C++에서 문자로 파일 문자 읽기
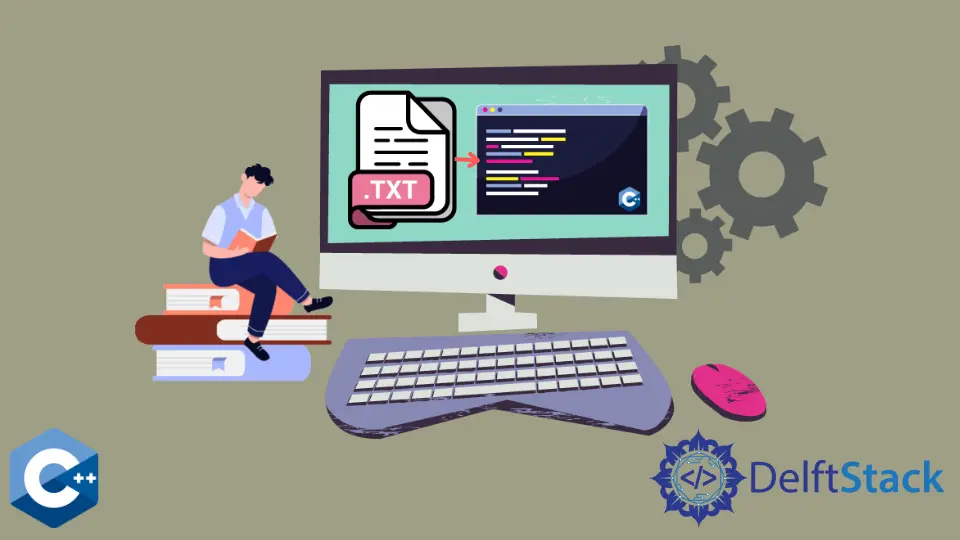
이 기사에서는 C++에서char
로 텍스트 파일char
를 읽는 방법에 대해 설명합니다.
ifstream
및get
메서드를 사용하여 Char로 파일 문자 읽기
파일 I/O를 C++ 방식으로 처리하는 가장 일반적인 방법은std::ifstream
을 사용하는 것입니다. 처음에ifstream
객체는 열어야하는 파일 이름의 인수로 초기화됩니다. if
문은 파일 열기가 성공했는지 확인합니다. 다음으로 내장 된get
함수를 사용하여 char로 파일 내의 텍스트를 검색하고vector
컨테이너에서push_back
합니다. 마지막으로 데모 목적으로 벡터 요소를 콘솔에 출력합니다.
#include <fstream>
#include <iostream>
#include <vector>
using std::cerr;
using std::cout;
using std::endl;
using std::ifstream;
using std::string;
using std::vector;
int main() {
string filename("input.txt");
vector<char> bytes;
char byte = 0;
ifstream input_file(filename);
if (!input_file.is_open()) {
cerr << "Could not open the file - '" << filename << "'" << endl;
return EXIT_FAILURE;
}
while (input_file.get(byte)) {
bytes.push_back(byte);
}
for (const auto &i : bytes) {
cout << i << "-";
}
cout << endl;
input_file.close();
return EXIT_SUCCESS;
}
getc
함수를 사용하여 Char로 파일 문자 읽기
char로 파일 char을 읽는 또 다른 방법은FILE*
스트림을 인수로 사용하고 가능한 경우 다음 문자를 읽는getc
함수를 사용하는 것입니다. 다음으로, 입력 파일 스트림은 마지막 char
에 도달 할 때까지 반복되어야하며, 파일의 끝에 도달했는지 확인하는 feof
함수로 구현됩니다. 열린 파일 스트림이 더 이상 필요하지 않으면 항상 닫는 것이 좋습니다.
#include <fstream>
#include <iostream>
#include <vector>
using std::cerr;
using std::cout;
using std::endl;
using std::ifstream;
using std::string;
using std::vector;
int main() {
string filename("input.txt");
vector<char> bytes;
FILE* input_file = fopen(filename.c_str(), "r");
if (input_file == nullptr) {
return EXIT_FAILURE;
}
unsigned char character = 0;
while (!feof(input_file)) {
character = getc(input_file);
cout << character << "-";
}
cout << endl;
fclose(input_file);
return EXIT_SUCCESS;
}
fgetc
함수를 사용하여 문자로 파일 문자 읽기
fgetc
는getc
와 똑같은 기능을 구현하는 이전 함수의 대체 방법입니다. 이 경우 파일 스트림의 끝에 도달하면 EOF
를 반환하므로 if
문에 fgetc
반환 값이 사용됩니다. 권장하는대로 프로그램을 종료하기 전에fclose
호출로 스트림을 닫습니다.
#include <fstream>
#include <iostream>
#include <vector>
using std::cerr;
using std::cout;
using std::endl;
using std::ifstream;
using std::string;
using std::vector;
int main() {
string filename("input.txt");
vector<char> bytes;
FILE* input_file = fopen(filename.c_str(), "r");
if (input_file == nullptr) {
return EXIT_FAILURE;
}
int c;
while ((c = fgetc(input_file)) != EOF) {
putchar(c);
cout << "-";
}
cout << endl;
fclose(input_file);
return EXIT_SUCCESS;
}
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook