C++에서 벡터의 내용을 인쇄하는 방법
-
요소 액세스 표기법과 함께
for
루프를 사용하여 벡터 내용 인쇄 - 요소 액세스 표기법과 함께 범위 기반 루프를 사용하여 벡터 내용 인쇄
-
std::copy
를 사용하여 벡터 내용 인쇄 -
std::for_each
를 사용하여 벡터 내용 인쇄
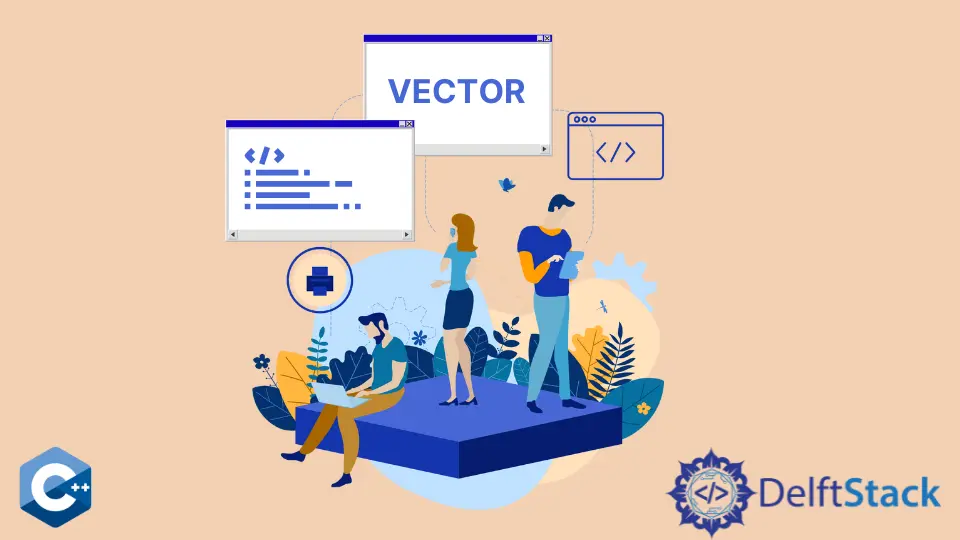
이 기사에서는 C++에서 벡터 내용을 인쇄하는 방법에 대한 몇 가지 방법을 소개합니다.
요소 액세스 표기법과 함께for
루프를 사용하여 벡터 내용 인쇄
vector
요소는 at()
메소드 또는[]
연산자로 액세스 할 수 있습니다. 이 솔루션에서 우리는 둘 다 시연하고cout
스트림으로 내용을 인쇄합니다. 처음에는 벡터 변수를 임의의 정수로 초기화 한 다음 요소를 하나씩 반복하여 스트림을 출력합니다.
#include <iostream>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::string;
using std::vector;
int main() {
vector<int> int_vec = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
for (size_t i = 0; i < int_vec.size(); ++i) {
cout << int_vec.at(i) << "; ";
}
cout << endl;
for (size_t i = 0; i < int_vec.size(); ++i) {
cout << int_vec[i] << "; ";
}
cout << endl;
return EXIT_SUCCESS;
}
출력:
1; 2; 3; 4; 5; 6; 7; 8; 9; 10;
1; 2; 3; 4; 5; 6; 7; 8; 9; 10;
요소 액세스 표기법과 함께 범위 기반 루프를 사용하여 벡터 내용 인쇄
이전 솔루션은 비교적 간단하지만 매우 장황 해 보입니다. 현대 C++는 반복을 표현하는보다 유연한 방법을 제공하며, 그중 하나는 범위 기반 루프입니다. 루프가 비교적 쉽게 작업을 수행하고 병렬화 할 필요가 없을 때 더 읽기 쉬운 것으로 간주됩니다.
#include <iostream>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::string;
using std::vector;
int main() {
vector<int> int_vec = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
for (const auto &item : int_vec) {
cout << item << "; ";
}
cout << endl;
return EXIT_SUCCESS;
}
std::copy
를 사용하여 벡터 내용 인쇄
벡터 반복 및 출력 연산을 하나의 문에서 수행하는 다소 고급 방법은<algorithm>
라이브러리에 정의 된copy
함수를 호출하는 것입니다. 이 메소드는 반복기로 지정된 벡터 범위를 취하고 세 번째 인수로 ostream_iterator
를 전달하여 범위의 내용을cout
스트림으로 리디렉션합니다.
#include <algorithm>
#include <iostream>
#include <iterator>
#include <vector>
using std::cin;
using std::copy;
using std::cout;
using std::endl;
using std::ostream_iterator;
using std::vector;
int main() {
vector<int> int_vec = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
copy(int_vec.begin(), int_vec.end(), ostream_iterator<int>(cout, "; "));
cout << endl;
return EXIT_SUCCESS;
}
std::for_each
를 사용하여 벡터 내용 인쇄
단일 문에서 반복 및 출력 작업을 수행하는 또 다른 STL 알고리즘은 for_each
입니다. 이 방법은 지정된 범위의 각 요소에 특정 함수 개체를 적용 할 수있는 강력한 도구입니다. 이 경우 출력 스트림에 요소를 인쇄하는lambda
표현식을 전달합니다.
#include <algorithm>
#include <iostream>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::for_each;
using std::vector;
int main() {
vector<int> int_vec = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
for_each(int_vec.begin(), int_vec.end(),
[](const int& n) { cout << n << "; "; });
cout << endl;
return EXIT_SUCCESS;
}
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook