Java에서 OutputStream을 문자열로 변환
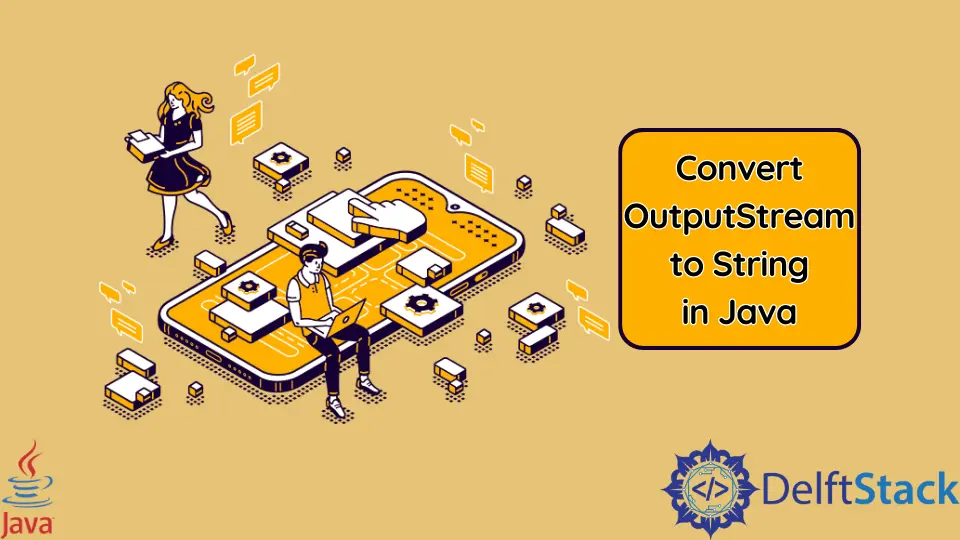
이 튜토리얼은 Java에서 OutputStream
을 String
으로 변환하는 방법을 보여줍니다.
Java에서 OutputStream
을 String
으로 변환
OutputStream
은 FileOutputStream
, ByteArrayOutputStream
, ObjectOutputStream
등과 같은 하위 클래스를 포함하는 Java의 io
패키지의 추상 클래스입니다.
반면 문자열
은 단순한 문자 집합입니다. 따라서 OutputStream
을 String
으로 변환하는 것은 쉽습니다. 이를 위해 java.io.ByteArrayOutputStream.toString()
메서드를 사용할 수 있습니다.
또는 OutputStream
을 String
으로 캐스팅할 수 있습니다. OutputStream
을 String
으로 변환하는 코드 예제를 작성해 봅시다.
예 1:
이 코드에는 5단계가 포함됩니다. 먼저 빈 String
을 초기화합니다. 둘째, String
의 ASCII
값으로 배열을 만듭니다. 세 번째 단계에서 OutputStream
개체를 만듭니다.
넷째, write
메서드를 사용하여 Byte
배열을 개체에 복사합니다. 마지막으로 최종 문자열을 인쇄합니다.
package delftstack;
import java.io.*;
class Example1 {
public static void main(String[] args) throws IOException {
// Initialize empty string and byte array
String DemoString = "";
byte[] ByteArray = {72, 101, 108, 108, 111, 33, 32, 84, 104, 105, 115, 32, 105, 115, 32, 100,
101, 108, 102, 116, 115, 116, 97, 99, 107, 46, 99, 111, 109, 46, 46};
// create ByteArrayOutputStream
ByteArrayOutputStream Output_Stream = new ByteArrayOutputStream();
// Now write byte array to the output stream
Output_Stream.write(ByteArray);
// convert buffers using the toString method into String type
DemoString = Output_Stream.toString();
// print The String
System.out.println("The converted String is: " + DemoString);
}
}
위의 코드는 ASCII
값이 있는 ByteArrayOutputStream
을 String
으로 변환합니다. 아래 출력을 참조하십시오.
The converted String is: Hello! This is delftstack.com..
예 2:
여기서 우리는 네 단계에 따라 코드를 작성할 수 있습니다. 먼저 ByteArrayOutputStream
객체를 만듭니다. 둘째, 빈 변수를 String
변수로 초기화합니다.
셋째, write
메서드를 사용하여 String
을 OutputStream
에 복사합니다. 마지막으로 다음과 같은 방법으로 최종 String
을 초기화합니다.
String Final_String = new String(Output_Stream.toByteArray());
다음 코드를 작성하여 배워봅시다.
package delftstack;
import java.io.*;
class Example2 {
public static void main(String[] args) throws IOException {
// declare ByteArrayOutputStream
ByteArrayOutputStream Output_Stream = new ByteArrayOutputStream();
// Initiale string
String DemoString = "Hello! This is delftstack.com..";
// write the bytes to the output stream
Output_Stream.write(DemoString.getBytes());
// convert the stream to byte array and use typecasting
String Final_String = new String(Output_Stream.toByteArray());
// print the strings
System.out.println("The Demo String is: " + DemoString);
System.out.println("The final String is: " + Final_String);
}
}
위의 코드는 ByteArrayOutputStream
을 String
으로 변환합니다. 아래 예를 참조하십시오.
The Demo String is: Hello! This is delftstack.com..
The final String is: Hello! This is delftstack.com..
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook