C++에서 숫자의 자릿수 계산
-
std::to_string
및std::string::size
함수를 사용하여 C++에서 숫자의 자릿수 계산 -
std::string::erase
및std::remove_if
메서드를 사용하여 C++에서 숫자의 자릿수를 계산합니다
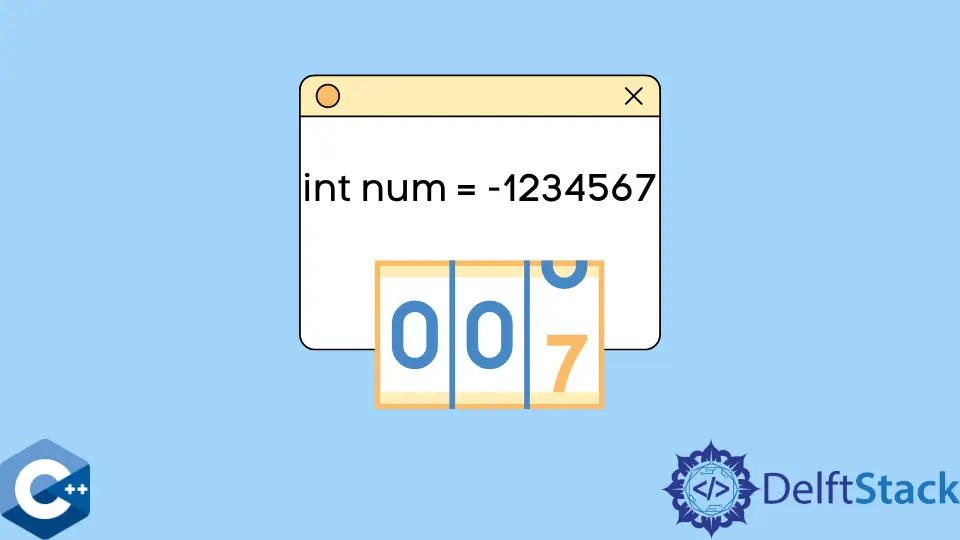
이 기사에서는 숫자 C++에서 자릿수를 계산하는 방법에 대한 여러 가지 방법을 보여줍니다.
std::to_string
및std::string::size
함수를 사용하여 C++에서 숫자의 자릿수 계산
숫자의 자릿수를 계산하는 가장 간단한 방법은 숫자를std::string
객체로 변환 한 다음std::string
의 내장 함수를 호출하여 개수를 검색하는 것입니다. 이 경우, 정수 유형으로 가정하고 크기를 정수로 반환하는 단일 인수를 사용하는 별도의 템플릿 함수countDigits
를 구현했습니다. 다음 예제는 부호 기호도 계산하므로 음의 정수에 대해 잘못된 숫자를 출력합니다.
#include <iostream>
#include <string>
#include <vector>
using std::cerr;
using std::cout;
using std::endl;
using std::string;
using std::to_string;
template <typename T>
size_t countDigits(T n) {
string tmp;
tmp = to_string(n);
return tmp.size();
}
int main() {
int num1 = 1234567;
int num2 = -1234567;
cout << "number of digits in " << num1 << " = " << countDigits(num1) << endl;
cout << "number of digits in " << num2 << " = " << countDigits(num2) << endl;
exit(EXIT_SUCCESS);
}
출력:
number of digits in 1234567 = 7
number of digits in -1234567 = 8
countDigits
함수의 이전 구현의 결함을 해결하기 위해 단일if
문을 추가하여 주어진 숫자가 음수인지 평가하고 1보다 작은 문자열 크기를 반환합니다. 숫자가0
보다 크면 다음 예제 코드에 구현 된대로 문자열 크기의 원래 값이 반환됩니다.
#include <iostream>
#include <string>
#include <vector>
using std::cerr;
using std::cout;
using std::endl;
using std::string;
using std::to_string;
template <typename T>
size_t countDigits(T n) {
string tmp;
tmp = to_string(n);
if (n < 0) return tmp.size() - 1;
return tmp.size();
}
int main() {
int num1 = 1234567;
int num2 = -1234567;
cout << "number of digits in " << num1 << " = " << countDigits(num1) << endl;
cout << "number of digits in " << num2 << " = " << countDigits(num2) << endl;
exit(EXIT_SUCCESS);
}
출력:
number of digits in 1234567 = 7
number of digits in -1234567 = 7
std::string::erase
및std::remove_if
메서드를 사용하여 C++에서 숫자의 자릿수를 계산합니다
앞의 예는 위의 문제에 대한 완전한 해결책을 제공하지만std::string::erase
및std::remove_if
함수 조합을 사용하여countDigits
를 과도하게 엔지니어링하여 숫자가 아닌 기호를 제거 할 수 있습니다. 또한이 메서드는 부동 소수점 값으로 작동 할 수있는 함수를 구현하는 디딤돌이 될 수 있습니다. 하지만 다음 샘플 코드는 부동 소수점 값과 호환되지 않습니다.
#include <iostream>
#include <string>
#include <vector>
using std::cerr;
using std::cout;
using std::endl;
using std::string;
using std::to_string;
template <typename T>
size_t countDigits(T n) {
string tmp;
tmp = to_string(n);
tmp.erase(std::remove_if(tmp.begin(), tmp.end(), ispunct), tmp.end());
return tmp.size();
}
int main() {
int num1 = 1234567;
int num2 = -1234567;
cout << "number of digits in " << num1 << " = " << countDigits(num1) << endl;
cout << "number of digits in " << num2 << " = " << countDigits(num2) << endl;
exit(EXIT_SUCCESS);
}
출력:
number of digits in 1234567 = 7
number of digits in -1234567 = 7
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook