C#에서 리플렉션을 사용하여 속성 값 가져오기
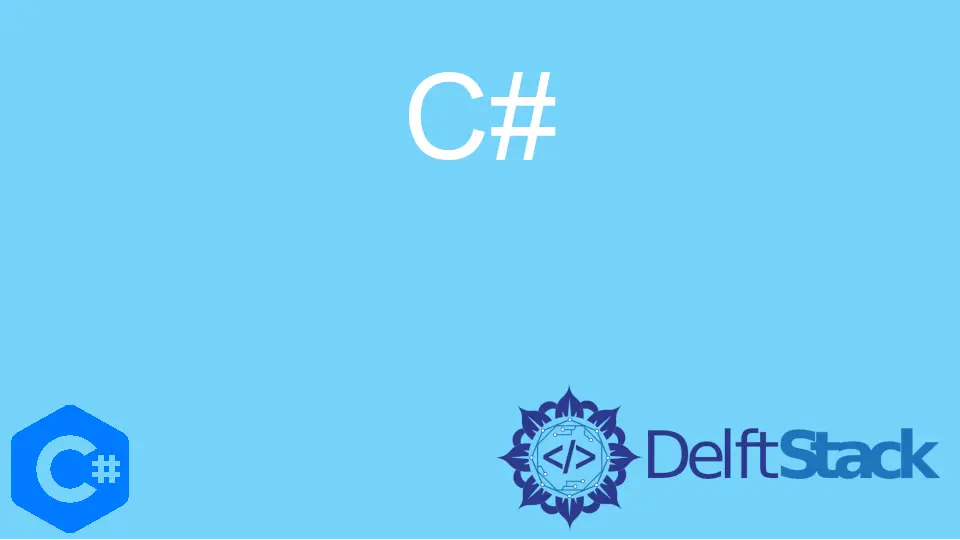
이 문서에서는 C#에서 System.Reflection
네임스페이스를 사용하는 방법을 자세히 설명합니다. Reflection
네임스페이스를 사용하여 샘플 프로젝트를 살펴보고 속성 값을 가져옵니다.
C#
의 System.Reflection
C#의 System.Reflection
네임스페이스는 코드의 매개 변수, 어셈블리, 메서드, 모듈 및 기타 요소에 대한 정보를 가져오는 데 사용됩니다. 이 네임스페이스를 프로그램으로 가져오면 모든 클래스 유형, 매개변수 값, 메서드 등을 가져올 수 있습니다.
System.Reflection
기능을 사용하여 개체의 속성 값을 가져오는 기술을 시연합니다.
C#
에서 리플렉션을 사용하여 속성 값 가져오기
Visual Studio에서 C# 프로젝트를 만듭니다. 먼저 Reflection
네임스페이스를 프로그램으로 가져옵니다.
using System.Reflection;
System
네임스페이스에는 개체 유형을 반환하는 Type
클래스가 포함되어 있습니다.
Type type = _obj.GetType();
Reflection
네임스페이스에는 유형의 속성을 가져오는 PropertyInfo
라는 클래스가 포함되어 있습니다. Type
클래스에는 각각 속성과 클래스 속성의 배열을 반환하는 메서드 GetProperty()
및 GetProperties()
가 있습니다.
PropertyInfo[] properties = type.GetProperties();
PropertyInfo
클래스에는 속성의 이름과 여기에 할당된 값을 각각 반환하는 Name
및 GetValue()
라는 멤버 특성 및 메서드가 포함되어 있습니다.
Console.WriteLine(p.Name + " : " + p.GetValue(_obj));
다음은 예제 프로젝트의 전체 코드 스니펫입니다.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Reflection;
namespace GettingPropertyValue {
public class Book {
private String bookTitle;
private int numberOfPages;
public Book(String title, int pages) {
bookTitle = title;
numberOfPages = pages;
}
public String Title {
get { return bookTitle; }
}
public int Pages {
get { return numberOfPages; }
set { numberOfPages = value; }
}
}
public class MyGetValue {
public static void Main() {
Console.WriteLine("Getting all properties...");
Book book = new Book("Get Property Value in C#", 198);
GetPropertyValues(book);
Console.WriteLine("\n\nGetting value of a single property...");
GetPropertyValue(book, "Pages");
Console.ReadKey();
}
private static void GetPropertyValues(Object _obj) {
// returns the type of _obj
Type type = _obj.GetType();
Console.WriteLine("Type of Object is: " + type.Name);
// create an array of properties
PropertyInfo[] properties = type.GetProperties();
Console.WriteLine("Properties (Count = " + properties.Length + "):");
// print each property name and value
foreach (var p in properties)
if (p.GetIndexParameters().Length == 0)
Console.WriteLine(p.Name + "(" + p.PropertyType.Name + ") : " + p.GetValue(_obj));
else
Console.WriteLine(" {0} ({1}): <Indexed>", p.Name, p.PropertyType.Name);
}
// method to get a single property value from a string
private static void GetPropertyValue(Object _obj, string property) {
// prints property name
Console.WriteLine("Property : " + _obj.GetType().GetProperty(property).Name);
// prints property value
Console.WriteLine("Value : " + _obj.GetType().GetProperty(property).GetValue(_obj));
}
}
}
우리는 두 가지 속성(제목과 페이지 수)을 가진 Book
이라는 클래스를 만들었습니다. 다른 클래스인 MyGetValue
에 두 개의 메서드(예: GetPropertyValue
및 GetPropertyValues
)를 만들었습니다.
GetProjectValue(Object _obj, string property)
는 개체와 문자열 또는 속성을 매개 변수로 사용하고 속성 이름과 값을 출력으로 인쇄합니다.
반면 GetPropertyValues(Object _obj)
메서드는 개체를 매개 변수로 사용합니다. 먼저 객체의 유형을 결정하고 해당 객체의 값과 함께 모든 속성을 가져옵니다.
코드 조각의 출력은 다음과 같습니다.
Getting all properties...
Type of Object is: Book
Properties (Count = 2):
Title(String) : Get Property Value in C#
Pages(Int32) : 198
Getting value of a single property...
Property : Pages
Value : 198
괴짜라면 System.Reflection
C#을 방문하여 C#의 Reflections에 대한 자세한 내용을 참조할 수 있습니다.