C++에서 한 줄씩 파일을 읽는 방법
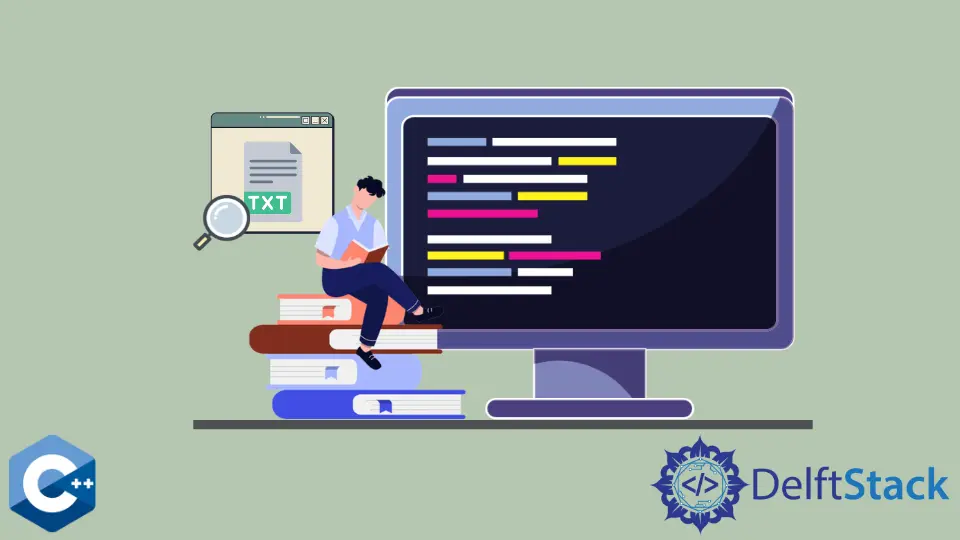
이 기사에서는 C++에서 한 줄씩 파일을 읽는 방법을 소개합니다.
std::getline()
함수를 사용하여 한 줄씩 파일 읽기
getline()
함수는 C++에서 한 줄씩 파일을 읽는 데 선호되는 방법입니다. 이 함수는 구분 기호 문자가 나타날 때까지 입력 스트림에서 문자를 읽은 다음 문자열에 저장합니다. 구분 기호는 세 번째 선택적 매개 변수로 전달되며 기본적으로 새 줄 문자 \n
으로 간주됩니다.
getline
메소드는 스트림에서 데이터를 읽을 수있는 경우 0이 아닌 값을 반환하므로 파일 끝에 도달 할 때까지 파일에서 행을 계속 검색하는while
루프 조건으로 지정할 수 있습니다. 입력 스트림에 is_open
메소드와 관련된 파일이 있는지 확인하는 것이 좋습니다.
#include <fstream>
#include <iostream>
#include <vector>
using std::cerr;
using std::cout;
using std::endl;
using std::ifstream;
using std::string;
using std::vector;
int main() {
string filename("input.txt");
vector<string> lines;
string line;
ifstream input_file(filename);
if (!input_file.is_open()) {
cerr << "Could not open the file - '" << filename << "'" << endl;
return EXIT_FAILURE;
}
while (getline(input_file, line)) {
lines.push_back(line);
}
for (const auto &i : lines) cout << i << endl;
input_file.close();
return EXIT_SUCCESS;
}
C 라이브러리getline()
함수를 사용하여 한 줄씩 파일 읽기
getline
함수는 파일을 한 줄씩 반복하고 추출 된 줄을 문자열 변수에 저장하는 데 유사하게 사용할 수 있습니다. 가장 큰 차이점은 나중에 세 번째 매개 변수로 전달 될FILE *
객체를 반환하는fopen
함수를 사용하여 파일 스트림을 열어야한다는 것입니다.
getline
함수는nullptr
로 설정된char
포인터에 저장됩니다. 이는 함수 자체가 검색된 문자에 대해 동적으로 메모리를 할당하기 때문에 유효합니다. 따라서getline
호출이 실패하더라도 프로그래머가char
포인터를 명시 적으로 해제해야합니다.
또한 함수를 호출하기 전에 size_t
유형의 두 번째 매개 변수를 0으로 설정하는 것이 중요합니다. getline
기능의 자세한 매뉴얼을보실 수 있습니다.
#include <iostream>
#include <vector>
using std::cerr;
using std::cout;
using std::endl;
using std::string;
using std::vector;
int main() {
string filename("input.txt");
vector<string> lines;
char* c_line = nullptr;
size_t len = 0;
FILE* input_file = fopen(filename.c_str(), "r");
if (input_file == nullptr) return EXIT_FAILURE;
while ((getline(&c_line, &len, input_file)) != -1) {
lines.push_back(line.assign(c_line));
}
for (const auto& i : lines) {
cout << i;
}
cout << endl;
fclose(input_file);
free(c_line);
return EXIT_SUCCESS;
}
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook