C#에서 클래스 확장
Muhammad Zeeshan
2023년10월12일
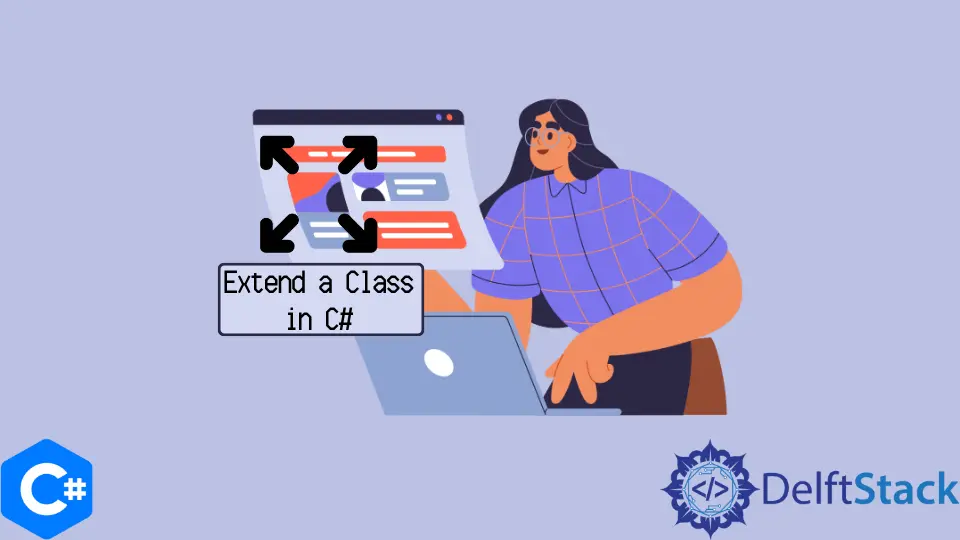
이 자습서에서는 C# 프로그래밍 언어를 사용하여 클래스를 확장하는 방법을 알려줍니다.
상속을 사용하여 C#
에서 클래스 확장
객체 지향 프로그래밍(OOP
)으로 작업할 때 상속은 계층 수준과 연결됩니다. 파생
클래스를 기본
클래스로 변환하는 것이 가능하지만 그 반대는 불가능합니다.
기본
클래스에서 파생
클래스로 변환하는 것은 불가능합니다. 더 잘 이해하려면 다음 예를 살펴보십시오.
다음 예제에서는 한 번에 하나씩 새 개체에 속성을 추가한 다음 새 개체를 사용하여 이전 속성에서 단일 속성을 확장합니다.
-
시작하려면 다음 라이브러리를 가져옵니다.
using System; using System.Collections.Generic; using System.Linq; using System.Text.RegularExpressions;
-
Firstname
및Lastname
속성이 있는Person
클래스를 만듭니다.public class Person { public string Firstname { get; set; } public string Lastname { get; set; } public Person(string fname, string lname) { Firstname = fname; Lastname = lname; } }
-
생성자 오버로딩은 이제
Person
에서Student
를 빌드하는 데 사용됩니다.public class Student : Person { public Student(Person person, string code) : base(person.Firstname, person.Lastname) { this.code = code; } public Student(Person person) : base(person.Firstname, person.Lastname) {} public string code { get; set; } }
-
Main()
메서드에서Person
개체를 만들고 아래와 같이 데이터를 채웁니다.Person person = new Person("Muhammad Zeeshan", "Khan");
-
그런 다음
s1
및s2
라는 두 개의Student
개체를 만들고person
개체 및code
를 매개 변수로 전달합니다.Student s1 = new Student(person, "3229"); Student s2 = new Student(person, "3227");
-
마지막으로
Person
속성을 포함하는Student
개체를 인쇄합니다.Console.WriteLine(s1.code); Console.WriteLine(s2.code);
-
완전한 소스 코드.
using System; using System.Collections.Generic; using System.Linq; using System.Text.RegularExpressions; public class Person { public string Firstname { get; set; } public string Lastname { get; set; } public Person(string fname, string lname) { Firstname = fname; Lastname = lname; } } public class Student : Person { public Student(Person person, string code) : base(person.Firstname, person.Lastname) { this.code = code; } public Student(Person person) : base(person.Firstname, person.Lastname) {} public string code { get; set; } } static class Program { static void Main(string[] args) { Person person = new Person("Muhammad Zeeshan", "Khan"); Student s1 = new Student(person, "3229"); Student s2 = new Student(person, "3227"); Console.WriteLine(s1.code); Console.WriteLine(s2.code); } }
출력:
3229 3227
작가: Muhammad Zeeshan
I have been working as a Flutter app developer for a year now. Firebase and SQLite have been crucial in the development of my android apps. I have experience with C#, Windows Form Based C#, C, Java, PHP on WampServer, and HTML/CSS on MYSQL, and I have authored articles on their theory and issue solving. I'm a senior in an undergraduate program for a bachelor's degree in Information Technology.
LinkedIn