C#에서 잘못된 인수 또는 매개 변수에 대해 throw되는 예외 유형
-
C#
의 유효하지 않거나 예기치 않은 매개변수에 대해ArgumentException
발생 -
C#
에서 유효하지 않거나 예기치 않은 매개변수에 대해ArgumentNullException
발생 -
C#
의 유효하지 않거나 예기치 않은 매개변수에 대해ArgumentOutOfRangeException
발생 -
C#
에서 유효하지 않거나 예기치 않은 매개변수에 대한 사용자 정의 예외 생성
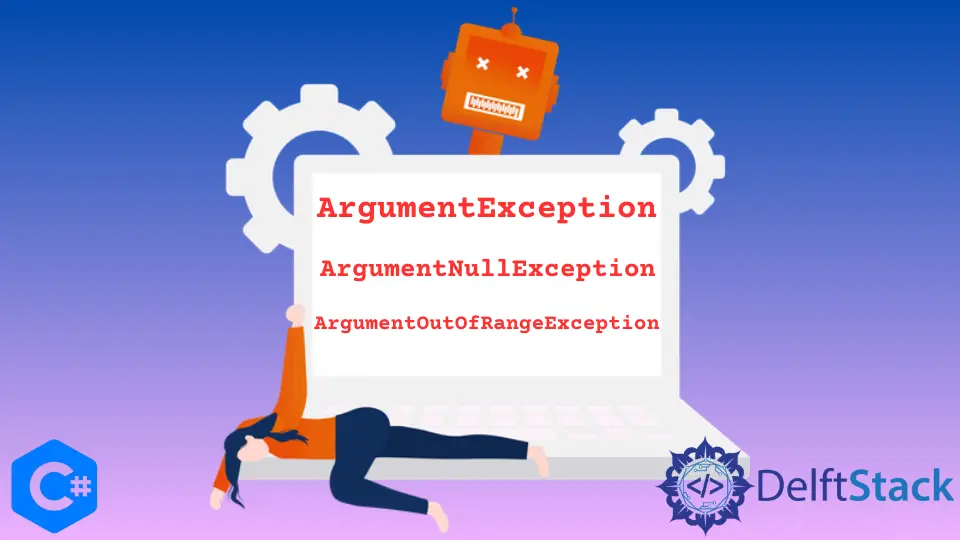
예외는 C# 프로그램의 런타임 오류 또는 발생할 것으로 예상되지 않거나 시스템/응용 프로그램 제약 조건을 위반하는 조건에 대한 정보를 제공합니다. 이 자습서에서는 잘못된 인수 또는 매개 변수와 관련된 세 가지 예외 및 C# 프로그램에서 이를 throw하는 방법을 배웁니다.
유효하지 않은 인수 또는 매개변수를 포착할 때 예외를 발생시키는 코드 블록을 정의하는 것이 예기치 않은 오류를 처리하는 가장 쉬운 방법입니다. 잘못된 코드나 사용자 입력은 오류를 유발할 수 있으며, 이를 감지하려면 C# 애플리케이션 코드 또는 런타임에서 예외가 발생해야 합니다.
C#
의 유효하지 않거나 예기치 않은 매개변수에 대해 ArgumentException
발생
메소드가 유효하지 않은 인수를 수신하면 ArgumentException
이 발생합니다. ArgumentException.GetType().Name
속성을 사용하여 예외 개체의 이름을 표시합니다.
Message
속성을 사용하면 발생 이유가 포함된 예외 메시지의 텍스트를 표시할 수 있습니다. C#에서 예외를 던질 때마다 해당 예외의 Message
속성에는 잘못된 인수와 예상되는 인수 값 범위를 설명하는 의미 있는 메시지가 포함되어야 합니다.
ArgumentNullException
및 ArgumentOutOfRangeException
은 ArgumentException
클래스의 파생 클래스입니다. ArgumentException
클래스 자체는 System.SystemException
클래스에서 상속됩니다.
일반적으로 System.Exception
은 C#에서 잘못된 인수 또는 매개변수에 대해 발생하는 모든 예외의 기본 클래스입니다.
using System;
namespace ArgumentExceptionExp {
class UserEnrollment {
static void Main(string[] args) {
Console.WriteLine("Please enter your first name and last name for enrollment.");
Console.WriteLine("First Name: ");
var userFirstN = Console.ReadLine();
Console.WriteLine("Last Name: ");
var userLastN = Console.ReadLine();
try {
var userFullN = ShowFullUserName(userFirstN, userLastN);
Console.WriteLine($"Congratulations, {userFullN}!");
} catch (ArgumentException ex) {
Console.WriteLine("Something went wrong, please try again!");
Console.WriteLine(ex);
}
}
static string ShowFullUserName(string userFirstN, string userLastN) {
if (userFirstN == "" || userLastN == "")
throw new ArgumentException(
"Attention, please! Your first or last user name cannot be empty.");
return $"{userFirstN} {userLastN}";
}
}
}
출력:
Please enter your first name and last name for enrollment.
First Name:
Last Name:
Kazmi
Something went wrong, please try again!
System.ArgumentException: Attention, please! Your first or last user name cannot be empty.
at ArgumentExceptionExp.UserEnrollment.ShowFullUserName (System.String userFirstN, System.String userLastN) [0x00020] in <8fb4997067094d929e0f81a3893882f9>:0
at ArgumentExceptionExp.UserEnrollment.Main (System.String[] args) [0x0002f] in <8fb4997067094d929e0f81a3893882f9>:0
이 경우 ArgumentException
은 예기치 않거나 잘못된 활동으로 인해 메서드가 정상적인 기능을 완료하지 못하는 경우에만 throw됩니다. 디버깅할 때 추가 정보를 제공하기 위해 다시 throw되는 예외에 정보를 추가하는 것은 좋은 프로그래밍 방법입니다.
throw
키워드와 System.Exception
클래스에서 파생된 새 인스턴스를 사용하여 예외를 throw할 수 있습니다. .NET
프레임워크는 잘못된 인수 및 매개변수를 처리하는 데 사용할 수 있는 많은 표준 사전 정의 예외 유형을 제공합니다.
C#
에서 유효하지 않거나 예기치 않은 매개변수에 대해 ArgumentNullException
발생
C# 프로그램의 메서드가 유효한 인수로 받아들이지 않는 null 참조를 수신하면 오류를 이해하기 위해 ArgumentNullException
을 throw해야 합니다. 오류를 방지하기 위해 인스턴스화되지 않은 개체가 메서드에 전달될 때 런타임에 throw됩니다.
또한 메서드 호출에서 반환된 개체가 두 번째 메서드에 인수로 전달되었지만 원래 반환된 개체의 값이 null
인 경우 throw됩니다.
동작은 ArgumentException
과 동일합니다. 그러나 값이 0x80004003
인 HRESULT E_POINTER
를 사용합니다.
using System;
namespace TryCatchArgNullExcep {
class ArgNullExpProgram {
static void Main(string[] args) {
try {
var ArgNullEVariable = new ArgNullMember(null, 32);
} catch (ArgumentNullException ex) {
Console.WriteLine("Sorry for the inconvenience, something went wrong...");
Console.WriteLine(ex);
}
}
class ArgNullMember {
public ArgNullMember(string memberName, int memberAge) {
if (memberName == null)
throw new ArgumentNullException(
nameof(memberName), "The member name cannot be `null` or empty. Please, try again!");
MemberName = memberName;
MemberAge = memberAge;
}
public string MemberName { get; private set; }
public int MemberAge { get; private set; }
}
}
}
출력:
Sorry for the inconvenience, something went wrong...
System.ArgumentNullException: The member name cannot be `null` or empty. Please, try again!
Parameter name: memberName
at TryCatchArgNullExcep.ArgNullExpProgram+ArgNullMember..ctor (System.String memberName, System.Int32 memberAge) [0x00010] in <1001b7741efd42ec97508d09c5fc60a2>:0
at TryCatchArgNullExcep.ArgNullExpProgram.Main (System.String[] args) [0x00002] in <1001b7741efd42ec97508d09c5fc60a2>:0
C#
의 유효하지 않거나 예기치 않은 매개변수에 대해 ArgumentOutOfRangeException
발생
이 예외는 인수 값이 C#에서 호출된 메서드에 정의된 허용 가능한 값 범위를 벗어날 때 throw됩니다. 일반적으로 ArgumentOutOfRangeException
은 개발자 오류로 인해 발생합니다.
try/catch
블록에서 처리하는 대신 예외의 원인을 제거하거나 인수를 메서드에 전달하기 전에 유효성을 검사해야 합니다.
클래스는 System.Collections
및 System.IO
네임스페이스, 어레이 클래스 및 String
클래스의 문자열 조작 메서드에서 이를 광범위하게 사용합니다. C#의 CLR 또는 다른 클래스 라이브러리에서 발생하며 개발자 오류를 나타냅니다.
using System;
namespace ArgumentOutOfRangeExceptionExp {
class userInfo {
static void Main(string[] args) {
try {
Console.WriteLine("Enter User Name: ");
string Hassan = Console.ReadLine();
Console.WriteLine("Enter your Age: ");
int ageU = Int32.Parse(Console.ReadLine());
var user = new userInfoProcess(Hassan, ageU);
} catch (ArgumentOutOfRangeException ex) {
Console.WriteLine("Please be patient, something went wrong.");
Console.WriteLine(ex);
}
}
}
class userInfoProcess {
public userInfoProcess(string userName, int userAge) {
if (userName == null) {
throw new ArgumentOutOfRangeException(nameof(userName),
"Your username is invalid, please try again!");
} else if (userName == "") {
throw new ArgumentOutOfRangeException(nameof(userName),
"Your username is invalid, please try again!");
}
if (userAge < 18 || userAge > 50)
throw new ArgumentOutOfRangeException(
nameof(userAge), "Your age is outside the allowable range, please enter a valid user.");
ArgNullUName = userName;
ArgNullUAge = userAge;
}
public string ArgNullUName { get; private set; }
public int ArgNullUAge { get; private set; }
}
}
출력:
Enter User Name:
Hassan
Enter your Age:
11
Please be patient, something went wrong.
System.ArgumentOutOfRangeException: Your age is outside the allowable range, please enter a valid user.
Parameter name: userAge
at ArgumentOutOfRangeExceptionExp.userInfoProcess..ctor (System.String userName, System.Int32 userAge) [0x00052] in <b199b92ace93448f8cc4c37cc4d5df33>:0
at ArgumentOutOfRangeExceptionExp.userInfo.Main (System.String[] args) [0x00029] in <b199b92ace93448f8cc4c37cc4d5df33>:0
C#
에서 유효하지 않거나 예기치 않은 매개변수에 대한 사용자 정의 예외 생성
C#에서 잘못된 인수 또는 매개 변수에 대한 예외를 정의할 수도 있습니다. 사용자 정의 예외 클래스는 Exception
클래스에서 파생됩니다.
try/catch
및 finally
블록에서 지원하는 C#의 예외 처리는 코드 런타임 오류를 감지하고 처리하는 메커니즘입니다.
using System;
namespace UserDefinedExceptionExp {
class ExceptionHandlingC {
static void Main(string[] args) {
string Name = "Hassan";
int Age = 18;
Check temp = new Check();
try {
temp.checkTemp(Name, Age);
} catch (InvalidNameException e) {
Console.WriteLine("InvalidNameException: {0}", e.Message);
}
Console.ReadKey();
}
}
}
public class InvalidNameException : Exception {
public InvalidNameException(string message) : base(message) {}
}
public class Check {
public void checkTemp(string name, int age) {
// this exception will be thrown if the user name will be `Hassan`
if (name == "Hassan") {
throw(new InvalidNameException("User is not valid."));
} else {
Console.WriteLine("You are successfully signed in with the name: {0}", name);
}
}
}
출력:
InvalidNameException: User is not valid.
이 자습서에서는 잘못된 인수 및 매개 변수에 대해 일반적으로 발생하는 세 가지 예외 유형과 C#에서 사용자 정의 예외를 만드는 방법을 배웠습니다.
비결은 무엇이 잘못되었는지, 왜 잘못되었는지, C# 프로그램에서 이를 수정하는 방법에 대한 세부 정보를 제공하는 올바른 예외를 사용하고 throw하는 것입니다.
Hassan is a Software Engineer with a well-developed set of programming skills. He uses his knowledge and writing capabilities to produce interesting-to-read technical articles.
GitHub