 생성자가 있는 경우 상속에서 Java 생성자 실행
예제 코드:
class parentClass {
parentClass() {
System.out.println("Default constructor of the parent class.");
}
parentClass(String name) {
System.out.println("Hi " + name + "! It's a parameterized constructor of the parent class");
}
}
class childClass extends parentClass {
childClass() {
System.out.println("Default constructor of the child class.");
}
}
class grandChildClass extends childClass {
grandChildClass() {
System.out.println("Default constructor of the grand child class.");
}
}
public class constructorsInInheritance {
public static void main(String[] args) {
grandChildClass obj = new grandChildClass();
}
}
출력:
Default constructor of the parent class.
Default constructor of the child class.
Default constructor of the grand child class.
여기 parentClass
에 매개변수화된 생성자가 있습니다. 그러나 기본 생성자는 main
메소드에서 grandChildClass()
기본 생성자를 호출하고 상위 클래스 기본 생성자를 추가로 호출하기 때문에 계속 호출됩니다.
childClass
와 grandChildClass
에 매개변수화된 생성자를 작성해 보겠습니다. 그런 다음 main
함수에서 grandChildClass
의 매개변수화된 생성자를 호출합니다.
기본 생성자이든 매개변수화 생성자이든 상관없이 어떤 생성자가 호출되는지 관찰하십시오.
예제 코드:
class parentClass {
parentClass() {
System.out.println("Default constructor of the parent class.");
}
parentClass(String name) {
System.out.println("Hi " + name + "! It's a parameterized constructor of the parent class");
}
}
class childClass extends parentClass {
childClass() {
System.out.println("Default constructor of the child class.");
}
childClass(String name) {
System.out.println("Hi " + name + "! It's a parameterized constructor of the child class");
}
}
class grandChildClass extends childClass {
grandChildClass() {
System.out.println("Default constructor of the grand child class.");
}
grandChildClass(String name) {
System.out.println(
"Hi " + name + "! It's a parameterized constructor of the grand child class");
}
}
public class constructorsInInheritance {
public static void main(String[] args) {
grandChildClass obj = new grandChildClass("Mehvish");
}
}
출력:
Default constructor of the parent class.
Default constructor of the child class.
Hi Mehvish! It's a parameterized constructor of the grand child class
위의 코드는 grandChildClass
의 매개변수화된 생성자만 호출합니다. super()
를 사용하여 parentClass
, childClass
및 grandChildClass
의 매개변수화된 생성자를 호출합니다.
부모 클래스 생성자 호출은 자식 클래스 생성자의 첫 번째 줄에 있어야 한다는 것을 기억하십시오.
super
를 사용하여 상위 클래스와 모든 하위 클래스의 매개변수화된 생성자를 호출합니다
예제 코드:
class parentClass {
parentClass() {
System.out.println("Default constructor of the parent class.");
}
parentClass(String name) {
System.out.println("Hi " + name + "! It's a parameterized constructor of the parent class");
}
}
class childClass extends parentClass {
childClass() {
System.out.println("Default constructor of the child class.");
}
childClass(String name) {
super(name);
System.out.println("Hi " + name + "! It's a parameterized constructor of the child class");
}
}
class grandChildClass extends childClass {
grandChildClass() {
System.out.println("Default constructor of the grand child class.");
}
grandChildClass(String name) {
super(name);
System.out.println(
"Hi " + name + "! It's a parameterized constructor of the grand child class");
}
}
public class constructorsInInheritance {
public static void main(String[] args) {
grandChildClass obj = new grandChildClass("Mehvish");
}
}
출력:
Hi Mehvish! It's a parameterized constructor of the parent class
Hi Mehvish! It's a parameterized constructor of the child class
Hi Mehvish! It's a parameterized constructor of the grand child class
super
키워드를 사용하여 매개변수화된 상위 클래스 생성자를 호출했습니다. 상위 클래스(수퍼 클래스 또는 기본 클래스)를 참조합니다.
우리는 그것을 사용하여 부모 클래스 생성자에 액세스하고 부모 클래스 메서드를 호출했습니다.
super
는 부모 및 자식 클래스의 정확한 이름을 가진 메서드에 매우 유용합니다.