C++에서 배열 크기를 찾는 방법
-
sizeof
연산자를 사용하여 C++에서 C 스타일 배열 크기 계산 -
std::array
컨테이너를 사용하여 배열 데이터 저장 및 크기 계산 -
std::vector
컨테이너를 사용하여 배열 데이터 저장 및 크기 계산 -
std::size
메서드를 사용하여 배열 크기 계산
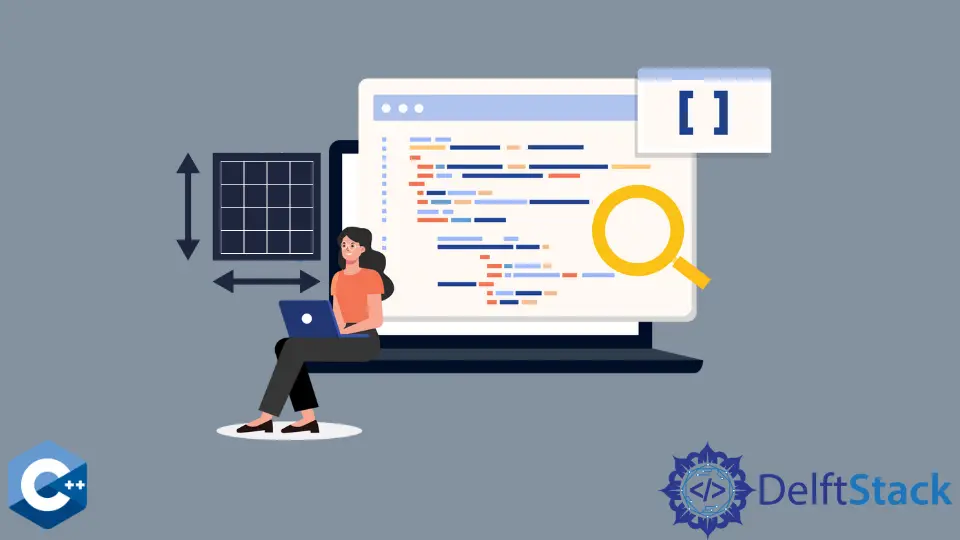
이 기사에서는 다양한 C++ 방법을 사용하여배열
크기를 얻는 방법을 소개합니다.
sizeof
연산자를 사용하여 C++에서 C 스타일 배열 크기 계산
먼저 C 스타일 배열의 크기를 찾으려고 할 때 어떤 일이 발생하는지 살펴 보겠습니다. 이 예에서는c_array
변수를 정의하고 10 개의 정수 값으로 초기화합니다. 이 배열의 크기를 계산하기 위해 객체 크기를 바이트 단위로 반환하는sizeof
단항 연산자를 사용합니다. 기본적으로,c_array
객체를 저장하는 데 걸리는 바이트 수를 단일 요소c_array[0]
의 크기로 나눕니다.
#include <iostream>
using std::cin;
using std::cout;
using std::endl;
int main() {
int c_array[] = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
cout << "array size: " << sizeof c_array / sizeof c_array[0] << endl;
return 0;
}
출력:
array size: 10
보시다시피 출력이 올바른 것 같습니다. 이제 동일한 작업을 수행하는 별도의 함수를 정의 해 보겠습니다.
#include <iostream>
using std::cin;
using std::cout;
using std::endl;
int get_array_size(int arr[]) { return sizeof arr / sizeof arr[0]; }
int main() {
int c_array[] = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
cout << "array size: " << get_array_size(c_array) << endl;
return 0;
}
출력:
array size: 2
우리는 잘못된 번호를 얻었습니다. sizeof
연산자를 사용하는 것은이 문제를 해결하는 데 신뢰할 수있는 방법이 아닙니다. sizeof
연산자가 올바르게 작동하려면 배열이 함수 매개 변수로 수신되어서는 안되며 힙에서 동적으로 할당되지 않아야합니다. sizeof
의 잘못된 사용을 식별하기 위해 IDE는 일반적으로 오류가 발생하기 쉬운 코드 섹션을 lint하지만 컴파일 중에 표시 할 경고에 대한 컴파일러 플래그를 지정할 수도 있습니다 (예 :-Wsizeof-pointer-div
또는-Wall
gcc
와 함께).
많은 엣지 케이스에 대해 걱정하지 않고 C 스타일 배열의 크기를 계산하는 보편적 인 방법이 없기 때문에std::vector
클래스가 존재합니다.
std::array
컨테이너를 사용하여 배열 데이터 저장 및 크기 계산
std::vector
를 사용하기 전에 고정 크기 배열을 저장하는 데 사용할 수있는std::array
를 언급해야합니다. 자원 효율적이라는 이점이 있으며size()
라는 크기를 검색하는 내장 메서드가 있습니다.
#include <array>
#include <iostream>
using std::array;
using std::cin;
using std::cout;
using std::endl;
int main() {
array<int, 10> arr = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
cout << "array size: " << arr.size() << endl;
return 0;
}
std::vector
컨테이너를 사용하여 배열 데이터 저장 및 크기 계산
std::vector
컨테이너는 동적 배열을 저장하는 데 사용할 수 있으며size()
를 포함하여 다양한 작업을위한 여러 메서드를 제공합니다.
#include <iostream>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::vector;
int main() {
vector<int> int_array = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
cout << "vector size: " << int_array.size() << endl;
return 0;
}
std::size
메서드를 사용하여 배열 크기 계산
std::size
메서드는<iterator>
라이브러리의 일부이며std::array
데이터뿐만 아니라 요소 수를 반환하는std::vector
에서도 작동 할 수 있습니다.
#include <array>
#include <iostream>
#include <vector>
using std::array;
using std::cin;
using std::cout;
using std::endl;
using std::size;
using std::vector;
int main() {
vector<int> int_array = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
array<int, 10> arr = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
cout << "vector size: " << size(int_array) << endl;
cout << "array size: " << size(arr) << endl;
return 0;
}
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook