C#에서 빈 배열 초기화
Fil Zjazel Romaeus Villegas
2023년10월12일
Csharp
Csharp Array
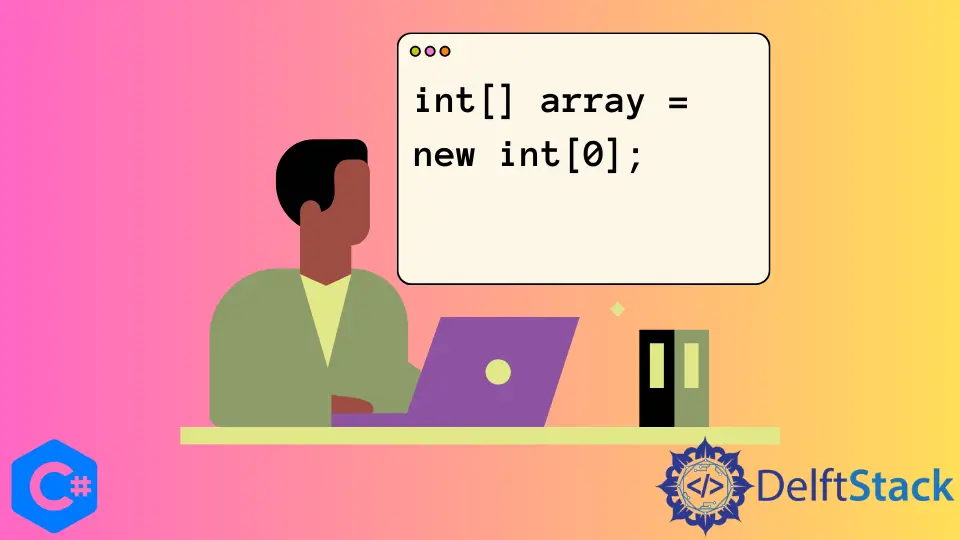
이 자습서는 알려진 크기 또는 빈 배열로 배열을 초기화하는 방법을 보여줍니다.
배열은 길이가 고정된 값 모음을 나타냅니다. 초기화 시 크기를 설정한 후에는 더 이상 배열을 추가하거나 축소할 수 없습니다. 더 이상 배열의 크기를 변경할 수 없지만 배열 내의 항목 값은 변경할 수 있습니다. 배열은 많은 양의 데이터를 구성할 때 유용합니다.
배열을 초기화하려면 다음 예 중 하나를 사용할 수 있습니다.
// An array with a size of 5, all values are set to the default value. In this case, a 0 because its
// an integer
int[] array = new int[5];
// An array with all values set. When initializing the array in this way, there is no need to
// specify the size
int[] array_2 = new int[] { 10, 9, 8, 7, 6 };
배열은 길이가 고정되어 있으므로 저장하려는 컬렉션 크기를 모르는 경우 필요한 만큼 항목을 추가할 수 있는 List<T>
와 같은 다른 옵션을 고려할 수 있습니다. 모든 항목을 추가한 후 ToArray()
함수를 사용하여 목록을 배열로 변환할 수 있습니다.
List<int> list = new List<int>();
int[] array = list.ToArray();
그러나 정말로 빈 배열이 필요한 경우 배열의 크기를 0으로 설정할 수 있습니다.
int[] array = new int[0];
예시:
using System;
namespace StringArray_Example {
class Program {
static void Main(string[] args) {
// Initialize an empty array
int[] empty_array = new int[0];
// Join the values of the array to a single string separated by a comma
string item_str = string.Join(", ", empty_array);
// Print the length of the array
Console.WriteLine("The array has a length of " + empty_array.Length.ToString());
// Print the values of the array
Console.WriteLine("Items: " + item_str);
try {
// Attempt to add a new item to the empty array
empty_array[0] = 1;
} catch (Exception ex) {
Console.WriteLine("You cannot add new items to an array!");
Console.WriteLine("Exception: " + ex.ToString());
}
Console.ReadLine();
}
}
}
위의 예에서 크기를 0으로 설정하여 빈 정수 배열을 선언했습니다. 값과 길이를 콘솔에 출력하여 실제로 비어 있음을 입증한 후 배열에 새 값을 추가하려고 했습니다. 어레이의 크기를 수정할 수 없기 때문에 불가피하게 실패했습니다. 따라서 인덱스가 어레이 범위를 벗어났습니다.
오류가 발생했습니다.
출력:
The array has a length of 0
Items:
You cannot add new items to an array!
Exception: System.IndexOutOfRangeException: Index was outside the bounds of the array.
튜토리얼이 마음에 드시나요? DelftStack을 구독하세요 YouTube에서 저희가 더 많은 고품질 비디오 가이드를 제작할 수 있도록 지원해주세요. 구독하다